Avoid Crashes: Smart Ways to Remove from ArrayList
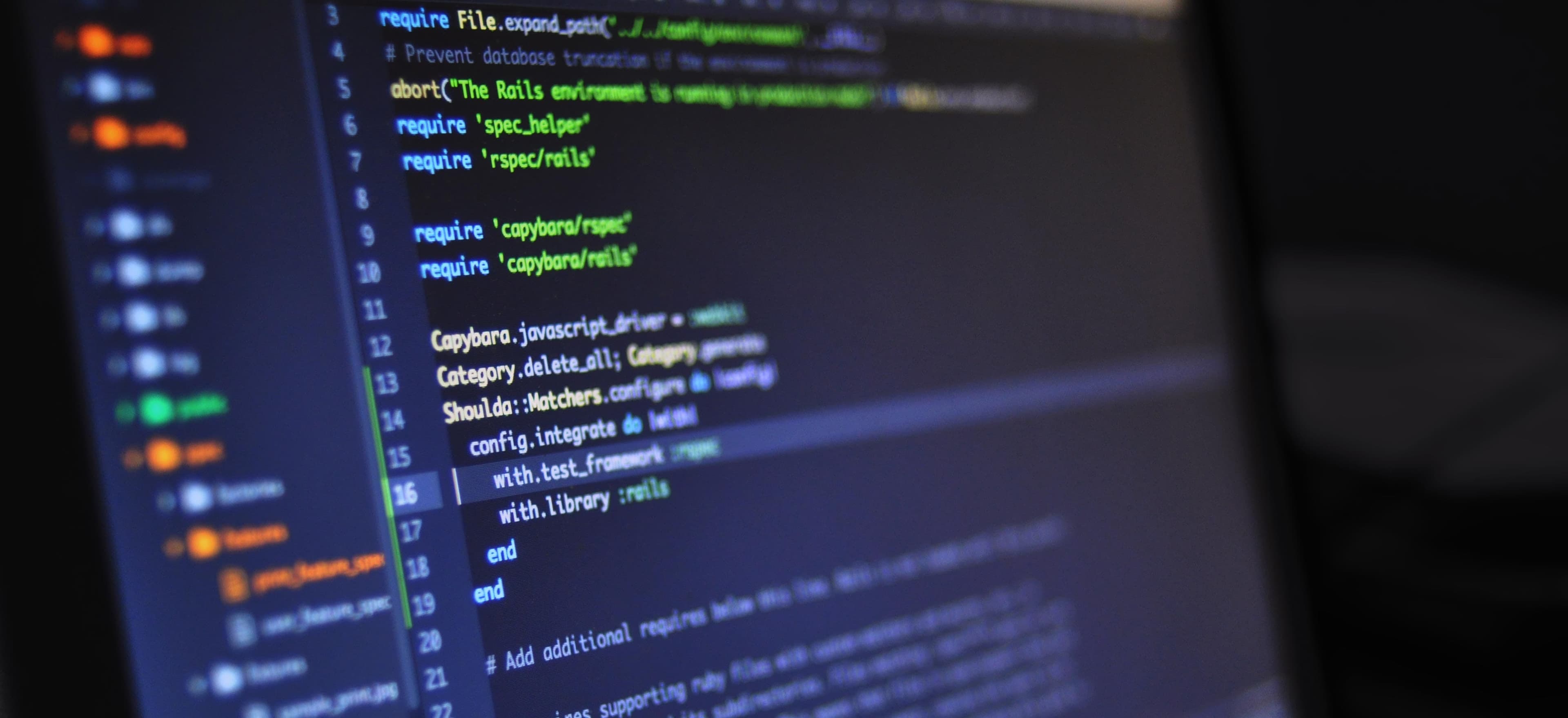
- Published on
How to Safely Remove Items from ArrayList in Java
When working with Java, you might find yourself working with ArrayLists, and at some point, you will need to remove items from them. However, removing items from an ArrayList in Java requires caution to avoid unexpected crashes. In this post, we'll delve into some smart and safe ways to remove items from an ArrayList in Java to help you avoid common pitfalls and ensure your code runs smoothly.
