Mastering Java: Solving Common Multithreading Issues
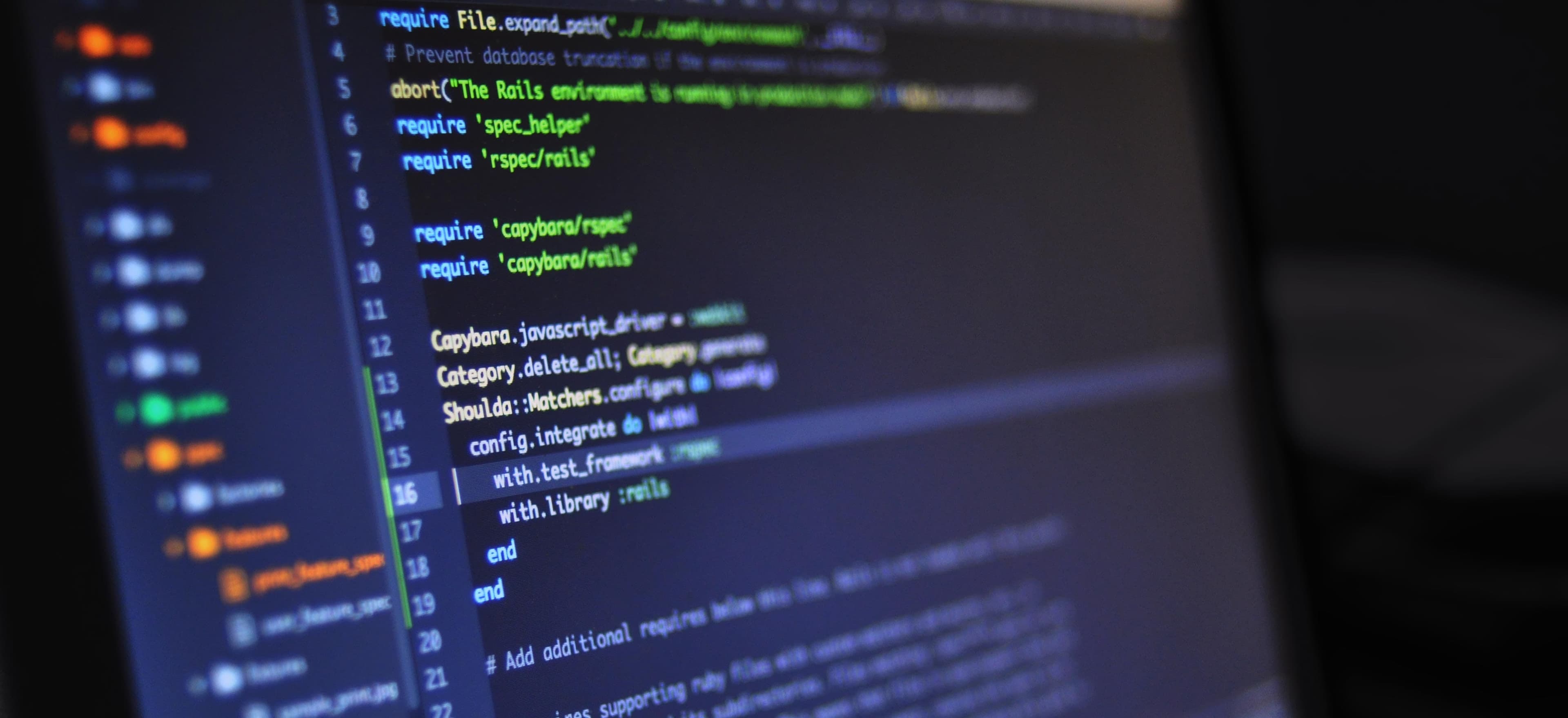
- Published on
Mastering Java: Solving Common Multithreading Issues
Java is a powerful and versatile language, known for its ability to handle complex tasks and accommodate high-performance requirements. Multithreading is one of the key features of Java, allowing developers to execute multiple threads simultaneously and improve application performance. However, multithreading in Java also comes with its fair share of challenges and common issues that developers often encounter. In this article, we will explore some of the most common multithreading issues in Java and how to solve them effectively.
Understanding Multithreading in Java
Before diving into the common issues and their solutions, it's important to have a clear understanding of multithreading in Java. In essence, multithreading allows multiple parts of a program to run concurrently, making good use of available resources and improving overall efficiency. However, managing multiple threads can lead to various complications if not handled properly.
