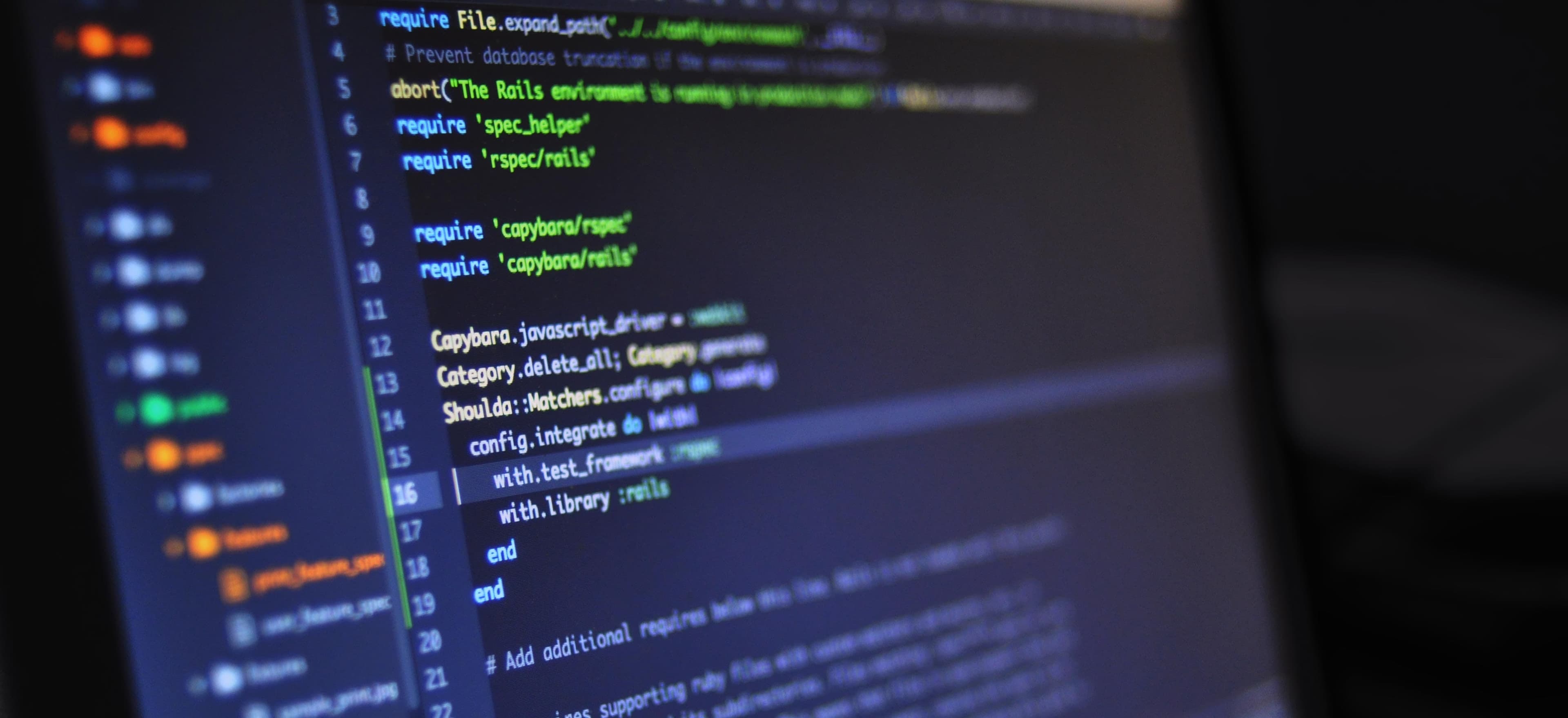
- Published on
Securing Spring: The Ultimate Guide to Password Protection
In today's digital age, security is paramount. With the prevalence of cyber threats, ensuring that sensitive information is protected has become a top priority for developers. When it comes to building applications in Java, the Spring Framework is a popular choice due to its robust features and ease of use. One critical aspect of application security is password protection. In this comprehensive guide, we will delve into the best practices for securing passwords in a Spring application.
