Cracking the Code: OpenSSL to Java RSA Decryption Dilemma
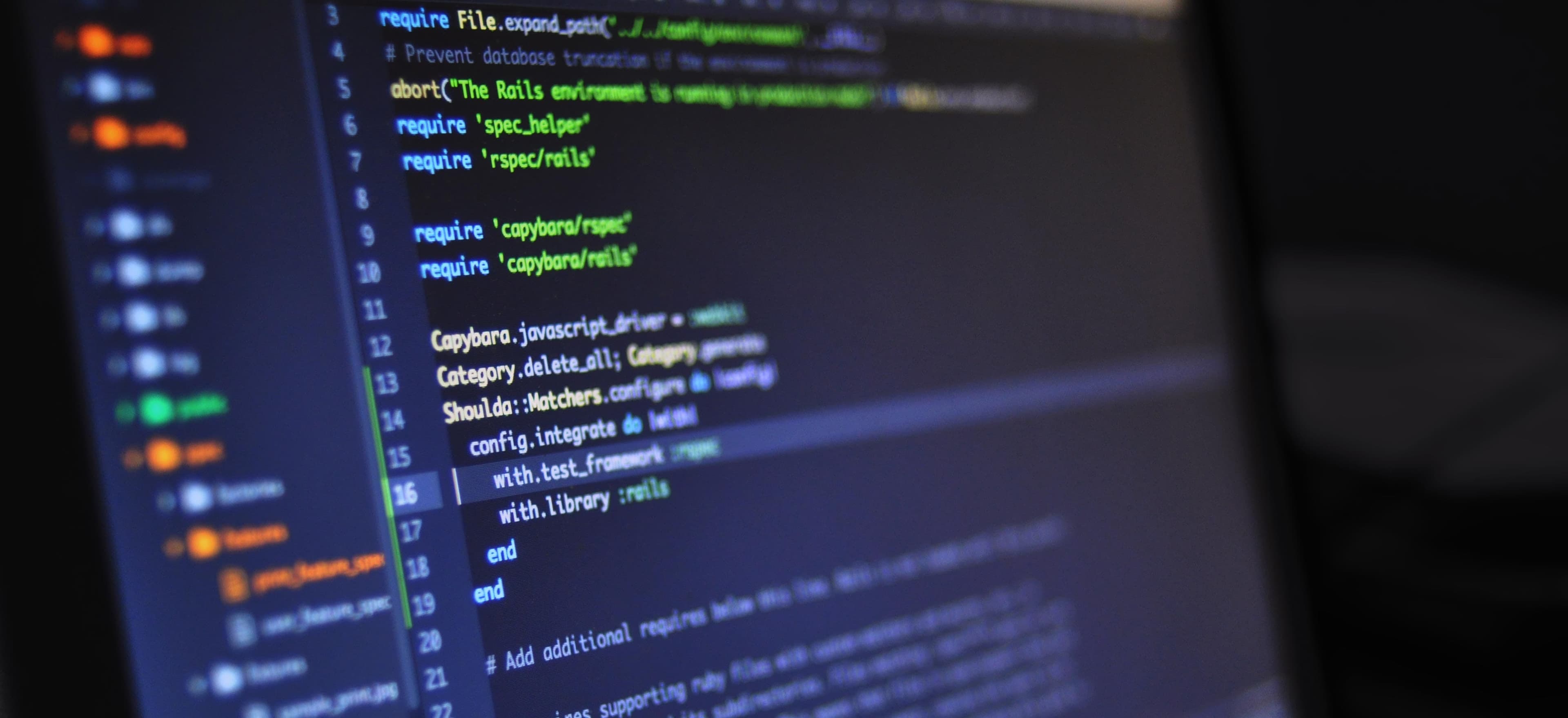
- Published on
Cracking the Code: OpenSSL to Java RSA Decryption Dilemma
If you have ever found yourself dealing with encryption and decryption across multiple platforms, you may have encountered the challenge of interoperability between OpenSSL and Java when working with RSA encryption. In this post, we will explore the intricacies of RSA encryption in OpenSSL and Java, and uncover the potential hurdles you might face when decrypting data encrypted with OpenSSL using Java.
