JUnit Test Runners Explained: Boost Your Testing Game!
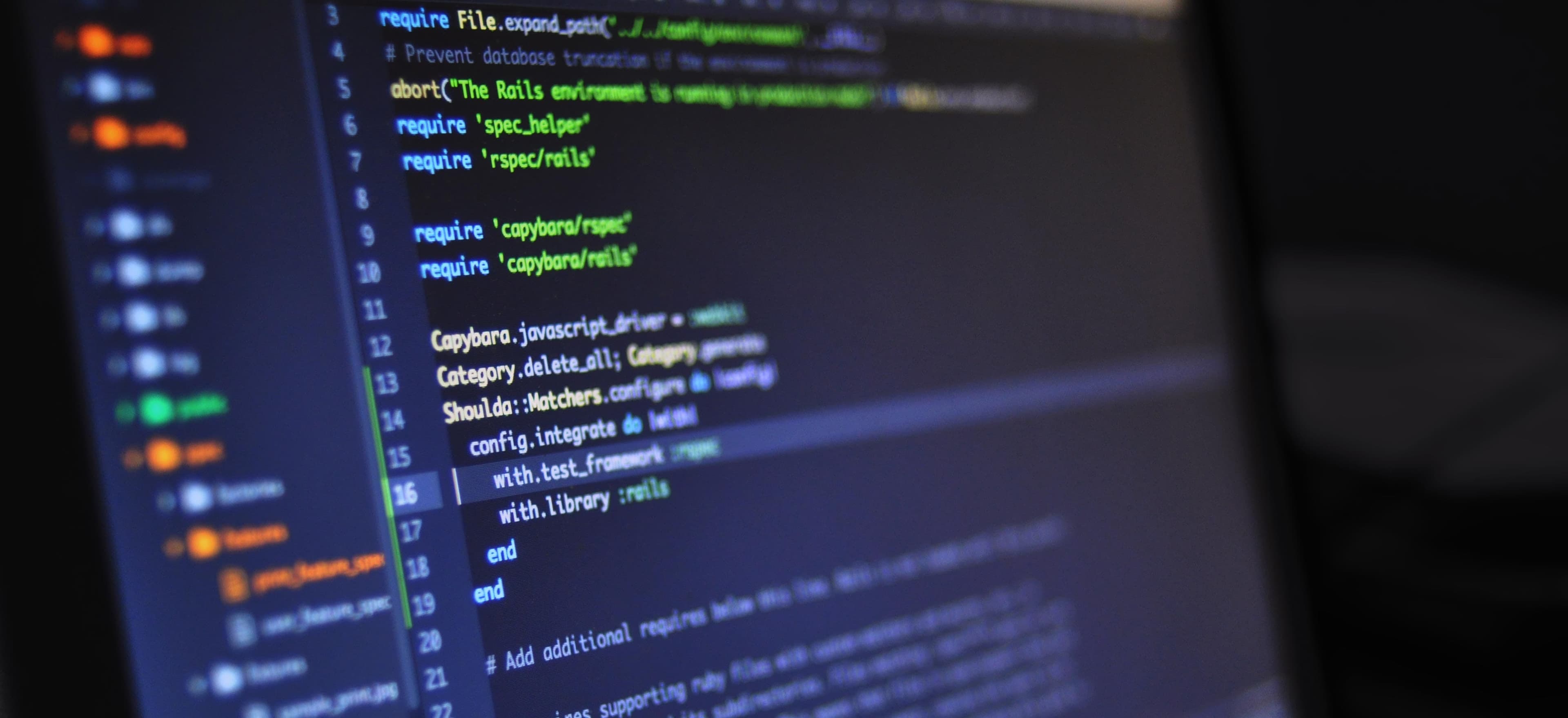
- Published on
JUnit Test Runners Explained: Boost Your Testing Game!
In the world of Java development, testing is an integral part of the software development lifecycle. It ensures that the code behaves as expected, even as changes are made. JUnit, a popular unit testing framework for Java, provides a seamless way to create and run tests, with its test runners playing a pivotal role in executing test cases.
In this article, we'll dive into the concept of JUnit test runners, understand their significance, and explore how they can elevate your testing game.
What are JUnit Test Runners?
In the context of JUnit, a test runner is responsible for executing test cases and reporting the results. When you create tests using JUnit, the test runner facilitates the invocation of these tests and provides feedback on their outcomes.
Understanding the Role of Test Runners
Test runners automate the execution of tests, handle the setup and teardown procedures, and present the test results. They serve as the backbone of the testing process, orchestrating the entire flow and ensuring that tests are executed in a consistent and reliable manner.
