Solving Common Issues with the Android Camera API
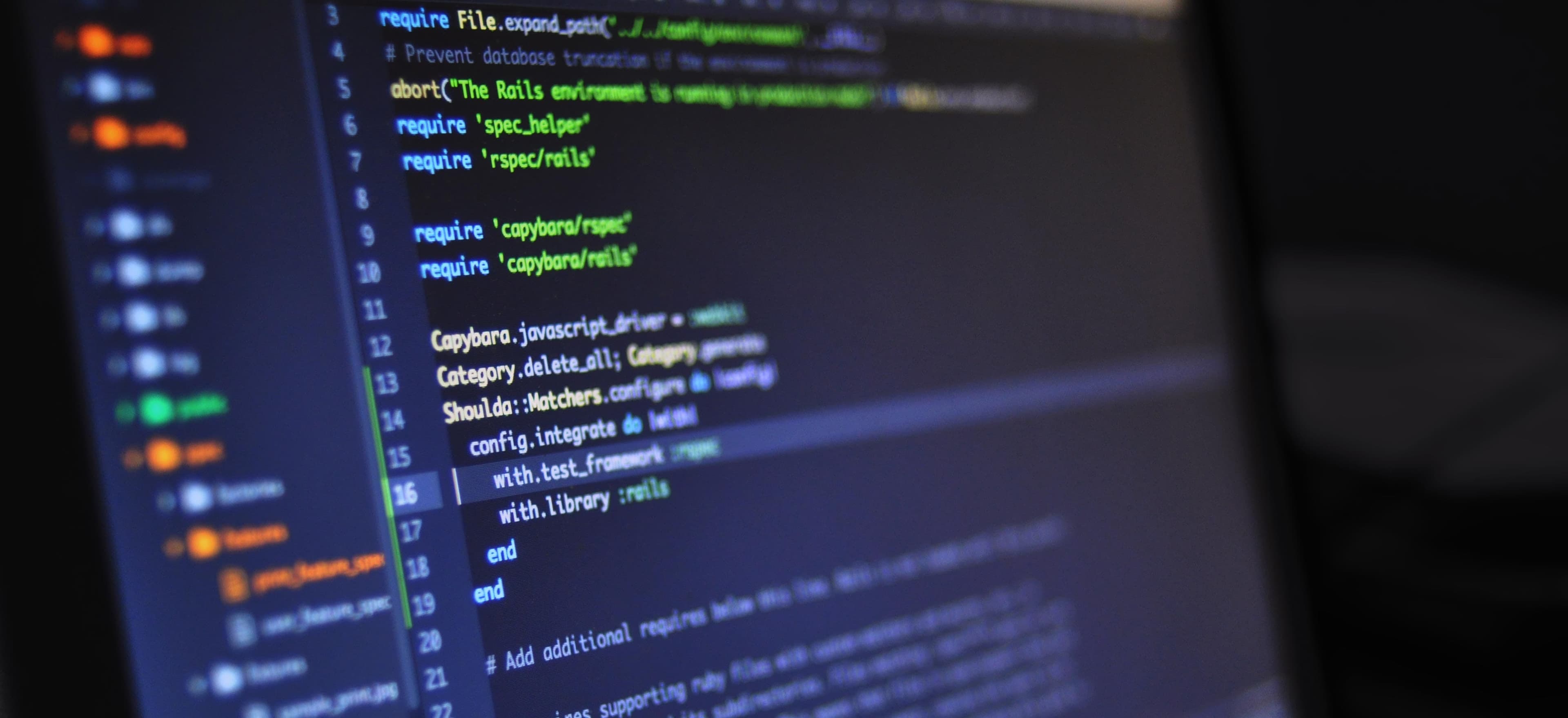
- Published on
Solving Common Issues with the Android Camera API
The Approach
Working with the Android Camera API can be a crucial and challenging task for developers, as it involves addressing various common issues related to camera functionality. These issues often include handling camera permissions, opening the camera device safely, managing different camera orientations, capturing high-quality images, and efficiently processing camera output. These challenges not only impact the functionality of the app but also affect the overall user experience. This guide aims to provide solutions to these common issues, ensuring developers can enhance their app's camera capabilities and provide a seamless user experience.