Understanding RSA Encryption
RSA (Rivest-Shamir-Adleman) is a widely used public-key cryptosystem for secure data transmission. It involves the use of a public key to encrypt the data and a private key to decrypt the data. RSA encryption often requires interoperability between different languages and platforms, which can lead to compatibility issues.
OpenSSL and Java RSA Encryption
OpenSSL is a robust, open-source toolkit for implementing SSL/TLS protocols and includes a cryptography library that provides an open-source implementation of the SSL and TLS protocols. Java, on the other hand, has its built-in security package, providing various cryptographic functionalities through the java.security
package.
When it comes to RSA encryption, OpenSSL and Java use different padding schemes and key formats, which can cause decryption issues when data encrypted with OpenSSL needs to be decrypted in Java.
The Challenge: OpenSSL's Default Padding Scheme
OpenSSL, by default, uses the PKCS#1 v1.5 padding scheme for RSA encryption. This scheme, while widely used, has known vulnerabilities. In contrast, Java uses the more secure OAEP (Optimal Asymmetric Encryption Padding) scheme by default.
When decrypting data in Java that has been encrypted with OpenSSL using PKCS#1 v1.5 padding, compatibility issues may arise due to the padding scheme mismatch.
Overcoming the Dilemma: Java Code for RSA Decryption
To effectively decrypt data encrypted with OpenSSL using Java, you need to apply the PKCS#1 v1.5 padding during decryption. Let's take a look at a Java code snippet that demonstrates how to achieve this:
import java.security.*;
import java.security.spec.*;
import javax.crypto.*;
import javax.crypto.spec.*;
import java.util.Base64;
public class RSADecryptor {
public static byte[] decrypt(byte[] encryptedData, PrivateKey privateKey) throws Exception {
Cipher cipher = Cipher.getInstance("RSA/ECB/PKCS1Padding");
cipher.init(Cipher.DECRYPT_MODE, privateKey);
return cipher.doFinal(encryptedData);
}
public static void main(String[] args) throws Exception {
String encryptedData = "lMoLf6oqf0vQRY/+zETaX4g=="; // Base64 encoded encrypted data
byte[] encryptedBytes = Base64.getDecoder().decode(encryptedData);
KeyFactory keyFactory = KeyFactory.getInstance("RSA");
// Replace privateKeyBytes with the actual private key bytes
byte[] privateKeyBytes = /* Your private key bytes */;
PKCS8EncodedKeySpec keySpec = new PKCS8EncodedKeySpec(privateKeyBytes);
PrivateKey privateKey = keyFactory.generatePrivate(keySpec);
byte[] decryptedData = decrypt(encryptedBytes, privateKey);
System.out.println("Decrypted Data: " + new String(decryptedData));
}
}
In the above Java code, we define a RSADecryptor
class with a decrypt
method that decrypts the provided encrypted data using the private key with PKCS#1 v1.5 padding. The main
method demonstrates how to use the RSADecryptor
class to decrypt the encrypted data.
By utilizing the Cipher.getInstance("RSA/ECB/PKCS1Padding")
method, we explicitly specify the PKCS#1 v1.5 padding scheme for RSA decryption, ensuring compatibility with data encrypted using OpenSSL.
Lessons Learned
In conclusion, interoperability between OpenSSL and Java for RSA encryption and decryption can be challenging due to differences in padding schemes and key formats. To overcome this dilemma, it is crucial to use the appropriate padding scheme during decryption in Java to match the encryption performed in OpenSSL.
Understanding the nuances of RSA encryption in different platforms and employing the correct cryptographic schemes is essential for seamless interoperability and secure data transmission across diverse systems.
By applying the insights shared in this post and utilizing the provided Java code snippet, you can effectively tackle the OpenSSL to Java RSA decryption dilemma and ensure the successful interoperability of RSA encryption across different platforms.
To dive deeper into RSA encryption, OpenSSL, and Java security, explore the following resources:
Mastering the intricacies of RSA encryption interoperability empowers you to navigate the complexities of secure data transmission with confidence and precision. Happy coding!