Overcoming Hurdles in Adopting OpenAPI v3.0 for JAX-RS APIs
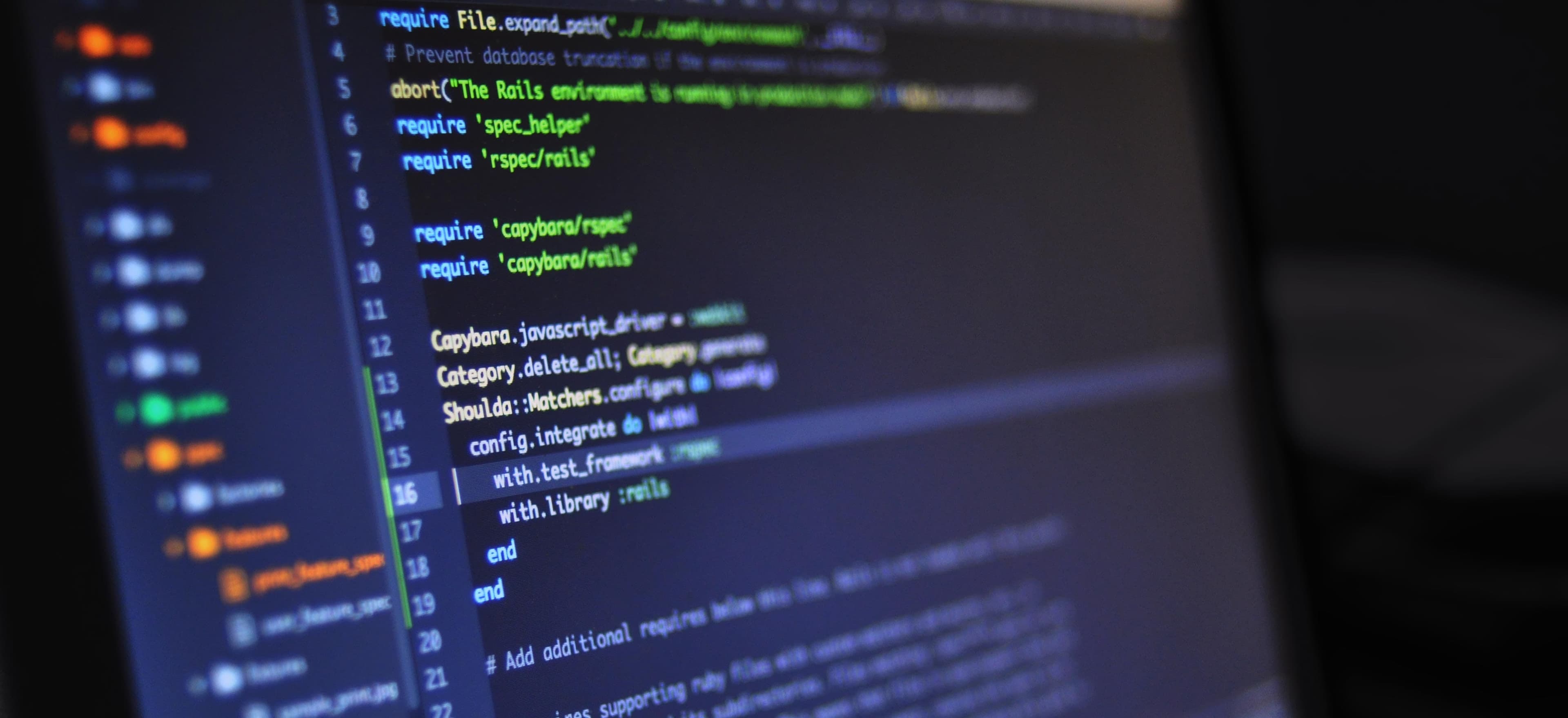
- Published on
Overcoming Hurdles in Adopting OpenAPI v3.0 for JAX-RS APIs
In the evolving landscape of web services, OpenAPI Specification (OAS) v3.0 emerges as a pivotal standard for defining and documenting RESTful APIs. It simplifies the design, development, testing, and management of API interfaces, enabling better communication between stakeholders and the technology. For Java developers, especially those leveraging JAX-RS (Java API for RESTful Web Services) for creating RESTful web services, transitioning to OpenAPI v3.0 from older specifications or other documentation formats offers numerous benefits but also poses several challenges. In this comprehensive guide, we explore strategies to effortlessly overcome these hurdles, ensuring a smooth adoption of OpenAPI v3.0 for your JAX-RS APIs.
