Turning Bugs Into Features: A Developer's Guide
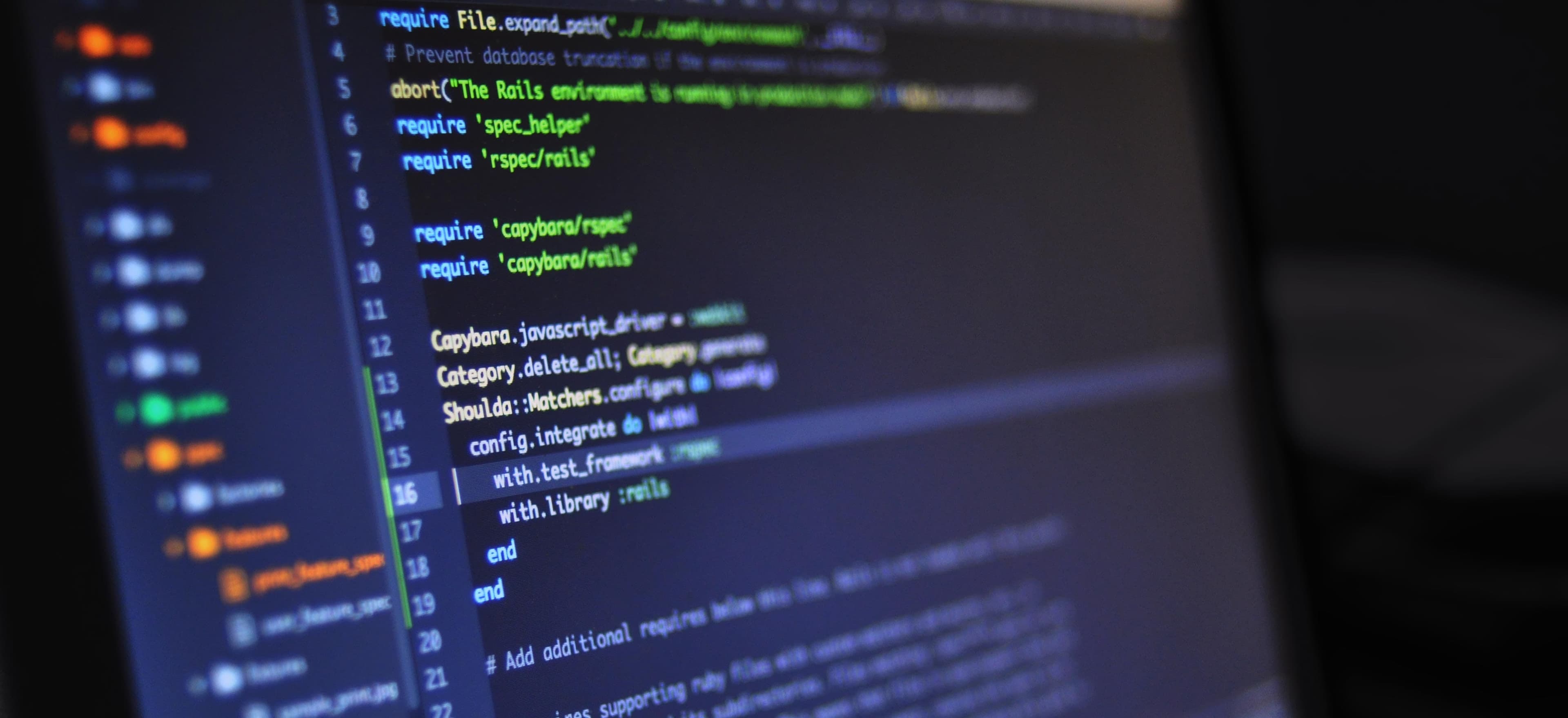
- Published on
Turning Bugs Into Features: A Developer's Guide in Java
In the fast-paced world of software development, Java remains a stalwart, powering millions of applications worldwide. From Android apps to enterprise-level systems, Java's versatility and efficiency make it a favorite among developers. However, even in the most meticulously crafted Java applications, bugs are an inevitable part of the development lifecycle. But what if we could turn these bugs into features? In this guide, we will explore how to leverage Java's robust ecosystem to transform mistakes into opportunities for enhancement, thereby elevating your coding craftsmanship.
