Mastering Java EE 8 MVC: Fixing Form Parameter Issues
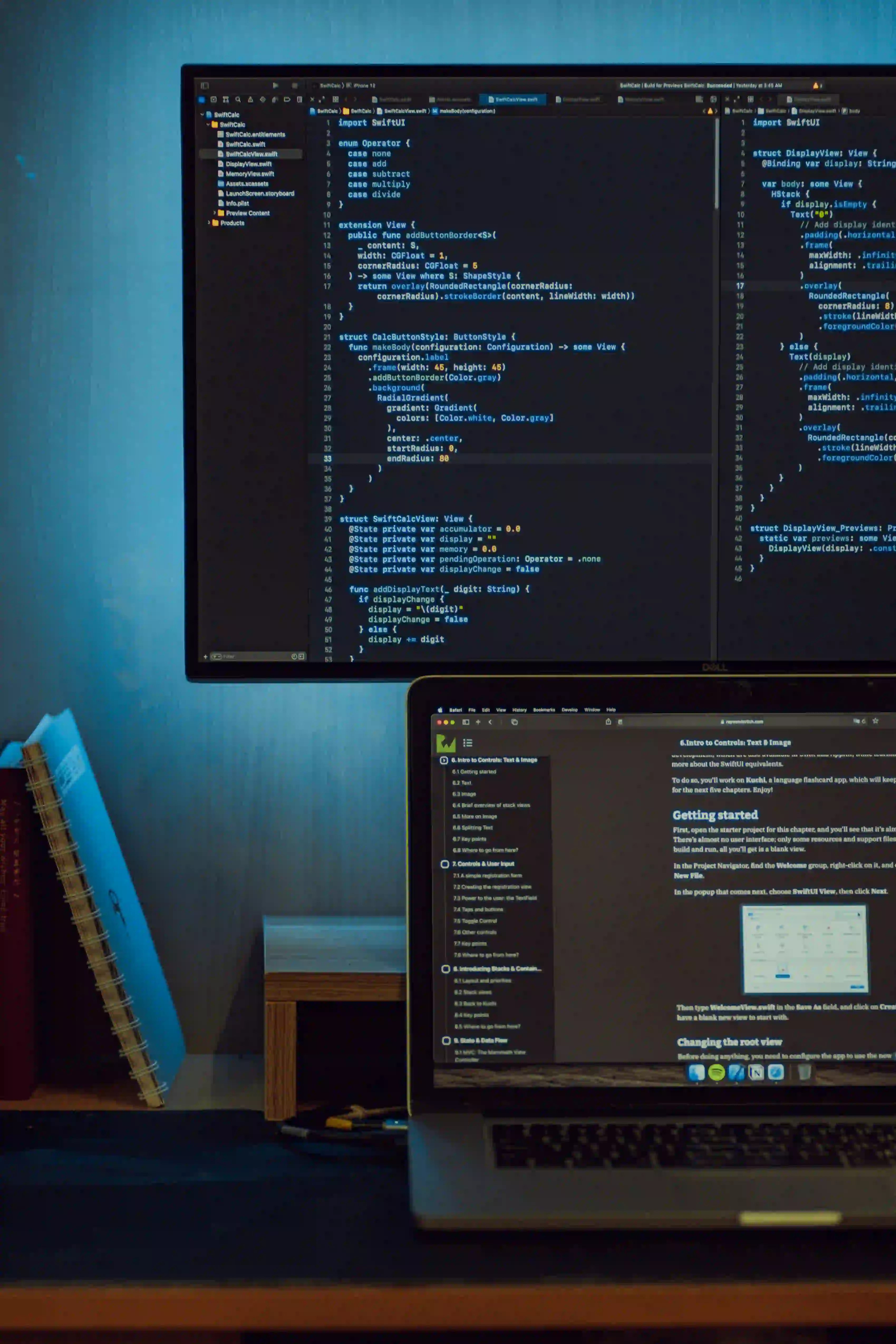
Mastering Java EE 8 MVC: Fixing Form Parameter Issues
In Java EE development, managing form parameters is essential for building robust and interactive web applications. The Model-View-Controller (MVC) architecture provides a structured approach to handling user input and updating the underlying data model. However, handling form parameters in Java EE 8 MVC can sometimes lead to unexpected issues, especially when dealing with complex forms or custom data types.