Understanding the Shift to OpenAPI v3.0
OpenAPI v3.0 isn't just an upgrade from its predecessors—it's a significant evolution that introduces enhanced capabilities for describing APIs. These include but are not limited to:
- Improved support for polymorphism.
- Richer semantics for describing RESTful APIs.
- Advanced security scheme declarations.
Before diving into the integration specifics, it's vital to grasp why this shift matters. The enhanced features of OpenAPI v3.0 facilitate more precise API descriptions, leading to better-generated documentation and client SDKs. This directly translates to reduced integration time and fewer misunderstandings between frontend and backend teams, a critical factor in agile and DevOps environments.
Challenges in Adopting OpenAPI v3.0 for JAX-RS
While the advantages are clear, several hurdles often deter JAX-RS API developers:
- Lack of Familiarity: OpenAPI v3.0 introduces new elements and structures, requiring a learning curve.
- Integration Complexity: Bridging JAX-RS with OpenAPI v3.0 is not always straightforward, given the technical differences.
- Tooling and Support: Finding the right set of tools to facilitate this transition can be daunting.
Tackling the Challenges Head-On
1. Invest Time in Understanding OpenAPI v3.0
The first step is foundational but crucial. Spend time with the OpenAPI Specification, understanding its components, such as Paths
, Operations
, Parameters
, Responses
, and Security Schemes
. Tools like Swagger Editor can be incredibly beneficial during this phase, offering real-time feedback and visualization.
2. Choose the Right Integration Tools
Several tools can ease the integration of JAX-RS with OpenAPI v3.0:
- Swagger Core: Enables the generation of OpenAPI definitions from JAX-RS annotations.
- SmallRye: A MicroProfile-based framework that offers seamless integration with OpenAPI.
Here's a snippet showing how you can use annotations in your JAX-RS application for OpenAPI documentation:
@Path("/users")
@Produces(MediaType.APPLICATION_JSON)
public class UserResource {
@GET
@Operation(summary = "List all users",
description = "Returns a list of users.",
responses = {
@ApiResponse(responseCode = "200", description = "Successful retrieval",
content = @Content(array = @ArraySchema(schema = @Schema(implementation = User.class))))})
public Response getAllUsers() {
// Implementation goes here
}
}
In this example, @Operation
and @ApiResponse
annotations from the Swagger Core library enrich the JAX-RS endpoint with OpenAPI semantics. This approach not only documents the API but does so in a way that's closer to the code, facilitating maintainability and accuracy.
3. Embrace the Learning Curve
Integrating OpenAPI v3.0 with JAX-RS is as much about technical adaptation as it is about cultural shift within the development team. Encourage knowledge sharing sessions, workshops, and pairing to disseminate OpenAPI knowledge across the team. Practical exposure combined with theoretical understanding accelerates the learning curve.
Overcoming Tooling and Support Hurdles
One of the most common concerns is the selection of tools that support OpenAPI v3.0 for JAX-RS. Beyond Swagger Core and SmallRye, consider exploring:
- Quarkus: Known for its container-first approach, Quarkus offers built-in support for OpenAPI and Swagger UI making it a go-to choice for modern Java applications.
- Eclipse MicroProfile: An open-source community specification that integrates OpenAPI, among other technologies, with enterprise Java microservices.
Each tool or framework comes with its peculiarities. For instance, Quarkus provides extensions for automatically generating and serving OpenAPI documents and Swagger UI.
import org.eclipse.microprofile.openapi.annotations.OpenAPIDefinition;
import org.eclipse.microprofile.openapi.annotations.info.Info;
@OpenAPIDefinition(info = @Info(title = "User API", version = "1.0"))
public class UserApplication extends Application {
}
This snippet shows how MicroProfile OpenAPI annotations directly interact with your JAX-RS application, providing an elegant way to define API-wide metadata.
Closing the Chapter
Adopting OpenAPI v3.0 for JAX-RS APIs heralds a substantial leap toward more robust, maintainable, and accessible web services. Despite the initial learning curve and integration challenges, the payoff in terms of API quality, team collaboration, and reduced time-to-market is unequivocal. By understanding OpenAPI v3.0's benefits, selecting appropriate tools, and fostering a culture of continuous learning, teams can navigate these hurdles successfully.
Remember, the journey to adopting OpenAPI v3.0 is a process of evolution, not a one-off event. Start small, iterate, and continuously seek feedback to refine your approach. With persistence and the right strategies, your JAX-RS APIs will thrive in the ecosystem of modern web services.
Checkout our other articles