Understanding Bugs in Java
Before diving into the metamorphosis of bugs into features, it's vital to understand the nature of bugs in Java applications. Bugs can range from simple syntax errors, which are easily caught by the compiler, to more complex issues like memory leaks or concurrency problems which can be elusive and challenging to diagnose.
Embrace the Bug
The first step in transforming bugs into features is to embrace the bug. Recognizing that bugs are not merely obstacles but opportunities for improvement is crucial. This mindset shift is the foundation of innovative problem-solving in software development.
Debugging with Purpose
Effective debugging is an art that every Java developer should master. Tools such as the integrated debugger in the Eclipse IDE or IntelliJ IDEA provide powerful functionalities to step through code, inspect variables, and evaluate expressions on the fly.
Example: Identifying a Concurrency Issue
Consider a scenario where your Java application faces inconsistent behavior during high concurrency operations. After several debugging sessions, you isolate the issue to a block of code that is not thread-safe.
public class InventoryCounter {
private int items = 0;
public void addItem() {
items++;
}
public void removeItem() {
items--;
}
public int getItems() {
return items;
}
}
The Solution
To resolve this, you refactor the code to use synchronization, ensuring that only one thread can modify the 'items' variable at a time, thus preventing the concurrency issue.
public class InventoryCounter {
private int items = 0;
public synchronized void addItem() {
items++;
}
public synchronized void removeItem() {
items--;
}
public synchronized int getItems() {
return items;
}
}
Why This Works
Synchronization in Java is a fundamental mechanism for controlling access to resources by multiple threads. By making the methods synchronized, we ensure thread safety, turning a concurrency bug into an opportunity to reinforce the application's reliability.
Refactoring: A Gateway to Enhanced Features
Refactoring is not just about fixing bugs; it's an avenue to revisit and improve the existing codebase, potentially adding new functionalities or optimizing performance. The discovery of a bug can often illuminate areas of the code that are ripe for refactoring.
Example: Enhancing Performance
Imagine you've discovered a performance bottleneck in your Java application, traced to an inefficient method of processing large datasets.
Initially, the method might look something like this:
public List<String> processData(List<String> data) {
List<String> processedData = new ArrayList<>();
for(String item : data) {
// Time-consuming processing
processedData.add(expensiveOperation(item));
}
return processedData;
}
The Improved Approach
By identifying this bottleneck, you have the opportunity to refactor the method to use Java's Stream
API, significantly improving its performance, especially for large datasets.
public List<String> processData(List<String> data) {
return data.parallelStream()
.map(this::expensiveOperation)
.collect(Collectors.toList());
}
Why This Improvement Matters
The use of parallelStream()
enables the method to process data in parallel, taking advantage of multi-core processors. This not only addresses the performance issue but also showcases how a bug can lead to the optimization of an application, enhancing its overall efficiency.
Learning Resources
As you journey through turning bugs into features, leveraging the wealth of learning resources available can be incredibly beneficial. The Official Java Documentation provides comprehensive insights into Java's core concepts, while platforms like Stack Overflow offer a community-driven approach to solving specific issues and exchanging knowledge.
Final Thoughts
Transforming bugs into features is a testament to a developer's resilience and creativity. It challenges us to see beyond the immediate frustrations and recognize the potential for growth and innovation. By embracing this philosophy, Java developers can not only enhance their applications but also evolve in their journey as software craftsmen.
Remember, the next time you encounter a bug in your Java code, it may just be an undiscovered feature waiting to shine. Happy coding!