Master Apache Kafka: Essential Cheatsheet for Beginners
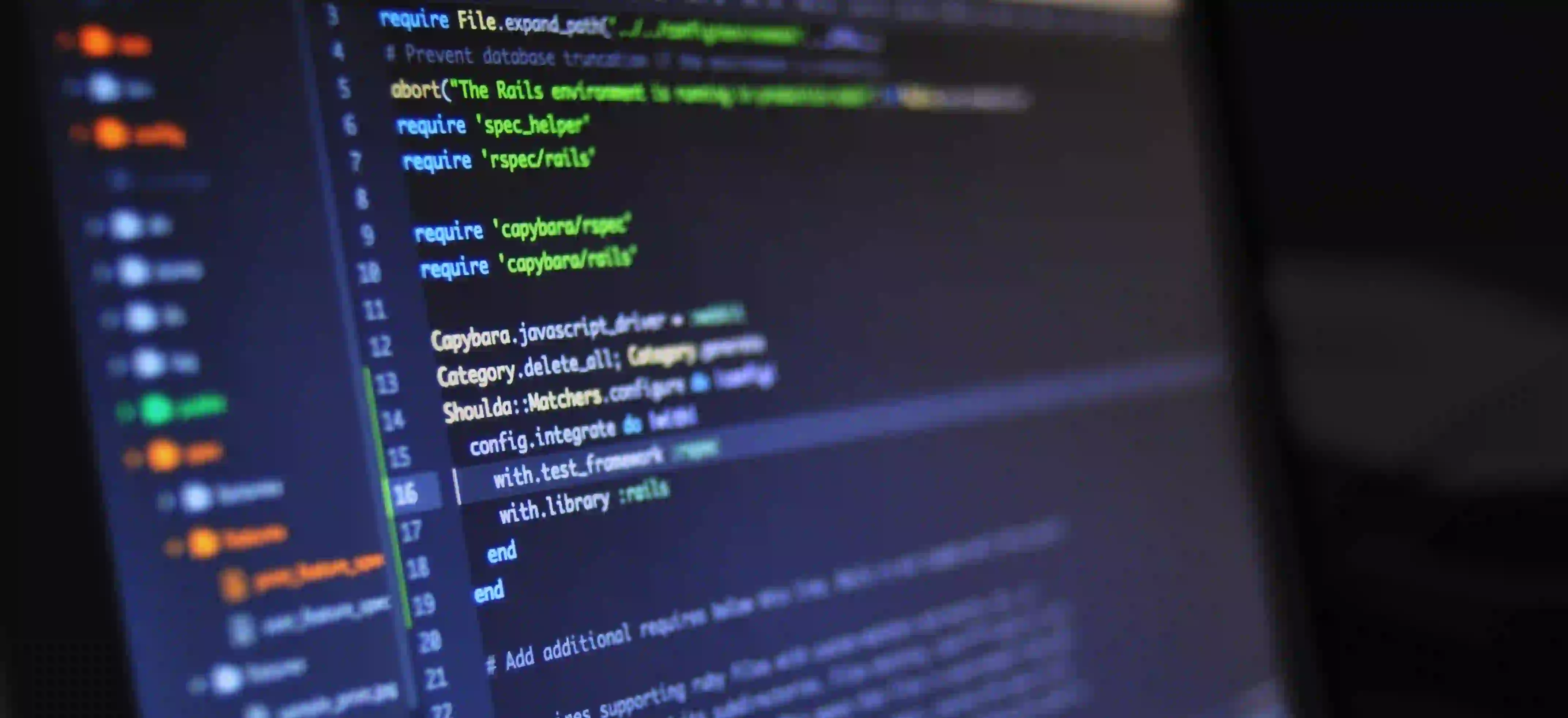
Master Apache Kafka: Essential Cheatsheet for Beginners
Apache Kafka is a popular distributed streaming platform that is known for its high-throughput, fault-tolerance, and scalability. In this article, we will explore the basics of Apache Kafka, its architecture, and essential concepts for beginners.
What is Apache Kafka?
Apache Kafka is a distributed event streaming platform that is used for building real-time data pipelines and streaming applications. It is designed to handle high-throughput, fault-tolerance, and allows for the storage and processing of streams of records in a fault-tolerant and durable manner.