In this blog post, we will explore common form parameter issues in Java EE 8 MVC and demonstrate effective solutions to address them. By diving into practical examples and code snippets, you will gain insights into best practices for form parameter handling in Java EE 8 MVC.
Understanding Form Parameter Issues
When working with forms in web applications, several challenges may arise, including:
- Handling Complex Form Data: Processing forms with nested structures or custom data types requires careful parameter mapping and validation.
- Dealing with Large Form Submissions: Managing large amounts of form data and preventing performance bottlenecks during submission and processing.
- Validating and Transforming Input: Ensuring that form parameters meet specific validation criteria and transforming them into appropriate data types for storage and processing.
Java EE 8 MVC offers robust features to address these challenges, but developers must be adept at leveraging these capabilities effectively.
Addressing Form Parameter Issues
Let's delve into the practical solutions for addressing form parameter issues in Java EE 8 MVC.
Handling Complex Form Data with @BeanParam
One common issue developers encounter is mapping complex form data to Java objects. Consider a scenario where a form comprises multiple input fields, each corresponding to nested properties of a domain object. In such cases, manually mapping each form parameter to the corresponding object properties can be cumbersome and error-prone.
To simplify this process, Java EE 8 introduces the @BeanParam
annotation, which allows consolidating form parameters into a dedicated Java bean. This approach streamlines the mapping of form data to complex object structures, enhancing code readability and maintainability.
@Path("/user")
public class UserController {
@POST
@Path("/update")
public Response updateUser(@BeanParam UserForm userForm) {
// Process userForm and update user details
return Response.ok("User details updated successfully").build();
}
}
In this example, the UserForm
class aggregates form parameters using corresponding properties, reducing the need for manual parameter mapping.
Dealing with Large Form Submissions Using @FormParam
When handling large form submissions, it's essential to optimize data transfer and processing to minimize performance overhead. Java EE 8 provides the @FormParam
annotation for efficiently extracting individual form parameters from the request body, particularly useful for large form data.
@POST
@Path("/process")
public Response processForm(
@FormParam("username") String username,
@FormParam("email") String email,
@FormParam("message") String message
) {
// Process individual form parameters
return Response.ok("Form submitted successfully").build();
}
By using @FormParam
, developers can extract specific form parameters directly, circumventing unnecessary parsing of the entire request body.
Validating and Transforming Input with Bean Validation and Conversion Providers
Form data validation and conversion are critical aspects of ensuring data integrity and consistency. Java EE 8 provides robust mechanisms for enforcing validation constraints and automatically converting form parameters to the desired data types.
public class RegistrationForm {
@NotNull
@Size(min = 3, max = 50)
private String username;
@Email
private String email;
// Other form fields and validation annotations
}
By applying Bean Validation annotations such as @NotNull
, @Size
, and @Email
to form bean properties, developers can enforce specific validation rules, ensuring that the submitted form data meets predefined constraints.
Additionally, Java EE 8 offers conversion providers to facilitate automatic transformation of form parameters to target data types, such as converting strings to numeric values or custom object representations.
@Provider
public class CustomDateConverter implements ParamConverter<Date> {
@Override
public Date fromString(String value) {
// Custom logic to convert form parameter to Date object
}
@Override
public String toString(Date value) {
// Custom logic to convert Date object to string representation
}
}
By implementing a custom ParamConverter
, developers can define tailored conversion logic for specific data types, ensuring seamless transformation of form parameters.
Final Considerations
Mastering form parameter handling is fundamental for building robust and user-friendly web applications in Java EE 8 MVC. By effectively addressing form parameter issues using @BeanParam
, @FormParam
, Bean Validation, and Conversion Providers, developers can streamline form processing, enhance data integrity, and improve overall user experience.
In summary, Java EE 8 offers a rich set of features and best practices for handling form parameters, empowering developers to build efficient and resilient web applications.
For further exploration, check out the official Java EE 8 documentation, which provides comprehensive insights into form parameter handling and other key aspects of Java EE development.
Start harnessing the power of Java EE 8 MVC for seamless form parameter management and elevate your web application development to the next level! Happy coding!
Remember, understanding the underlying mechanisms and best practices for addressing form parameter issues in Java EE 8 MVC is key to building robust and efficient web applications.