Turning Data into Decisions: A Step-By-Step Guide
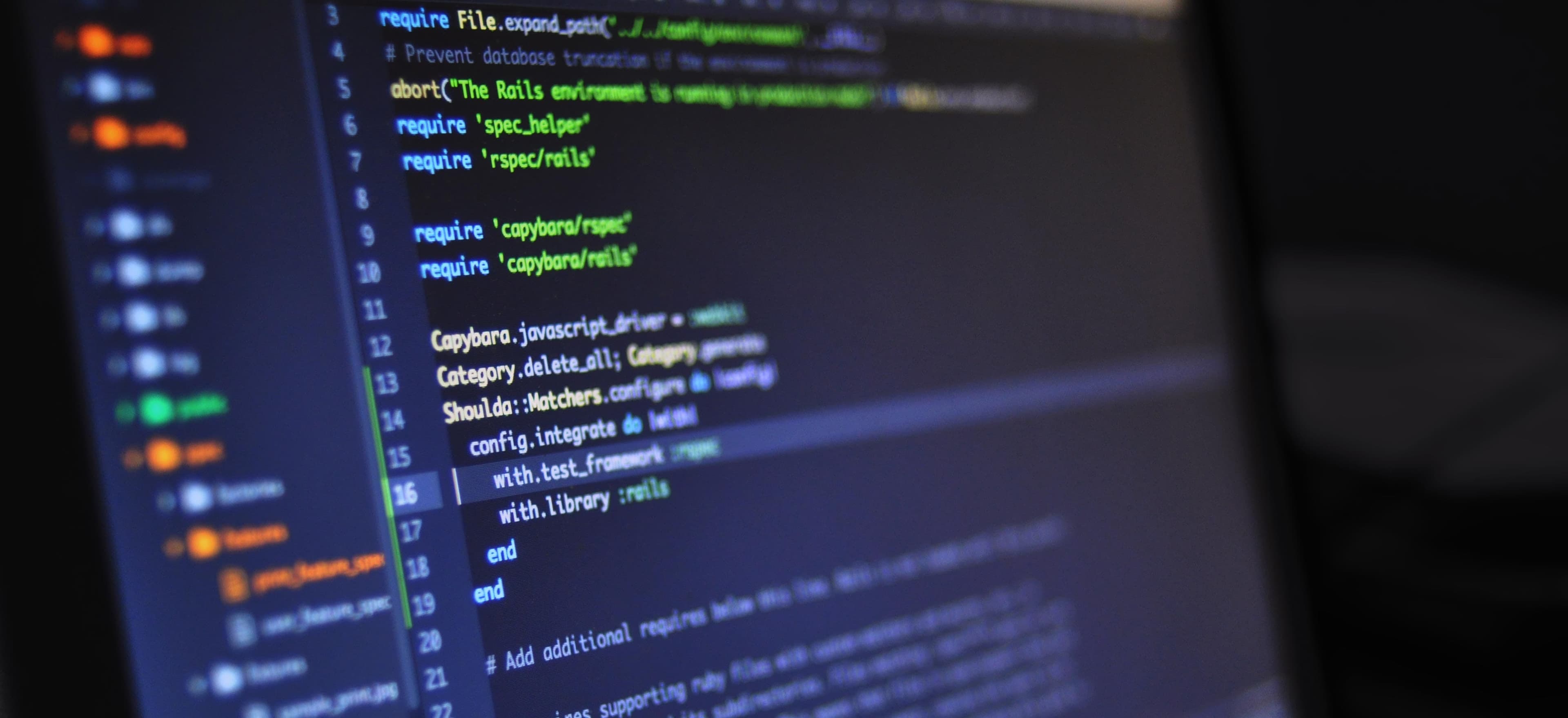
- Published on
Turning Data into Decisions: A Step-By-Step Guide
In today's data-driven world, businesses rely heavily on data to make informed decisions. Java, as a versatile and powerful programming language, plays a significant role in processing and analyzing data. In this blog post, we will explore how Java can be used to turn raw data into actionable insights, guiding you through the process step by step.
Step 1: Data Collection
The first step in the data analysis process is collecting relevant data. This can be done through various sources such as databases, web services, or files. In Java, you can use libraries like JDBC for database connectivity, Apache HTTP Client for web services, or Apache Commons IO for file operations.
// Example of collecting data from a database using JDBC
try (Connection connection = DriverManager.getConnection(url, username, password)) {
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM table");
// Process the data
} catch (SQLException e) {
// Handle any SQL exceptions
}
In the above code snippet, we use JDBC to connect to a database and execute a query to retrieve data. This demonstrates the use of Java for data collection from a database.
Step 2: Data Preprocessing
Once the data is collected, it often requires preprocessing before analysis. This may involve cleaning the data, handling missing values, or transforming the data into a suitable format. Java offers various libraries such as Apache Commons CSV for working with CSV files and Apache Commons Lang for string manipulation, which can be used for data preprocessing.
// Example of cleaning data using Apache Commons Lang
String uncleanData = "Example$%String";
String cleanData = StringUtils.strip(uncleanData, "$%"); // cleanData = "ExampleString"
Here, we use Apache Commons Lang to clean the data by removing unwanted characters from a string. This showcases how Java can be utilized for preprocessing data to make it suitable for analysis.
Step 3: Data Analysis
With the preprocessed data at hand, the next step is to perform analysis to derive meaningful insights. Java provides libraries such as Apache Commons Math and Weka that offer various statistical and machine learning algorithms for data analysis.
// Example of performing linear regression using Apache Commons Math
SimpleRegression regression = new SimpleRegression();
// Add data points to the regression
regression.addData(x, y);
double slope = regression.getSlope();
double intercept = regression.getIntercept();
In this example, we use Apache Commons Math to perform linear regression analysis on the data. Java's rich ecosystem of libraries makes it a powerful choice for data analysis tasks.
Step 4: Decision Making
The final step involves leveraging the insights gained from data analysis to make informed decisions. Java can be used to build decision support systems or integrate with business intelligence tools to facilitate decision-making processes.
// Example of using data insights for decision making
if (predictedSales > threshold) {
makeInvestmentDecision();
} else {
holdOffInvestment();
}
In the above code snippet, we depict a simplified decision-making process based on the insights obtained from data analysis. Java's versatility allows for the implementation of decision-making logic based on data-driven insights.
Lessons Learned
In this step-by-step guide, we have demonstrated how Java can be employed to turn raw data into informed decisions. From data collection to decision making, Java's rich ecosystem of libraries and powerful capabilities make it a compelling choice for data analysis and decision support. By following this guide, you can harness the power of Java to unlock the potential of your data and drive impactful decision-making processes.
Remember, turning data into decisions is not just a process – it's a journey towards business success.
Ready to dive deeper into the world of Java and data analysis? Check out Java Data Analysis: Leveraging Libraries for Efficient Processing and Oracle's Java Database Connectivity for further insights.
Start your data-driven journey with Java today!
Checkout our other articles