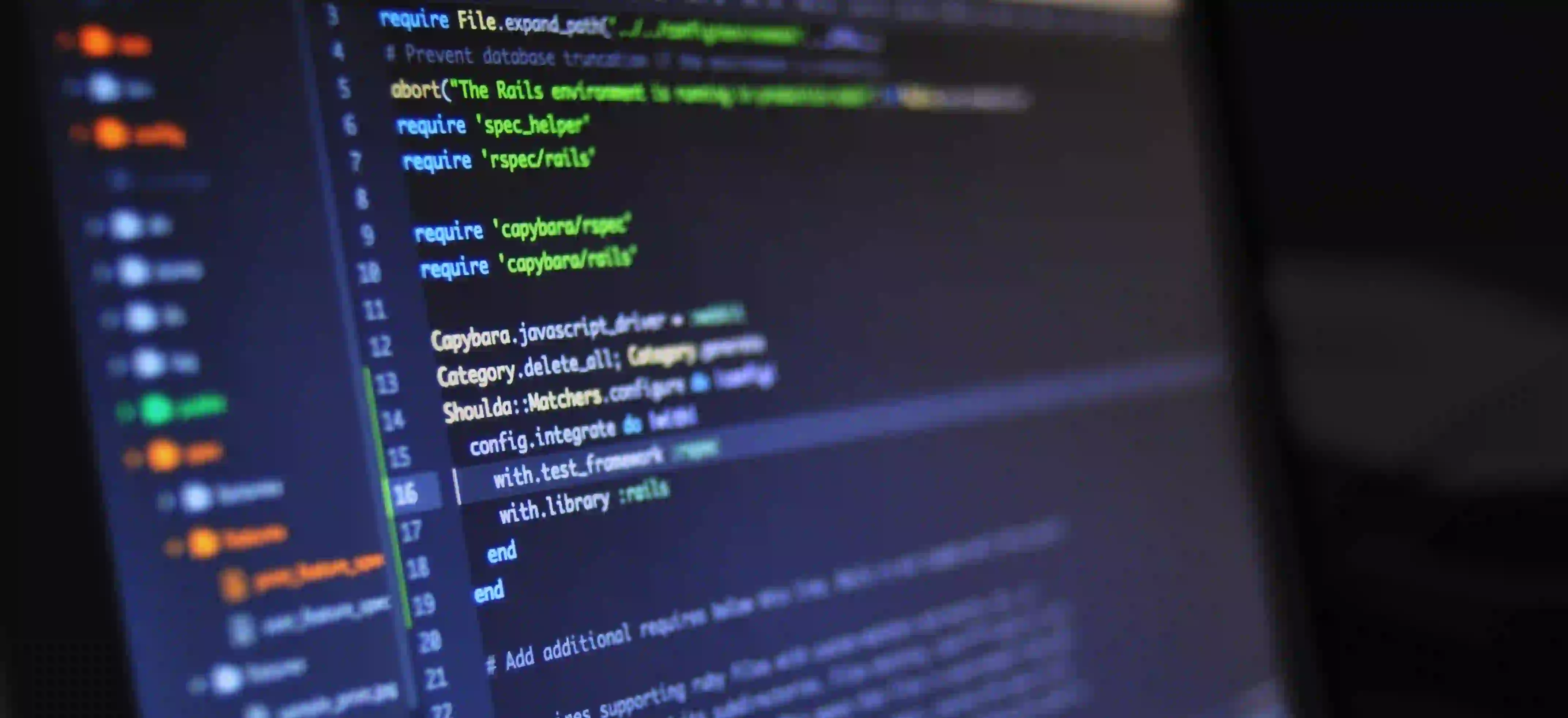
Crafting JSF Apps with Databases Using NetBeans & Java EE
JavaServer Faces (JSF) is a popular Java framework for building component-based user interfaces for web applications. It provides a rich set of tools for simplifying the development of web interfaces.
In this tutorial, we will explore the process of building a JSF web application that interacts with a database. We will leverage the power of NetBeans and Java EE to create a fully functional web application with database connectivity.
Getting Started
Before we dive into the code, it's essential to set up the development environment. We'll need NetBeans, a powerful integrated development environment (IDE) for Java development. Download and install the latest version of NetBeans from here.
We'll also require a MySQL database for this tutorial. If you don't have MySQL installed, you can download it from here.
