Boost Your Java 9 Apps: Mastering HTTP/2 Support!
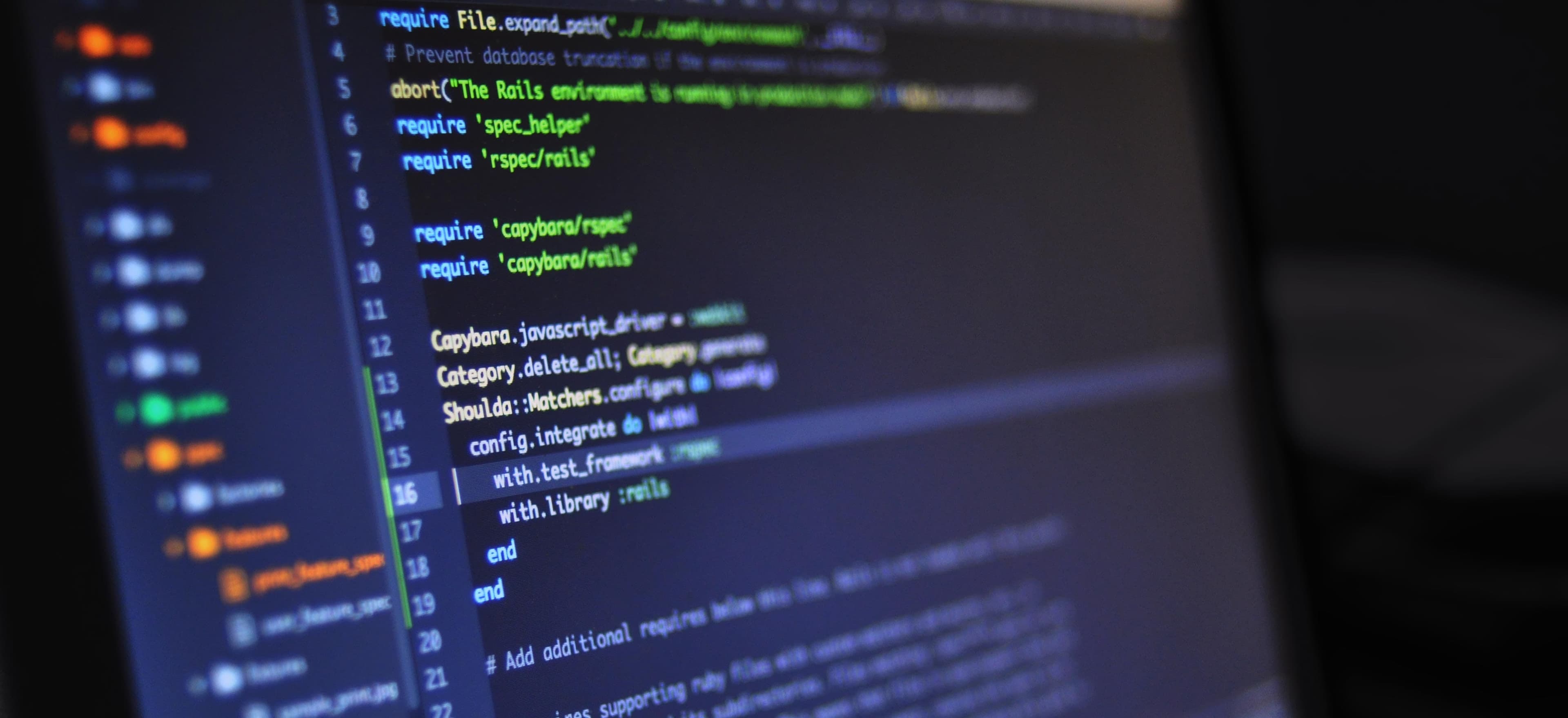
- Published on
Embrace the Power of HTTP/2 in Java 9
In today's fast-paced digital world, it's crucial for Java developers to stay updated with the latest advancements to ensure optimal performance and user experience. With the release of Java 9, one of the notable enhancements is the comprehensive support for HTTP/2, which brings about significant improvements in web performance.
Understanding the Significance of HTTP/2
HTTP/2, the successor of the HTTP/1.1 protocol, is designed to address the limitations and inefficiencies of its predecessor. It introduces several features such as multiplexing, header compression, server push, and more, which collectively result in reduced latency, enhanced concurrency, and improved overall efficiency when compared to HTTP/1.1.
What Does HTTP/2 Bring to Java 9?
Java 9 introduces a standardized HTTP client API that fully supports HTTP/2, providing developers with a seamless and efficient way to leverage the capabilities of this modern protocol. By utilizing HTTP/2, Java applications can benefit from faster page load times, reduced resource utilization, and improved responsiveness.
Embracing HTTP/2 in Your Java 9 Applications
Let's delve into the practical implementation of HTTP/2 support in Java 9. Below, we'll explore the key steps to utilize the HTTP/2 capabilities and optimize your applications for enhanced performance.
Step 1: Creating an HTTP/2 Client
To commence, create an instance of the HttpClient
class with HTTP/2 support enabled by invoking the newBuilder().version(Version.HTTP_2)
method:
HttpClient client = HttpClient.newBuilder()
.version(Version.HTTP_2)
.build();
The HttpClient
class provides a modern, asynchronous, and reactive API for sending and receiving HTTP requests, making it ideal for harnessing the potential of HTTP/2 in Java 9 applications.
Step 2: Issuing HTTP/2 Requests
Next, utilize the created HttpClient
instance to send HTTP/2 requests. The following snippet demonstrates sending a simple GET request to a specified URI:
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://example.com"))
.GET()
.build();
HttpResponse<String> response = client.send(request, BodyHandlers.ofString());
By utilizing the HTTP/2-enabled HttpClient
, Java 9 applications can efficiently communicate with servers that support the protocol, thereby taking advantage of its optimizations and performance benefits.
Step 3: Handling HTTP/2 Responses
Upon receiving a response, handle it according to the requirements of your application. The code snippet below illustrates how to extract and process the response body:
String responseBody = response.body();
System.out.println(responseBody);
By embracing the native support for HTTP/2 in Java 9, developers can streamline the handling of responses and seamlessly integrate these capabilities into their applications.
Verifying HTTP/2 Support
To ensure that the established connections indeed utilize the HTTP/2 protocol, you can inspect the protocol used via the HttpClient
and the received responses:
HttpClient.Version version = client.version();
System.out.println("HTTP version: " + version);
// Check if the response used HTTP/2
boolean isHTTP2 = response.version().equals(Version.HTTP_2);
System.out.println("HTTP/2 used: " + isHTTP2);
By verifying the protocol version and response details, developers can validate the successful integration of HTTP/2 support within their Java 9 applications.
Closing the Chapter
In conclusion, Java 9's built-in support for HTTP/2 equips developers with the tools necessary to enhance the performance and efficiency of their applications. By leveraging the native HTTP/2 capabilities, Java developers can ensure that their applications are well-equipped to meet the demands of modern web development and deliver an optimized user experience.
The transition to HTTP/2 in Java 9 is not just a step towards modernization, but a leap towards maximizing the potential of web applications in today's digital ecosystem. Embracing HTTP/2 is an essential stride for Java developers aiming to stay at the forefront of performance optimization and deliver superior web experiences.
So, what are you waiting for? Dive into Java 9's HTTP/2 support and unlock the potential of faster, more efficient web communications in your applications!
To explore further details, insights, and real-world scenarios of HTTP/2 support in Java 9, refer to the official Java documentation and The Java™ Tutorials for comprehensive coverage.
Start optimizing your Java 9 applications with HTTP/2 today!
Checkout our other articles