Unlock Terracotta Health with Groovy: A Quick Guide
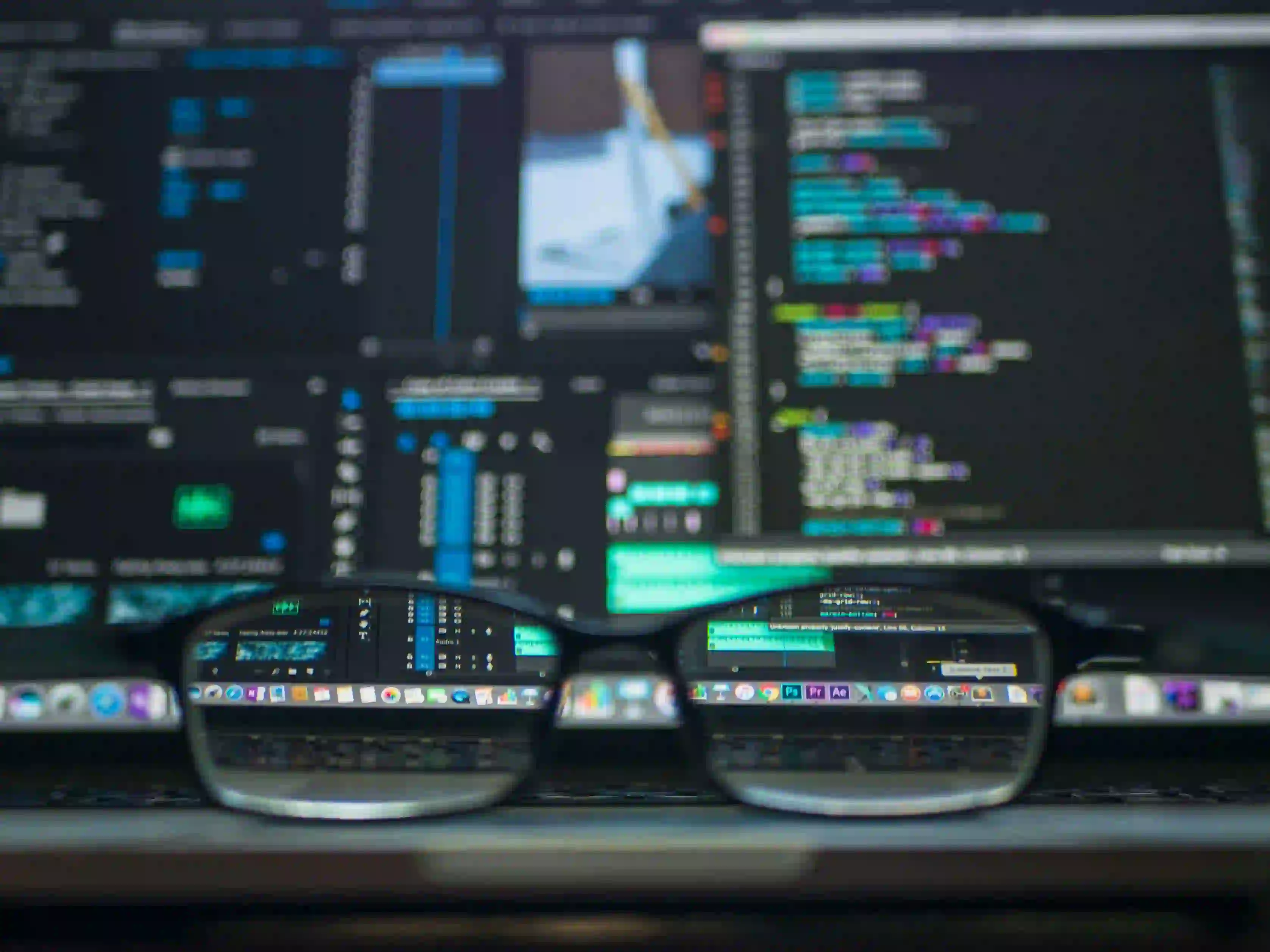
Unlock Terracotta Health with Groovy: A Quick Guide
If you are working with Java and looking to leverage the power of Terracotta for caching, then you are in the right place! In this guide, we will explore how to use Groovy, a powerful, concise, and highly productive language that seamlessly integrates with Java, to unlock the potential of Terracotta for managing distributed caches with ease.
