Overcoming DSL Integration Hurdles with ANTLR4 and PrimeFaces
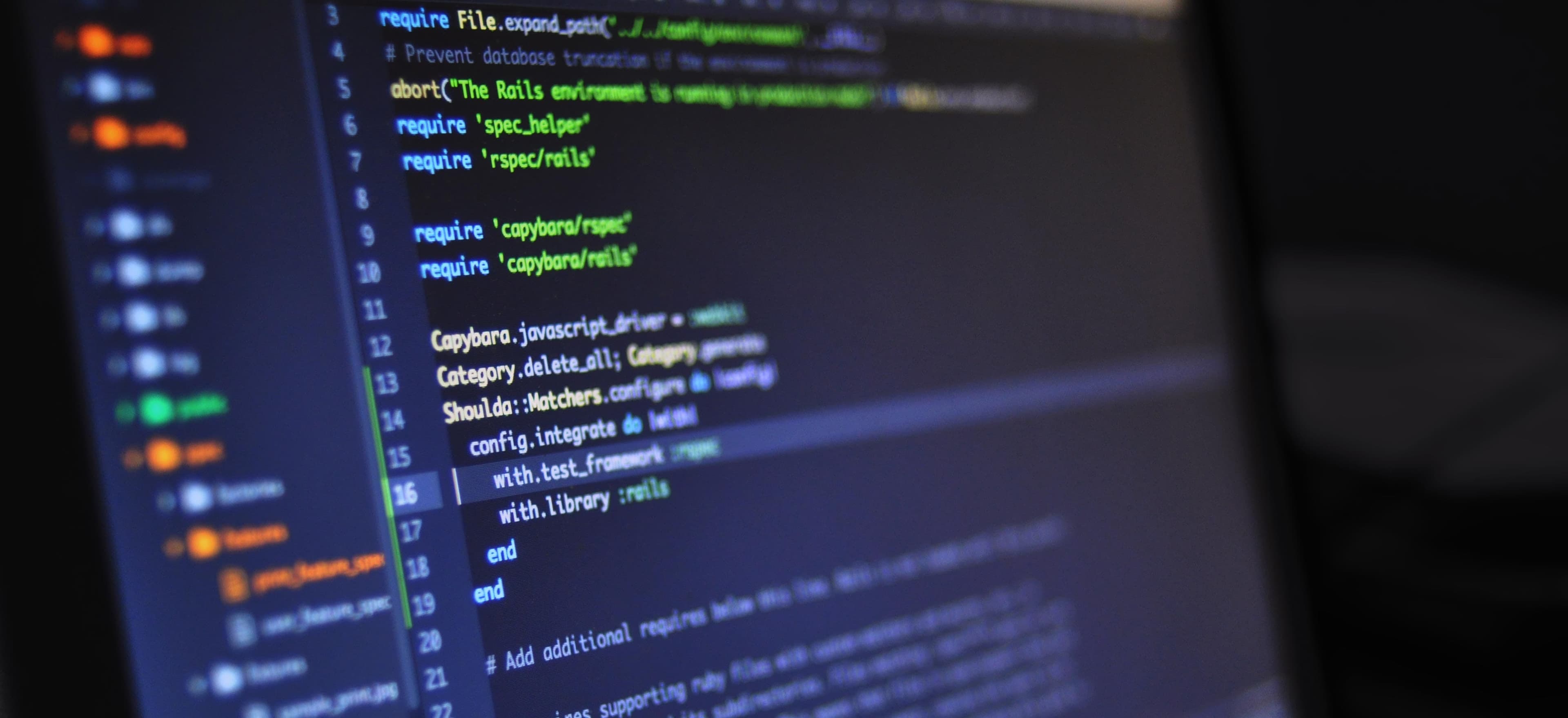
- Published on
Overcoming DSL Integration Hurdles with ANTLR4 and PrimeFaces
In the world of software development, creating domain-specific languages (DSLs) is a powerful tool for crafting concise, intuitive code tailored to specific problem domains. However, integrating DSLs into existing applications can often pose significant challenges. In this post, we'll explore how to leverage ANTLR4 and PrimeFaces to overcome these hurdles and seamlessly integrate DSLs into Java applications.
Understanding ANTLR4
ANTLR (ANother Tool for Language Recognition) is a powerful parser generator that can be used to build parsers, interpreters, and translators for domain-specific languages. ANTLR4, the most recent version of the tool, provides a robust framework for creating and working with DSLs in Java.
