Troubleshooting Mixed Messages in Memory Unit Systems
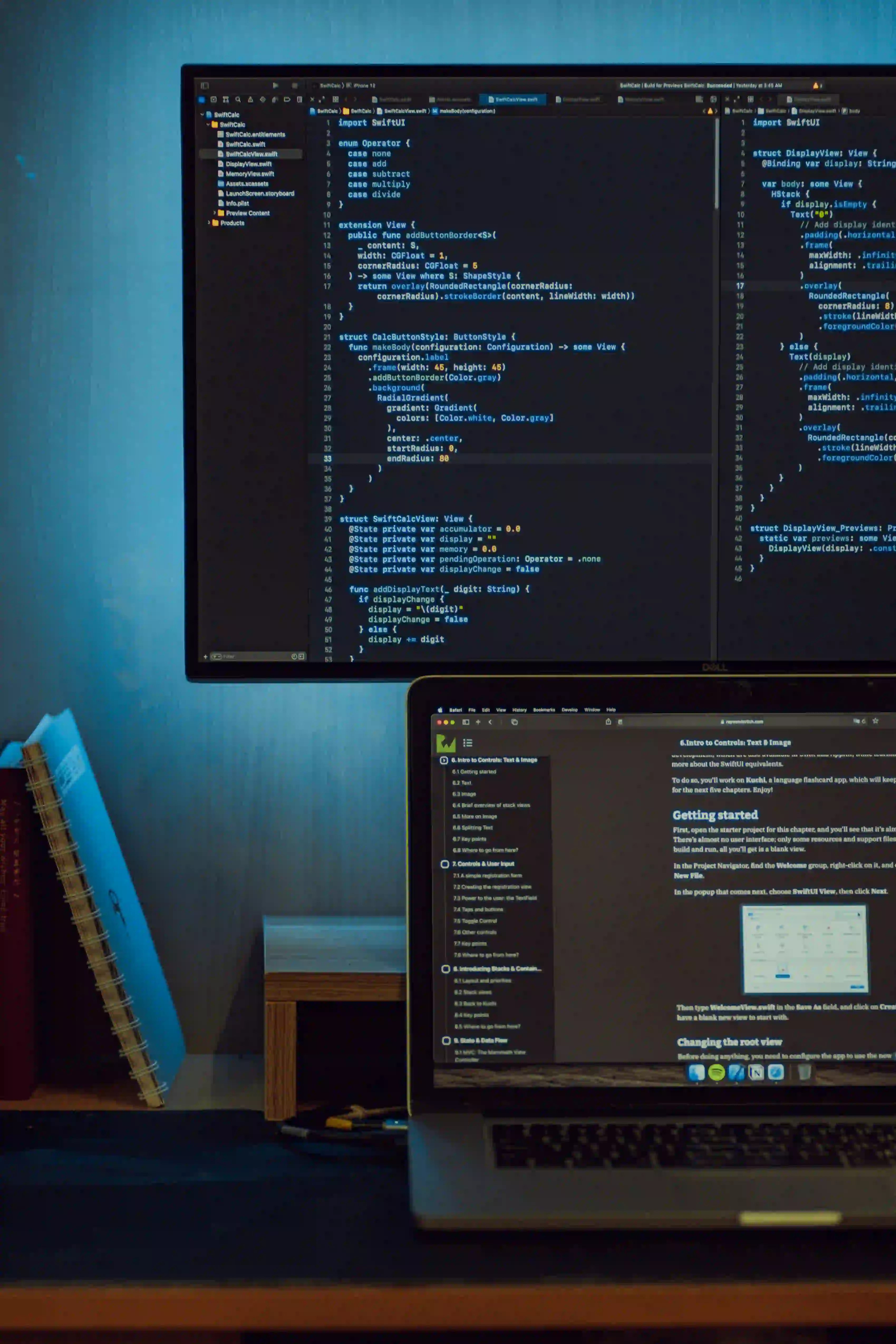
Troubleshooting Mixed Messages in Memory Unit Systems
In the complex realm of software engineering, especially in Java programming, developers often encounter memory-related issues. One common problem that arises is the presence of mixed messages in memory unit systems. These mixed messages can lead to unpredictable application behavior and performance degradation. In this blog post, we will delve into the nature of mixed messages in memory units, identify their causes, and explore troubleshooting techniques.
Understanding Memory Unit Systems
Before we dive into mixed messages, let's first understand what memory unit systems are. In Java, memory management is primarily handled by the Java Virtual Machine (JVM), and it consists of several components, including:
- Heap Memory: Where objects are stored.
- Stack Memory: Where method references and locals are stored.
- Method Area: Holds class definitions and metadata.
