Cloneable in Java: Solving Deep vs Shallow Copy Dilemma
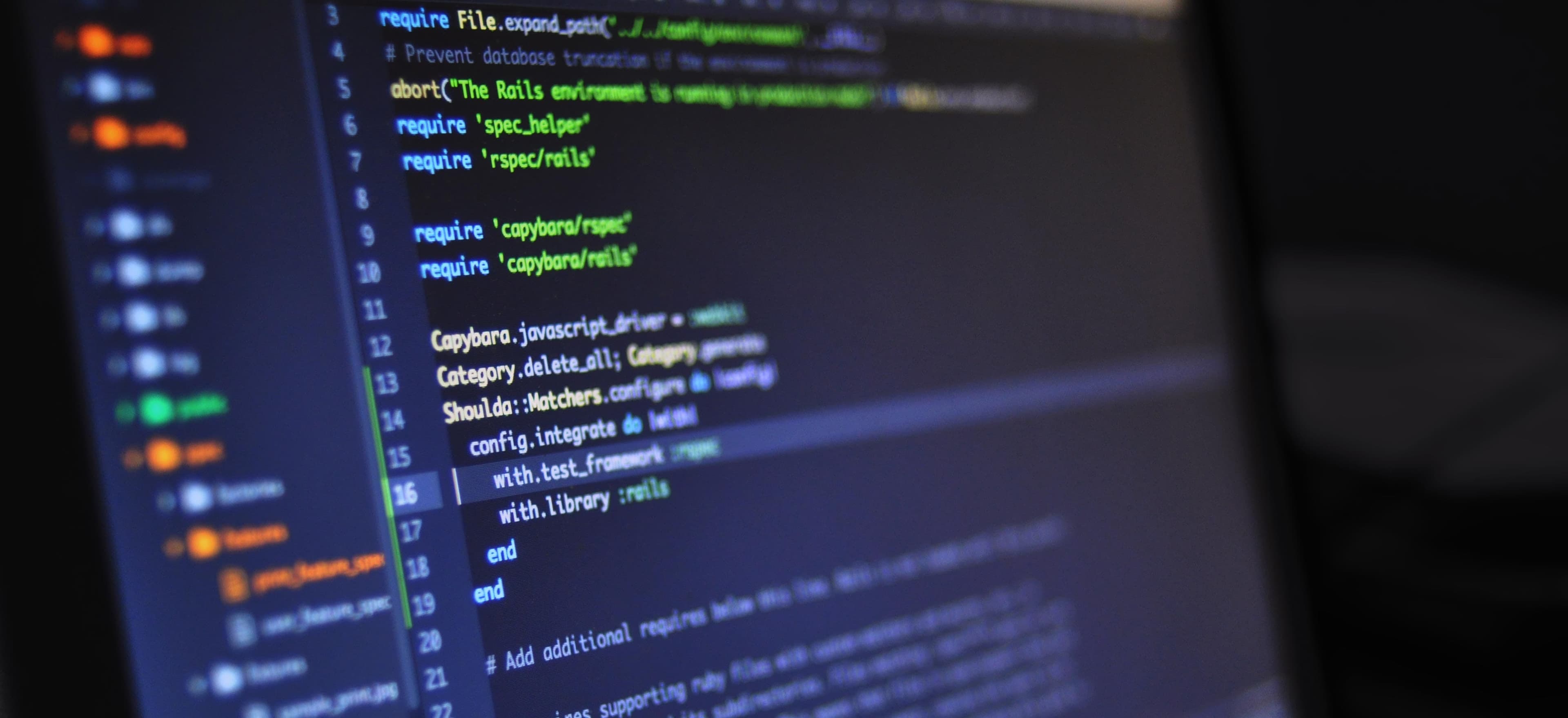
- Published on
Cloneable in Java: Solving Deep vs Shallow Copy Dilemma
In Java, cloning an object can be a tricky task, especially when it comes to making deep or shallow copies. The Cloneable
interface in Java provides a way to manage this by controlling the behavior of the clone()
method. In this article, we'll explore the challenges of copying objects in Java, understand the concept of deep and shallow copies, and learn how to use Cloneable
to address this dilemma.
Understanding Deep and Shallow Copies
Before delving into the Cloneable
interface, it's crucial to grasp the distinction between deep and shallow copies.
-
Shallow Copy: It involves copying the object along with its references, but not the referenced objects themselves. As a result, changes to the copied object's attributes can affect the original object and vice versa.
-
Deep Copy: It involves copying the object as well as all the objects referenced by it, creating a completely independent clone. This ensures that changes to the copied object do not impact the original object or its referenced objects.
The Quandary of Object Cloning in Java
Java provides the clone()
method for making copies of objects. However, the default behavior of clone()
is to perform a shallow copy, which can lead to unexpected side effects. Consider the following example:
class Person implements Cloneable {
private String name;
public Person(String name) {
this.name = name;
}
public String getName() {
return name;
}
@Override
public Object clone() throws CloneNotSupportedException {
return super.clone();
}
}
public class Main {
public static void main(String[] args) {
Person person1 = new Person("Alice");
try {
Person person2 = (Person) person1.clone();
person2.setName("Bob"); // Changes name of person2
System.out.println(person1.getName()); // Outputs "Bob" instead of "Alice" due to shallow copy
} catch (CloneNotSupportedException e) {
e.printStackTrace();
}
}
}
In the above example, person2
is intended to be a clone of person1
. However, due to the shallow copy behavior, modifying person2
affects person1
. This is where the Cloneable
interface comes into play.
Leveraging Cloneable Interface for Deep Copy
To achieve a deep copy using the Cloneable
interface, the clone()
method needs to be overridden to create a deep copy of the object and its referenced objects. Let's enhance the Person
class to support deep copy using the Cloneable
interface:
class Person implements Cloneable {
private String name;
public Person(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public Object clone() throws CloneNotSupportedException {
Person clonedPerson = (Person) super.clone();
return clonedPerson;
}
}
With this updated implementation, let's revisit the previous example:
public class Main {
public static void main(String[] args) {
Person person1 = new Person("Alice");
try {
Person person2 = (Person) person1.clone();
person2.setName("Bob");
System.out.println(person1.getName()); // Outputs "Alice" as expected
} catch (CloneNotSupportedException e) {
e.printStackTrace();
}
}
}
By implementing Cloneable
and overriding the clone()
method, the Person
class now creates a deep copy when cloned, effectively resolving the dilemma of object cloning in Java.
Closing Remarks
In Java, the Cloneable
interface serves as a powerful tool for handling object cloning, enabling developers to create deep copies with ease. By understanding the difference between deep and shallow copies, and harnessing the capabilities of Cloneable
, developers can ensure the integrity of cloned objects and avoid unexpected side effects.
In summary, when faced with the deep vs shallow copy dilemma in Java, remember to leverage the Cloneable
interface and override the clone()
method to enable deep copying, safeguarding the independence of cloned objects.
For further information on object cloning and the Cloneable
interface in Java, you can refer to the official Java documentation.
Happy coding!