Why Password Protection Matters
Passwords are the most common form of authentication used to protect user accounts. However, if not handled correctly, passwords can become a vulnerability. Weak or poorly protected passwords can lead to unauthorized access, data breaches, and compromised user accounts. It is essential for developers to implement strong password protection mechanisms to safeguard user credentials and maintain the integrity of their applications.
Best Practices for Password Protection
1. Password Hashing
Storing passwords in plain text is never a good idea. In the event of a data breach, plaintext passwords can be easily exploited. Instead, passwords should be securely hashed and salted before being stored in a database. Hashing algorithms such as bcrypt or Argon2 are designed for secure password storage and are resistant to brute force attacks. Let's take a look at an example of how password hashing can be implemented using Spring Security:
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
BCryptPasswordEncoder passwordEncoder = new BCryptPasswordEncoder();
String rawPassword = "mysecurepassword";
String encodedPassword = passwordEncoder.encode(rawPassword);
System.out.println(encodedPassword);
In this example, the BCryptPasswordEncoder
from Spring Security is used to hash the password "mysecurepassword". The resulting hash is then stored in the database. This ensures that even if the database is compromised, the original passwords remain secure.
2. Password Policies
Enforcing strong password policies is crucial for preventing weak passwords. Developers should mandate the use of complex passwords that include a mix of uppercase and lowercase letters, numbers, and special characters. Additionally, implementing password expiration and history policies can further enhance security by prompting users to change their passwords regularly and preventing them from reusing old passwords.
3. Two-Factor Authentication
Implementing two-factor authentication (2FA) provides an extra layer of security on top of passwords. In addition to something the user knows (their password), 2FA requires an additional form of verification, such as a one-time code sent to their mobile device or generated by an authenticator app. Spring Security provides robust support for integrating 2FA into applications, offering flexibility in using various authentication methods.
Implementing Password Protection in a Spring Application
Now that we've covered the best practices for password protection, let's delve into how these principles can be applied within a Spring application.
1. Using Spring Security
Spring Security is a powerful and customizable security framework that provides comprehensive authentication and authorization capabilities. By integrating Spring Security into a Spring application, developers can easily implement password protection mechanisms.
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth
.inMemoryAuthentication()
.withUser("user")
.password("{noop}password")
.roles("USER");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.and()
.httpBasic();
}
}
In this example, we configure a simple in-memory authentication with a username "user" and password "password". The {noop}
prefix indicates that the password is stored in plaintext. While storing plaintext passwords is not recommended for production applications, this example serves to illustrate the basic configuration of authentication using Spring Security.
2. Customizing Password Encoding
To apply password hashing with Spring Security, we can customize the password encoding process by defining a PasswordEncoder
bean in our configuration.
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
By defining a PasswordEncoder
bean, Spring Security will automatically use the specified hashing algorithm to encode passwords before storing them in the database.
3. Implementing Two-Factor Authentication
Integrating two-factor authentication with Spring Security can be achieved by utilizing its extensible authentication mechanisms. By incorporating additional verification steps, such as SMS-based codes or TOTP (Time-based One-Time Password) authentication, developers can enhance the security of user accounts.
The Closing Argument
In conclusion, securing passwords in a Spring application is a critical aspect of application security. By following best practices such as password hashing, enforcing strong password policies, and implementing two-factor authentication, developers can significantly bolster the security of user credentials. Furthermore, leveraging the capabilities of Spring Security allows for seamless integration of robust password protection mechanisms within Spring applications.
Implementing effective password protection not only safeguards user accounts but also instills trust and confidence in the application. As cyber threats continue to evolve, it is imperative for developers to prioritize the security of user passwords and stay vigilant in fortifying their applications against potential risks.
By adhering to the principles outlined in this guide and harnessing the capabilities of Spring Security, developers can build resilient and secure applications that inspire confidence and protect user data.
To further enhance your understanding of Spring Security and password protection, I recommend exploring the official Spring Security documentation and OWASP Password Storage Cheat Sheet. These resources offer valuable insights and guidance for implementing robust password protection in your Spring applications.