Common Multithreading Issues and Their Solutions
1. Race Conditions
A race condition occurs when multiple threads access and modify shared data concurrently, leading to unpredictable behavior and potential data corruption. To mitigate race conditions, the synchronized
keyword can be used to create a critical section, allowing only one thread to execute the synchronized block at a time.
public class Counter {
private int count;
public synchronized void increment() {
count++;
}
}
Using the synchronized
keyword ensures that only one thread can execute the increment
method at a time, preventing race conditions and maintaining data integrity.
2. Deadlocks
Deadlocks occur when two or more threads are blocked forever, waiting for each other to release the resources they need. To prevent deadlocks, it's essential to follow a strict ordering when acquiring locks and avoid holding a lock while making resource requests.
public class DeadlockExample {
private final Object lock1 = new Object();
private final Object lock2 = new Object();
public void method1() {
synchronized (lock1) {
synchronized (lock2) {
// Code here
}
}
}
public void method2() {
synchronized (lock2) {
synchronized (lock1) {
// Code here
}
}
}
}
In the above example, both method1
and method2
follow a strict ordering when acquiring locks, reducing the risk of deadlock.
3. Thread Starvation
Thread starvation occurs when a thread is unable to gain regular access to shared resources and is unable to make progress. To address thread starvation, the use of fairness policies and avoiding long-running tasks in critical sections can help distribute resources more equitably among threads.
4. Visibility Issues
In a multithreaded environment, changes made by one thread may not be immediately visible to other threads due to caching and memory model issues. Using the volatile
keyword ensures that changes to the variable are immediately visible to other threads.
public class SharedData {
private volatile boolean stop = false;
public void stopThread() {
stop = true;
}
public void run() {
while (!stop) {
// Execute task
}
}
}
By declaring the stop
variable as volatile
, any changes made to it will be immediately visible to other threads, resolving visibility issues.
5. Thread Safety
Ensuring thread safety is crucial when working with shared resources in a multithreaded environment. Utilizing concurrent data structures such as ConcurrentHashMap
and ConcurrentLinkedQueue
as well as using proper synchronization techniques can help maintain thread safety and prevent data corruption.
Best Practices for Multithreading in Java
In addition to addressing common multithreading issues, following best practices can further enhance the effectiveness of multithreaded applications in Java.
1. Use Executors and Thread Pools
Using executors and thread pools simplifies the management of threads, allowing for better resource utilization and improved performance. The ExecutorService
interface provides a high-level API for executing tasks asynchronously.
ExecutorService executor = Executors.newFixedThreadPool(5);
executor.submit(() -> {
// Task execution
});
2. Immutable Objects
Using immutable objects eliminates the need for synchronization, as immutable objects cannot be modified once created. This reduces the complexity of dealing with shared data and ensures thread safety.
3. Java Memory Model
Understanding the Java Memory Model is crucial for writing effective multithreaded code. It defines the rules for reading and writing shared variables and ensures proper synchronization.
4. Avoid Thread.sleep()
Using Thread.sleep()
for synchronization or timing control is generally discouraged, as it can lead to unpredictable behavior and hinder performance. Instead, consider using higher-level concurrency utilities provided by the java.util.concurrent
package.
To Wrap Things Up
Mastering multithreading in Java involves understanding the common issues that may arise and applying effective solutions to mitigate them. By addressing race conditions, deadlocks, thread starvation, visibility issues, and ensuring thread safety, developers can create robust and efficient multithreaded applications. Additionally, following best practices such as using executors, working with immutable objects, understanding the Java Memory Model, and avoiding Thread.sleep()
contributes to the overall effectiveness of multithreading in Java.
Incorporating these strategies and best practices not only resolves common multithreading issues but also enhances the performance and reliability of multithreaded applications, ultimately mastering the art of multithreading in Java.
Remember, mastering multithreading in Java is a continuous learning process, and staying updated with the latest advancements and practices is key to excelling in this domain.
For further exploration and deeper understanding of multithreading in Java, consider exploring the official Java Concurrency in Practice book and the Java Documentation on Concurrency provided by Oracle. These resources offer invaluable insights and practical knowledge for mastering multithreading in Java.
Mastering multithreading in Java unlocks the potential to build highly scalable, efficient, and responsive applications, making it a crucial skill for Java developers.
Checkout our other articles