Creating a Database
We'll start by creating a simple database to store and retrieve data for our web application. Use the following SQL script to create a new database and a table:
CREATE DATABASE jsf_example;
USE jsf_example;
CREATE TABLE employees (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100),
designation VARCHAR(100)
);
This script creates a database called jsf_example
and a table called employees
with columns for id
, name
, and designation
.
Setting Up the Project in NetBeans
Open NetBeans and create a new "Java Web" project. Choose "JavaServer Faces" as the web framework.
Once the project is created, we need to configure the database connection. Right-click on the project and select "New" > "Other" > "Persistence" > "Entity Classes from Database". Follow the wizard to create a new data source and connection to the MySQL database.
Creating the Entity Class
In the project, create a new package called model
. Inside this package, create a new Java class called Employee
. This class will map to the employees
table in the database using Java Persistence API (JPA) annotations.
package model;
import java.io.Serializable;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Employee implements Serializable {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int id;
private String name;
private String designation;
// Getters and setters
}
In this code snippet, we define a simple JPA entity class Employee
with fields mapped to the columns in the employees
table. The @Entity
annotation marks the class as an entity, and the @Id
and @GeneratedValue
annotations define the primary key.
Creating the Managed Bean
Next, we'll create a managed bean to handle user interactions and data retrieval. In the controller
package, create a new Java class called EmployeeController
.
package controller;
import java.io.Serializable;
import java.util.List;
import javax.annotation.PostConstruct;
import javax.ejb.EJB;
import javax.faces.view.ViewScoped;
import javax.inject.Named;
import model.Employee;
import service.EmployeeService;
@Named
@ViewScoped
public class EmployeeController implements Serializable {
@EJB
private EmployeeService employeeService;
private List<Employee> employees;
@PostConstruct
public void init() {
employees = employeeService.getAllEmployees();
}
// Getters and setters
}
In this snippet, we use the @Named
annotation to make the bean accessible to the JSF pages and the @ViewScoped
annotation to define the scope of the bean. The @EJB
annotation injects the EmployeeService, and the @PostConstruct
method initializes the employees
list by calling the getAllEmployees
method from the EmployeeService
.
Creating the Service Class
In the service
package, create a new Java class called EmployeeService
to handle the business logic and database operations.
package service;
import java.util.List;
import javax.ejb.Stateless;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import model.Employee;
@Stateless
public class EmployeeService {
@PersistenceContext
private EntityManager entityManager;
public List<Employee> getAllEmployees() {
return entityManager.createQuery("SELECT e FROM Employee e", Employee.class).getResultList();
}
public void addEmployee(Employee employee) {
entityManager.persist(employee);
}
// Other operations such as update and delete
}
In this code, the @Stateless
annotation marks the class as an EJB session bean. The @PersistenceContext
annotation injects the entity manager for performing database operations. The getAllEmployees
method retrieves all employees from the database, and the addEmployee
method adds a new employee.
Creating the JSF Page
Now, it's time to create the user interface for displaying and adding employees. In the webapp
folder, create a new XHTML file called index.xhtml
.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core"
xmlns:ui="http://java.sun.com/jsf/facelets">
<h:head>
<title>Employee Management</title>
</h:head>
<h:body>
<h2>Employee List</h2>
<h:dataTable value="#{employeeController.employees}" var="employee">
<h:column>
#{employee.id}
</h:column>
<h:column>
#{employee.name}
</h:column>
<h:column>
#{employee.designation}
</h:column>
</h:dataTable>
<h2>Add Employee</h2>
<h:form>
<h:inputText value="#{employee.name}" />
<h:inputText value="#{employee.designation}" />
<h:commandButton value="Add" action="#{employeeController.addEmployee}" />
</h:form>
</h:body>
</html>
This XHTML file defines the layout for displaying the list of employees and adding a new employee. The h:dataTable
tag iterates over the employees list obtained from the managed bean, and the form allows users to input a new employee's name and designation.
Running the Application
We can now run the application and see it in action. Right-click on the project and select "Run". NetBeans will deploy the application to the embedded GlassFish server and open a web browser to display the JSF page.
You should see the list of employees from the database displayed on the web page. You can also try adding new employees and see them reflected in the database.
A Final Look
In this tutorial, we've explored the process of building a JSF web application with database connectivity using NetBeans and Java EE. We've created an entity class to map to the database table, a managed bean to handle user interactions, a service class to perform database operations, and a JSF page for the user interface.
By following these steps, you can build robust web applications with Java EE technologies, leveraging the power of NetBeans for rapid development and easy database integration.
Give it a try and start creating your own JSF applications with database interactions!