The Basics of ArrayList in Java
Before we dive into removal techniques, let's quickly recap what an ArrayList is and how it works in Java. An ArrayList is a dynamic array that can grow or shrink in size at runtime. It's part of the java.util
package and provides a more flexible way of working with arrays compared to the traditional array in Java.
Removing Items from an ArrayList: The Common Pitfalls
When it comes to removing items from an ArrayList, there are several common mistakes that can lead to crashes or unexpected behavior in your code. Some of these pitfalls include:
- Modifying the ArrayList while iterating over it
- Using the wrong index to remove an item
- Failing to handle concurrent modifications
Now, let's explore some safe and efficient ways to remove items from an ArrayList in Java.
Method 1: Using Iterator to Safely Remove Items
One of the safest ways to remove items from an ArrayList in Java is by using an Iterator. By using an Iterator, you can avoid concurrent modification exceptions and safely remove items from the ArrayList while iterating over it.
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
Iterator<String> iterator = names.iterator();
while (iterator.hasNext()) {
String name = iterator.next();
if (name.equals("Bob")) {
iterator.remove();
}
}
In the example above, we use an Iterator to iterate over the names
ArrayList and remove the item "Bob" safely without causing any concurrent modification exceptions.
Why use Iterator?
Using an Iterator is a safe way to remove items from an ArrayList because it allows you to remove items while iterating over the list without causing concurrent modification exceptions. The Iterator provides a way to modify the collection safely during iteration, ensuring that the underlying collection is modified as expected.
Method 2: Using Enhanced for-loop to Remove Items
Another approach to safely remove items from an ArrayList is by using an enhanced for-loop. While this method is not as safe as using an Iterator, it can still be used effectively for simple cases where concurrent modifications are not a concern.
List<Integer> numbers = new ArrayList<>();
numbers.add(10);
numbers.add(20);
numbers.add(30);
for (Integer number : new ArrayList<>(numbers)) {
if (number == 20) {
numbers.remove(number);
}
}
In the example above, we use an enhanced for-loop to iterate over a copy of the numbers
ArrayList and remove the item "20" from the original list. By iterating over a copy of the list, we avoid concurrent modification exceptions.
Why use Enhanced for-loop?
While using an enhanced for-loop may not be as safe as using an Iterator, it can still be effective in certain scenarios, especially when concurrent modifications are not a concern. It provides a more concise and readable way to iterate over the elements of the ArrayList.
Method 3: Using removeAll() to Remove Multiple Items
If you need to remove multiple items from an ArrayList based on certain criteria, the removeAll()
method can be a powerful tool. This method allows you to remove all elements from an ArrayList that are contained in another collection, effectively removing multiple items in a single operation.
List<String> colors = new ArrayList<>();
colors.add("red");
colors.add("blue");
colors.add("green");
colors.add("yellow");
List<String> toBeRemoved = Arrays.asList("blue", "green");
colors.removeAll(toBeRemoved);
In this example, we use the removeAll()
method to remove the elements "blue" and "green" from the colors
ArrayList based on the contents of the toBeRemoved
list.
Why use removeAll()?
The removeAll()
method provides a convenient and efficient way to remove multiple items from an ArrayList in a single operation. It simplifies the process of removing elements based on specific criteria, improving code readability and maintainability.
My Closing Thoughts on the Matter
Removing items from an ArrayList in Java requires careful consideration to avoid crashes and unexpected behavior. By utilizing the techniques discussed in this post, such as using Iterators, enhanced for-loops, and the removeAll()
method, you can safely and efficiently remove items from an ArrayList while minimizing the risk of errors and concurrency issues.
By understanding the safe removal techniques and when to use them, you can write more robust and reliable code when working with ArrayLists in Java.
Remember, it's always better to be cautious when modifying collections to ensure the stability and correctness of your code.
So, go ahead and apply these smart ways to remove items from ArrayLists in your Java projects to keep your code running smoothly!
If you want to dig deeper into ArrayLists and other Java collections, you can check out the Java Collections Framework documentation for a comprehensive understanding.