Understanding these fundamentals is critical as they form the backdrop against which mixed messages occur.
Causes of Mixed Messages
Mixed messages in memory units usually arise from:
- Concurrency Issues: When multiple threads access shared memory without appropriate synchronization, leading to inconsistent states.
- Garbage Collection Delays: Long pauses in garbage collection can cause the application to misinterpret the state of memory, resulting in erroneous messages.
- Buffer Overflows: This occurs when more data is written to a buffer than it can hold, corrupting nearby memory.
- Improper Memory Allocation: Poorly managed memory allocation can lead to leaks and fragmented memory, causing unexpected behavior.
Diagnosing Mixed Messages
Step 1: Enable Verbose Garbage Collection
Garbage collection can play a pivotal role in memory-related issues. By enabling verbose garbage collection logs, you can obtain a clearer picture of how often garbage collection occurs and how long it takes.
To enable verbose garbage collection, run your Java application with the following commands:
java -verbose:gc -XX:+PrintGCDetails -XX:+PrintGCTimeStamps -jar YourApp.jar
Step 2: Utilize Profiling Tools
Profiling tools like VisualVM or JProfiler can help identify memory leaks and allocation patterns. These tools provide a visual representation of memory consumption and help you analyze the lifecycle of Java objects.
Here’s a simple way to use VisualVM:
- Install VisualVM.
- Start your Java application.
- Open VisualVM, and connect to your application.
- Navigate to the “Monitor” tab to observe memory usage in real-time.
Step 3: Analyze Thread Dumps
When you start to notice mixed messages, generating a thread dump can provide insights into what your threads are doing. Use the following command to generate a thread dump:
jstack <PID>
In the output, look for threads that are in the BLOCKED or WAITING states, as they can be indicators of concurrency issues.
Example: Debugging a Concurrency Issue
Let's consider a simple example where multiple threads attempt to modify a shared list. This situation can lead to mixed messages if proper synchronization is not implemented.
Code Snippet: Unsynchronized Access
import java.util.ArrayList;
import java.util.List;
public class MixedMessagesExample {
private static List<String> sharedList = new ArrayList<>();
public static void main(String[] args) {
Runnable task = () -> {
for (int i = 0; i < 5; i++) {
// Unsynchronized access to shared list
sharedList.add(Thread.currentThread().getName() + " added value " + i);
}
};
Thread thread1 = new Thread(task);
Thread thread2 = new Thread(task);
thread1.start();
thread2.start();
}
}
Why This Can Cause Mixed Messages
In the above code, multiple threads concurrently attempt to modify sharedList
. Since there's no synchronization, this can lead to unexpected behavior, resulting in mixed messages when accessing the list's contents.
Solution: Implementing Synchronization
We can fix this using synchronized methods or blocks:
import java.util.ArrayList;
import java.util.List;
public class OrderedMessagesExample {
private static List<String> sharedList = new ArrayList<>();
public static synchronized void addToList(String value) {
sharedList.add(value);
}
public static void main(String[] args) {
Runnable task = () -> {
for (int i = 0; i < 5; i++) {
addToList(Thread.currentThread().getName() + " added value " + i);
}
};
Thread thread1 = new Thread(task);
Thread thread2 = new Thread(task);
thread1.start();
thread2.start();
}
}
Explanation
In this synchronized version, the addToList
method ensures that only one thread can execute it at a time. This prevents mixed messages arising from concurrent modifications, thereby maintaining data integrity.
Advanced Troubleshooting Techniques
Memory Analysis Tools
In addition to using profiling tools, you can utilize memory analysis tools like Eclipse MAT (Memory Analyzer Tool) to detect memory leaks and analyze heap dumps. Following is how to analyze a heap dump:
-
Generate a heap dump when your application is experiencing issues using:
🔧snippet.shjmap -dump:live,format=b,file=heapdump.hprof <PID>
-
Open the generated
heapdump.hprof
in Eclipse MAT. -
Use the tool to find objects that are occupying significant memory.
Review Your Codebase
Reviewing your codebase systematically can often reveal hidden issues. Focus on:
- Reviewing thread management techniques.
- Ensuring good coding practices around collections and data structures.
- Investigating the use of external libraries that might not handle memory properly.
Closing Thoughts
Mixed messages in memory unit systems can severely impact application performance. By understanding the underlying causes and employing effective troubleshooting techniques, developers can pinpoint and resolve these issues seamlessly. Remember to leverage tools like VisualVM, invoke garbage collection logs, and practice good coding standards to keep your Java applications running cleanly in a well-managed memory environment.
By following these guidelines, not only will your applications perform better, but your coding skills will also improve. For more on Java memory management, check out the Java Official Documentation. Happy coding!