With ANTLR4, developers can define the grammar of a DSL using a concise notation, which is then used to automatically generate parser and lexer classes. This greatly simplifies the process of parsing and interpreting DSL code, allowing for seamless integration into Java applications.
Let's consider a simple DSL for representing mathematical expressions, such as addition, subtraction, multiplication, and division. Using ANTLR4, we can define the grammar for this DSL as follows:
grammar MathExpression;
expression : addition ;
addition : multiplication ('+' multiplication)* ;
multiplication : atom ('*' atom)* ;
atom : Number | '(' expression ')' ;
Number : [0-9]+ ;
In this grammar, we specify the rules for constructing mathematical expressions, including the hierarchy of operations and the handling of parentheses. Once the grammar is defined, ANTLR4 can automatically generate the corresponding parser and lexer classes, saving us the effort of writing these components from scratch.
Integrating ANTLR4 with PrimeFaces
PrimeFaces is a popular open-source user interface (UI) component library for JavaServer Faces (JSF) applications. With a rich set of UI components and seamless integration with JSF, PrimeFaces provides a strong foundation for building dynamic and interactive web applications.
To integrate our DSL parsing capabilities with PrimeFaces, we can create a web application that allows users to input DSL code representing mathematical expressions, and then parse and evaluate these expressions using ANTLR4. We'll start by creating a simple JSF form with an input field for the DSL code and a button to trigger the parsing process.
<h:form>
<p:inputTextarea id="dslInput" value="#{dslBean.dslCode}" rows="5" cols="60"/>
<p:commandButton value="Parse" action="#{dslBean.parseDSL}" update="output"/>
</h:form>
In this example, we use a p:inputTextarea
component from PrimeFaces to capture the DSL code input by the user. When the "Parse" button is clicked, it triggers the parseDSL
method in the dslBean
managed bean, which will use ANTLR4 to parse the input DSL code and generate the output.
Let's take a look at the parseDSL
method in the managed bean:
public void parseDSL() {
String input = dslCode;
MathExpressionLexer lexer = new MathExpressionLexer(CharStreams.fromString(input));
CommonTokenStream tokens = new CommonTokenStream(lexer);
MathExpressionParser parser = new MathExpressionParser(tokens);
ParseTree tree = parser.expression();
// ... perform evaluation or further processing based on the parse tree
// Update the output panel with the result or any error messages
}
In this method, we first retrieve the input DSL code from the dslCode
property. We then use the generated lexer and parser classes from ANTLR4 to tokenize and parse the DSL code, producing a parse tree that represents the structure of the input expression. Depending on the specific requirements of the application, we can perform evaluation or further processing based on the parse tree, and update the output panel with the result or any error messages.
Handling Errors and Providing Feedback
When integrating DSLs into Java applications, it's important to consider how to handle errors and provide feedback to the user. ANTLR4 provides robust support for error handling and recovery, allowing us to customize error messages and gracefully handle syntax errors in the input DSL code.
For example, we can extend the default ANTLRErrorStrategy to customize error handling in the parser:
public class CustomErrorStrategy extends DefaultErrorStrategy {
@Override
public void reportError(Parser recognizer, RecognitionException e) {
// Custom error reporting and recovery logic
}
}
By customizing the error reporting and recovery logic, we can provide meaningful feedback to the user when syntax errors are encountered, helping them understand and correct their DSL code effectively.
Final Thoughts
By leveraging ANTLR4 and PrimeFaces, developers can overcome the integration hurdles associated with DSLs and seamlessly incorporate domain-specific languages into Java applications. The powerful parsing capabilities of ANTLR4, combined with the rich UI components of PrimeFaces, enable the creation of intuitive and user-friendly interfaces for working with DSLs.
In summary, integrating DSLs with Java applications involves defining grammars using ANTLR4, creating web interfaces with PrimeFaces to capture and process DSL code, and providing effective error handling and feedback mechanisms. By following these best practices, developers can harness the full potential of DSLs and empower users to express complex ideas in a clear and concise manner within their Java applications.
In conclusion, ANTLR4 and PrimeFaces provide a robust foundation for integrating DSLs into Java applications, offering powerful parsing capabilities and rich UI components for building intuitive interfaces. By following best practices and leveraging the strengths of these tools, developers can overcome DSL integration hurdles and empower users to express complex ideas in a clear and concise manner within their Java applications.