Boost Your Sprint with These Top Planning Tips for POs
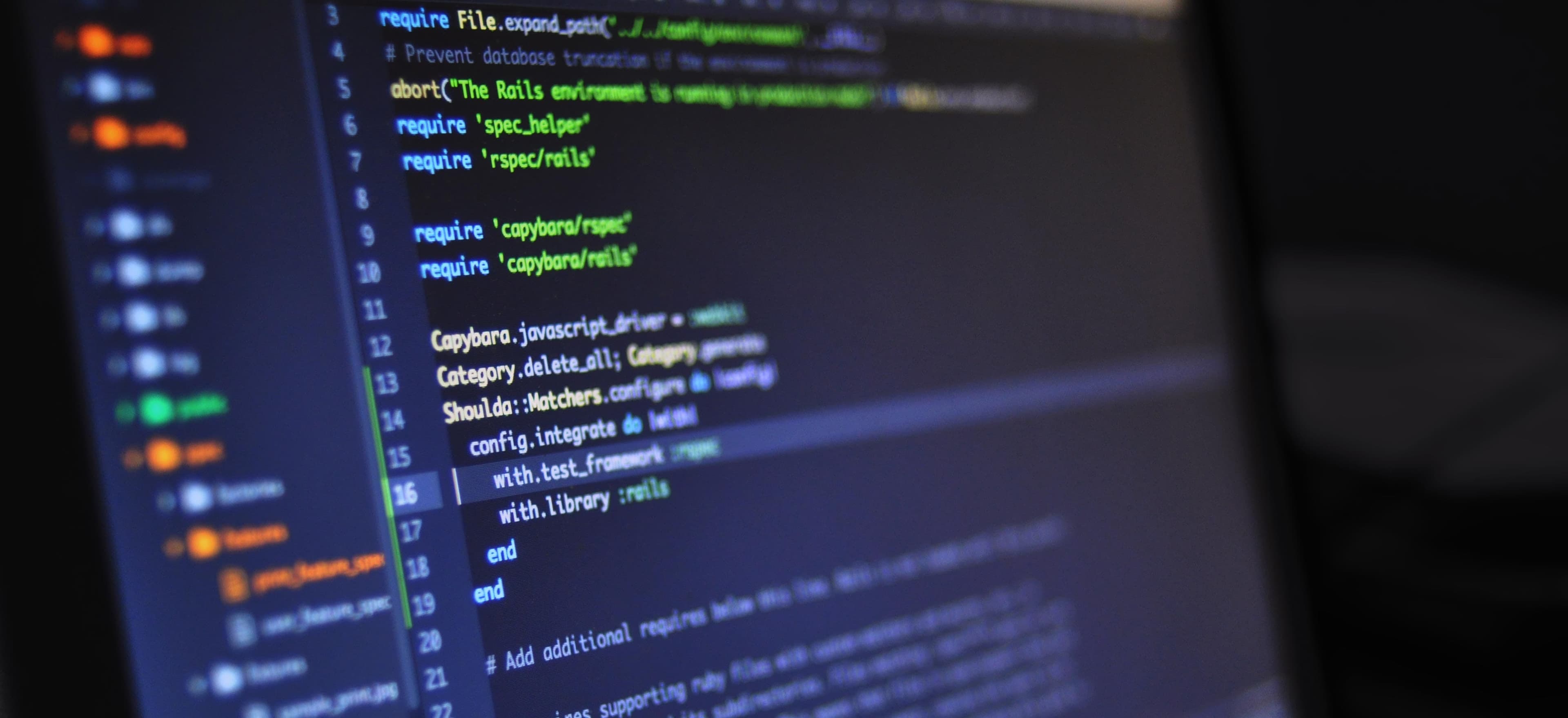
- Published on
Top Java Optimization Techniques for Boosting Performance
As a Java developer, optimizing your code is essential for enhancing the performance and efficiency of your applications. In this article, we will explore some of the top Java optimization techniques that can help you achieve better performance and responsiveness in your applications. By implementing these techniques, you can ensure that your Java applications run smoothly and efficiently, providing a seamless user experience.
1. Use Proper Data Structures
Choosing the right data structures is crucial for optimizing Java code. For example, using HashMap instead of ArrayList for faster key-based lookup can significantly improve performance. When dealing with large datasets, opting for efficient data structures such as HashSet, TreeMap, or ConcurrentHashMap can make a noticeable difference in the overall performance of your application.
// Bad: Using ArrayList for key-based lookup
List<String> names = new ArrayList<>();
// Good: Using HashMap for key-based lookup
Map<String, Integer> ageMap = new HashMap<>();
2. Minimize Object Creation
Excessive object creation can lead to increased memory usage and garbage collection overhead. Reusing objects and employing techniques such as object pooling can help minimize the impact of object instantiation on your application's performance.
// Bad: Creating new object instances excessively
String info = new String("Some information");
// Good: Reusing existing objects
String reusableInfo = "Some information";
3. Efficient Exception Handling
While exceptions are a crucial part of Java programming, inefficient handling can severely impact performance. It is essential to use exceptions for exceptional cases rather than regular program flow. Additionally, using specific exception types instead of broad catch blocks can help in improving performance.
// Bad: Using broad catch blocks
try {
// Code that may throw various exceptions
} catch (Exception e) {
// Handling all exceptions
}
// Good: Using specific exception types
try {
// Code that may throw specific exceptions
} catch (IOException e) {
// Handling IO exceptions
} catch (ParseException e) {
// Handling parse exceptions
}
4. String Manipulation Efficiency
When dealing with string manipulation in Java, using StringBuilder or StringBuffer instead of concatenating strings using the '+' operator can significantly improve performance. This is due to the immutability of strings in Java, which can lead to unnecessary object creation and memory overhead when using the '+' operator for concatenation.
// Bad: Concatenating strings using the '+' operator
String result = "Hello, " + name + "!";
// Good: Using StringBuilder for efficient string manipulation
StringBuilder builder = new StringBuilder();
builder.append("Hello, ").append(name).append("!");
String result = builder.toString();
5. Method and Code Optimization
Optimizing methods and code snippets by minimizing loops, reducing unnecessary calculations, and employing efficient algorithms can drastically enhance the performance of your Java applications. Additionally, utilizing built-in methods and libraries for common operations such as sorting, searching, and data manipulation can lead to improved efficiency.
// Bad: Inefficient loop with unnecessary iterations
for (int i = 0; i < someList.size(); i++) {
// Do something with each element
}
// Good: Utilizing enhanced for loop for improved readability and efficiency
for (String item : someList) {
// Process each element
}
To Wrap Things Up
In conclusion, optimizing Java code is imperative for achieving high performance and responsiveness in your applications. By leveraging proper data structures, minimizing object creation, efficient exception handling, string manipulation, and code optimization, you can significantly enhance the efficiency and speed of your Java applications. Employing these optimization techniques will not only improve the user experience but also lead to more resource-efficient and maintainable codebases.
By incorporating these Java optimization techniques into your development workflow, you can ensure that your applications run smoothly and efficiently, delivering an exceptional user experience.
Implementing these best practices will undoubtedly enhance the performance of your Java applications, making them more efficient and responsive. So, why wait? Start optimizing your Java code today and witness the remarkable improvements in your application's performance.
Remember, consistently reviewing and refactoring your code using these techniques will lead to more robust, scalable, and high-performing Java applications.
What are your favorite Java optimization techniques? Share your thoughts in the comments below.
To further enhance your knowledge of Java optimization, check out this detailed guide on Java performance optimization. Additionally, keep exploring and experimenting with various optimization strategies to refine your Java programming skills. Happy coding!