Key Concepts in Apache Kafka
1. Topics
In Apache Kafka, data is organized and distributed into topics. A topic is a category or feed name to which records are published. Topics in Kafka are always multi-subscriber, which means that a topic can have zero, one, or many consumers that subscribe to the data written to it.
2. Producers
Producers are responsible for publishing records to Kafka topics. They push data into Kafka topics for consumption by the consumers.
3. Consumers
Consumers read data from Kafka topics by subscribing to them. They process the data published by the producers and perform various operations on it.
4. Brokers
Kafka is designed as a cluster-centric system, where one or more servers in the cluster act as brokers. Kafka brokers are responsible for maintaining the published data in topics and serving the consumers.
5. Partitions
Each topic in Kafka is divided into partitions, which allows the data to be distributed across multiple brokers. Partitions enable parallelism and scalability in processing data.
Setting up Apache Kafka
To get started with Apache Kafka, you need to set up a Kafka cluster. You can either choose to set up Kafka on a single machine for development and testing purposes, or you can set up a multi-broker Kafka cluster for production use.
Kafka Installation
First, download the Apache Kafka binaries from the official website. Once downloaded, extract the files to your desired location on your machine.
Starting Zookeeper
Apache Kafka uses Zookeeper for managing and coordinating the Kafka brokers. Start the Zookeeper server using the following command:
bin/zookeeper-server-start.sh config/zookeeper.properties
Starting Kafka Broker
Next, start the Kafka broker using the following command:
bin/kafka-server-start.sh config/server.properties
After following these steps, you should have a basic Kafka setup running on your machine.
Producing and Consuming Messages
Now that you have a Kafka cluster set up, let's look at how to produce and consume messages using Kafka.
Producing Messages
To produce messages to a Kafka topic, you can use the Kafka command-line utilities or write a simple producer application in Java.
Here's an example of a simple Kafka producer application in Java:
import org.apache.kafka.clients.producer.*;
import java.util.Properties;
public class KafkaProducerExample {
public static void main(String[] args) {
Properties properties = new Properties();
properties.put("bootstrap.servers", "localhost:9092");
properties.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
properties.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
Producer<String, String> producer = new KafkaProducer<>(properties);
ProducerRecord<String, String> record = new ProducerRecord<>("my_topic", "key", "Hello, Kafka!");
producer.send(record);
producer.close();
}
}
In this example, we use the Kafka Java client to create a producer and send a message to the "my_topic" topic.
Consuming Messages
Similarly, consuming messages from a Kafka topic can be accomplished using the Kafka command-line utilities or by writing a simple consumer application in Java.
Here's an example of a simple Kafka consumer application in Java:
import org.apache.kafka.clients.consumer.*;
import org.apache.kafka.common.serialization.StringDeserializer;
import java.util.Collections;
import java.util.Properties;
public class KafkaConsumerExample {
public static void main(String[] args) {
Properties properties = new Properties();
properties.put("bootstrap.servers", "localhost:9092");
properties.put("key.deserializer", StringDeserializer.class.getName());
properties.put("value.deserializer", StringDeserializer.class.getName());
properties.put("group.id", "my_consumer_group");
Consumer<String, String> consumer = new KafkaConsumer<>(properties);
consumer.subscribe(Collections.singletonList("my_topic"));
while (true) {
ConsumerRecords<String, String> records = consumer.poll(100);
for (ConsumerRecord<String, String> record : records) {
System.out.printf("Offset = %d, Key = %s, Value = %s%n", record.offset(), record.key(), record.value());
}
}
}
}
In this example, we create a consumer and subscribe to the "my_topic" topic to receive and process messages from Kafka.
Lessons Learned
In this article, we've covered the basics of Apache Kafka, including its key concepts, setting up a Kafka cluster, and producing/consuming messages using Kafka's Java client. Apache Kafka's robust architecture and high scalability make it a popular choice for real-time stream processing.
With the essential concepts and examples provided, you should now have a good foundation to start exploring and utilizing Apache Kafka for your streaming and real-time data processing needs. Happy streaming!