Default Test Runner in JUnit 4
In JUnit 4, the default test runner is BlockJUnit4ClassRunner. This runner is responsible for executing test cases annotated with @Test
. It leverages the JUnit framework to run the tests and produce the test results. It supports various annotations, such as @Before
, @After
, @BeforeClass
, and @AfterClass
, which enable the setup and teardown of test fixtures.
Custom Test Runners
While JUnit provides a default test runner, it also allows developers to create custom test runners by extending the ParentRunner class. This capability enables the customization of the test execution process to cater to specific requirements or scenarios.
Let's take a look at an example of a custom test runner that showcases the selective execution of test methods based on a condition.
import org.junit.internal.builders.AllDefaultPossibilitiesBuilder;
import org.junit.runner.Runner;
import org.junit.runners.model.RunnerBuilder;
public class CustomTestRunner extends AllDefaultPossibilitiesBuilder {
@Override
public Runner runnerForClass(Class<?> testClass, RunnerBuilder builder) throws Throwable {
if (System.getProperty("run.all.tests").equals("true")) {
return super.runnerForClass(testClass, builder);
}
// Custom logic for selective test execution
}
}
In this example, the CustomTestRunner
extends AllDefaultPossibilitiesBuilder
and overrides the runnerForClass
method to conditionally execute the test methods based on the value of the run.all.tests
system property. This demonstrates the flexibility offered by custom test runners in tailoring the test execution flow.
Parameterized Test Runners
Another powerful feature of JUnit test runners is the support for parameterized tests. Parameterized tests allow the same test logic to be executed with different sets of parameters, enabling efficient testing of multiple input combinations.
The Parameterized runner in JUnit provides this functionality, allowing developers to annotate test methods with @Parameters
and provide a method to supply the input values.
Let's explore a concise example of a parameterized test runner to illustrate its usage.
@RunWith(Parameterized.class)
public class ParameterizedTest {
@Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][] {
{ 1, true },
{ 2, false },
{ 3, true }
});
}
private int input;
private boolean expectedResult;
public ParameterizedTest(int input, boolean expectedResult) {
this.input = input;
this.expectedResult = expectedResult;
}
@Test
public void testParameterized() {
// Test logic using input and asserting against expectedResult
}
}
In this example, the ParameterizedTest
class is annotated with @RunWith(Parameterized.class)
, indicating that it is a parameterized test. The @Parameters
-annotated method provides the input data, and the constructor of the test class receives the parameters for each test iteration. This showcases how parameterized test runners enable efficient testing across diverse scenarios.
Leveraging Test Runners for Test Suites
In addition to running individual test cases, JUnit test runners can be utilized to execute test suites. A test suite allows the grouping of multiple test classes for cohesive execution and result reporting.
To create a test suite, developers can leverage the Suite runner provided by JUnit. This runner enables the aggregation of test classes and their subsequent execution as a unified suite.
Here's an example of a test suite that includes multiple test classes:
@RunWith(Suite.class)
@Suite.SuiteClasses({TestClass1.class, TestClass2.class, TestClass3.class})
public class TestSuite {
// This class remains empty - used only as a holder for the above annotations
}
In this example, the TestSuite
class is annotated with @RunWith(Suite.class)
and @Suite.SuiteClasses
, specifying the test classes to be included in the suite. When the test suite is executed using the JUnit test runner, all the designated test classes are invoked and their results are aggregated.
The Closing Argument
Understanding and harnessing the power of JUnit test runners is paramount for Java developers striving to build robust, high-quality software. These test runners form the foundation of efficient and effective testing, offering capabilities for customization, parameterization, and suite execution.
By comprehending the role of test runners, developers can enhance their testing strategies, improve test coverage, and streamline the testing process. Embracing the versatility of JUnit test runners can propel the quality and reliability of Java applications, ultimately contributing to a seamless and resilient software ecosystem.
Incorporating JUnit test runners into your testing repertoire can empower you to tackle complex testing scenarios with confidence, and elevate your testing game to new heights!
So, what are you waiting for? Dive into the world of JUnit test runners and unlock the full potential of your testing endeavors!
Remember, robust testing today leads to resilient software tomorrow. Happy testing!
Resources:
Implementing JUnit test runners can significantly enhance the test execution process in Java development. By understanding the significance of test runners, developers can optimize their testing strategies for improved software reliability. Whether for individual test cases, parameterized tests, or test suites, JUnit test runners provide the necessary flexibility and customization to elevate the testing game.