Understanding the Android Camera API
Overview of Camera API vs. Camera2 API
Android offers two primary camera APIs: the Camera API and the Camera2 API. The original Camera API is now deprecated, and developers are encouraged to use the Camera2 API for new projects. The Camera2 API provides more extensive control over camera functionality, including manual control over camera settings, improved performance, and support for additional features such as RAW image capture and burst mode. It also addresses some of the limitations present in the older Camera API, making it a preferred choice for developers looking to leverage the full potential of the device's camera hardware.
Key Concepts of Camera2 API
The Camera2 API introduces several key concepts that developers must understand to effectively work with the camera functionality. These concepts include camera sessions, capture requests, and image readers. Camera sessions represent the connection between the application and the camera device, providing a platform for capturing images and processing camera data. Capture requests are used to configure the capture of a single image, including setting exposure, focus, and other parameters. Image readers facilitate the acquisition of image data from the camera for further processing or storage.
Common Issues and Solutions
Let's address the common issues related to the Android Camera API and provide comprehensive solutions for each problem.
Issue 1: Handling Camera Permissions
Explanation
Starting from Android 6.0 (Marshmallow) and later, apps must request camera permissions at runtime to access the device's camera. Without proper permission handling, attempting to access the camera will result in a security exception, and the app's behavior will be affected.
Solution with Code Snippet
// Check for camera permissions
if (ContextCompat.checkSelfPermission(this, Manifest.permission.CAMERA) != PackageManager.PERMISSION_GRANTED) {
// Request camera permissions
ActivityCompat.requestPermissions(this, new String[] {Manifest.permission.CAMERA}, CAMERA_PERMISSION_REQUEST_CODE);
} else {
// Camera permissions have been granted
openCamera();
}
In the code snippet above, we first check if the camera permissions have been granted. If not, we request the necessary permissions from the user using ActivityCompat.requestPermissions()
. Once the permissions are granted, the openCamera()
method can be called to proceed with accessing the camera.
Issue 2: Opening the Camera Device Safely
Explanation
When opening the camera device, complications such as resource contention with other apps can occur. It's essential to handle the camera device safely to avoid conflicts and ensure proper disconnection from the camera when not in use.
Solution with Code Snippet
CameraManager manager = (CameraManager) getSystemService(Context.CAMERA_SERVICE);
try {
// Attempt to open the camera device
String cameraId = manager.getCameraIdList()[0];
manager.openCamera(cameraId, new CameraDevice.StateCallback() {
@Override
public void onOpened(@NonNull CameraDevice camera) {
// Camera is successfully opened, perform further operations
// ...
}
@Override
public void onDisconnected(@NonNull CameraDevice camera) {
// Handle camera disconnection
// ...
}
@Override
public void onError(@NonNull CameraDevice camera, int error) {
// Handle camera errors
// ...
}
}, null);
} catch (CameraAccessException e) {
e.printStackTrace();
}
In this code snippet, we use the CameraManager
to obtain access to the camera device. We then attempt to open the camera device using manager.openCamera()
, providing a CameraDevice.StateCallback
to handle the camera's state changes. This ensures that the camera device is safely opened and that appropriate actions are taken in the event of disconnection or errors.
Issue 3: Handling Different Camera Orientations
Explanation
One of the critical aspects of working with the camera is handling different device orientations. It's essential to capture images or videos that correctly match the orientation in which the device is being held, providing a seamless user experience.
Solution with Code Snippet
// Determine the device's orientation
int deviceOrientation = getWindowManager().getDefaultDisplay().getRotation();
// Determine the required rotation for the captured image
int rotation = getRotationCompensation(cameraId, deviceOrientation);
CaptureRequest.Builder captureBuilder = cameraDevice.createCaptureRequest(CameraDevice.TEMPLATE_STILL_CAPTURE);
captureBuilder.set(CaptureRequest.JPEG_ORIENTATION, rotation);
In the code snippet above, we retrieve the device's current orientation using getWindowManager().getDefaultDisplay().getRotation()
. We then calculate the required rotation for the captured image using the getRotationCompensation()
method and apply it to the capture request using captureBuilder.set(CaptureRequest.JPEG_ORIENTATION, rotation)
. This ensures that the captured image aligns with the device's orientation.