What is Terracotta?
Terracotta is an open-source platform known for its in-memory data management and caching solutions. It provides seamless integration with popular caching libraries such as Ehcache, enabling distributed caching and in-memory data management across multiple nodes. With Terracotta, you can seamlessly scale your caching infrastructure and maintain high availability for your applications.
Why Groovy?
Groovy, with its seamless integration with Java, provides a concise, expressive, and highly productive way to work with Java APIs and libraries. Its scripting capabilities, along with a rich set of features, make it an ideal choice for interacting with and leveraging the capabilities of Terracotta for distributed caching.
Setting up the Environment
Before we dive into using Groovy with Terracotta, let’s ensure that you have Java and Groovy installed in your environment. To check if you have Java installed, run the following command in your terminal:
java -version
To check if you have Groovy installed, run the following command:
groovy -version
If Java or Groovy is not installed, refer to the official documentation to set up your environment:
Adding Terracotta to your Project
To add Terracotta to your Java project, you can include the required dependencies in your pom.xml
if you are using Maven, or build.gradle
if you are using Gradle.
If you are using Maven, include the following dependency:
<dependency>
<groupId>net.sf.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>${ehcache.version}</version>
</dependency>
<dependency>
<groupId>org.terracotta</groupId>
<artifactId>terracotta-toolkit-10.7.1</artifactId>
<version>${terracotta.version}</version>
</dependency>
If you are using Gradle, include the following dependency:
implementation 'net.sf.ehcache:ehcache:$ehcacheVersion'
implementation 'org.terracotta:terracotta-toolkit-10.7.1:$terracottaVersion'
Make sure to replace ${ehcache.version}
and ${terracotta.version}
with the appropriate versions you intend to use. After adding the dependencies, remember to sync your project to fetch the new dependencies.
Using Groovy with Terracotta
Now that the environment is set up and the project is configured to use Terracotta, let’s dive into how we can use Groovy to unlock the potential of Terracotta for managing distributed caches. We will go through a simple example to illustrate the usage of Groovy with Terracotta.
Creating a Cache Manager
In this example, we will first create an EhCacheManager
for managing our distributed cache using Groovy. Here’s a simple code snippet:
import net.sf.ehcache.Cache
import net.sf.ehcache.CacheManager
import net.sf.ehcache.Element
def cacheManager = new CacheManager()
def cache = new Cache("myDistributedCache", 10000, true, false, 5, 2)
cacheManager.addCache(cache)
In the above code, we import the necessary classes from the net.sf.ehcache
package, create a new CacheManager
instance, and then create a new cache named "myDistributedCache" with a maximum of 10000 elements, eternal set to true, overflowToDisk set to false, timeToIdleSeconds set to 5, and timeToLiveSeconds set to 2.
The code is concise and straightforward, thanks to the expressive nature of Groovy.
Adding and Retrieving Data from the Cache
Next, let’s look at how we can add data to the cache and retrieve it using Groovy:
def key = "exampleKey"
def value = "exampleValue"
def element = new Element(key, value)
cache.put(element)
def cachedElement = cache.get(key)
println "Retrieved value: ${cachedElement.getObjectValue()}"
In the above code, we create a key-value pair, wrap it in an Element
, and add it to the cache using the put
method. We then retrieve the value associated with the key from the cache using the get
method.
Groovy’s concise syntax and dynamic nature make the code simple and easy to read and understand.
Closing the Chapter
In this quick guide, we explored how to use Groovy to unlock the potential of Terracotta for managing distributed caches. We set up the environment, added Terracotta to our project, and leveraged Groovy’s concise and expressive syntax to create a cache manager and interact with the cache. Groovy’s seamless integration with Java and its scripting capabilities make it an ideal choice for working with Terracotta and achieving high-performance distributed caching.
So, are you ready to take your caching to the next level using Groovy and Terracotta? Give it a try and experience the power of concise, expressive, and highly productive code!
Now it’s your turn. Explore the possibilities, experiment with Groovy and Terracotta, and unlock the full potential of distributed caching in your Java projects. Happy coding!