Issue 4: Capturing High-Quality Images
Explanation
Capturing high-quality images involves considering factors such as resolution settings and processing options. It's crucial to configure the camera settings to capture images at the desired quality and resolution.
Solution with Code Snippet
// Configure the image reader to capture high-quality images
imageReader = ImageReader.newInstance(imageWidth, imageHeight, ImageFormat.JPEG, MAX_IMAGES);
imageReader.setOnImageAvailableListener(onImageAvailableListener, backgroundHandler);
// Create a capture request for capturing high-quality images
CaptureRequest.Builder captureBuilder = cameraDevice.createCaptureRequest(CameraDevice.TEMPLATE_STILL_CAPTURE);
captureBuilder.addTarget(imageReader.getSurface());
In this code snippet, we create an ImageReader
to capture high-quality JPEG images at a specified width and height. We configure the ImageReader
with an onImageAvailableListener
to handle the captured images. Additionally, we create a capture request targeting the ImageReader
's surface, ensuring that the camera captures images at the desired quality and resolution.
Issue 5: Efficient Background Processing
Explanation
Efficiently processing camera output in the background is essential for maintaining a smooth user interface. This involves using background threads or handlers to handle camera data without blocking the main thread.
Solution with Code Snippet
// Create a handler for processing camera data on a background thread
HandlerThread backgroundThread = new HandlerThread("CameraBackground");
backgroundThread.start();
backgroundHandler = new Handler(backgroundThread.getLooper());
// Prepare for capturing images in the background
cameraDevice.createCaptureSession(Arrays.asList(imageReader.getSurface()), new CameraCaptureSession.StateCallback() {
@Override
public void onConfigured(@NonNull CameraCaptureSession session) {
// Camera session is successfully configured, continue with image capture
// ...
}
@Override
public void onConfigureFailed(@NonNull CameraCaptureSession session) {
// Handle camera session configuration failure
// ...
}
}, backgroundHandler);
In this code snippet, we create a background thread for processing camera data using a HandlerThread
. We then use the backgroundHandler
to execute operations related to capturing images and processing camera output. The CameraCaptureSession.StateCallback
ensures that the camera session is properly configured to capture images in the background.
Best Practices for Working with the Camera2 API
When working with the Camera2 API, developers are encouraged to follow these best practices:
- Properly handle camera permissions to ensure the app adheres to runtime permission requirements.
- Manage camera resources efficiently, including safely opening and releasing the camera device when not in use.
- Implement error handling for various camera-related operations, providing informative feedback to users in case of failures.
- Optimize battery usage by gracefully managing camera usage and releasing resources when not needed.
- Test the app's compatibility across different Android devices to ensure consistent behavior and performance.
My Closing Thoughts on the Matter
In conclusion, addressing common issues related to the Android Camera API is crucial for developing apps with robust camera capabilities and providing an excellent user experience. By implementing the solutions provided in this guide, developers can overcome challenges such as handling camera permissions, safely opening the camera device, managing different camera orientations, capturing high-quality images, and processing camera output efficiently. Experimenting with these solutions and adapting them to specific app requirements can significantly enhance the camera functionality of Android apps.
We encourage developers to explore the Android Camera2 API documentation and leverage other resources available to further enhance their understanding of camera-related development on the Android platform.
Further Reading and Resources
For further reading and resources on working with the Android Camera2 API and addressing camera-related issues, we recommend the following:
- Android Camera2 API Documentation
- CameraView: A well-documented library for simplifying Camera2 API usage
- Android Camera2 Sample Project on GitHub
- Stack Overflow: A community of developers discussing Android camera-related issues
By addressing common issues and providing comprehensive solutions for working with the Android Camera2 API, this article aims to equip developers with the knowledge and tools necessary to enhance their app's camera functionality. Whether it's capturing high-quality images, efficiently processing camera output, or managing camera permissions, following best practices and implementing the solutions outlined in this guide can contribute to the development of robust and user-friendly camera features within Android apps.