Securing SOAP Messages with Apache Camel
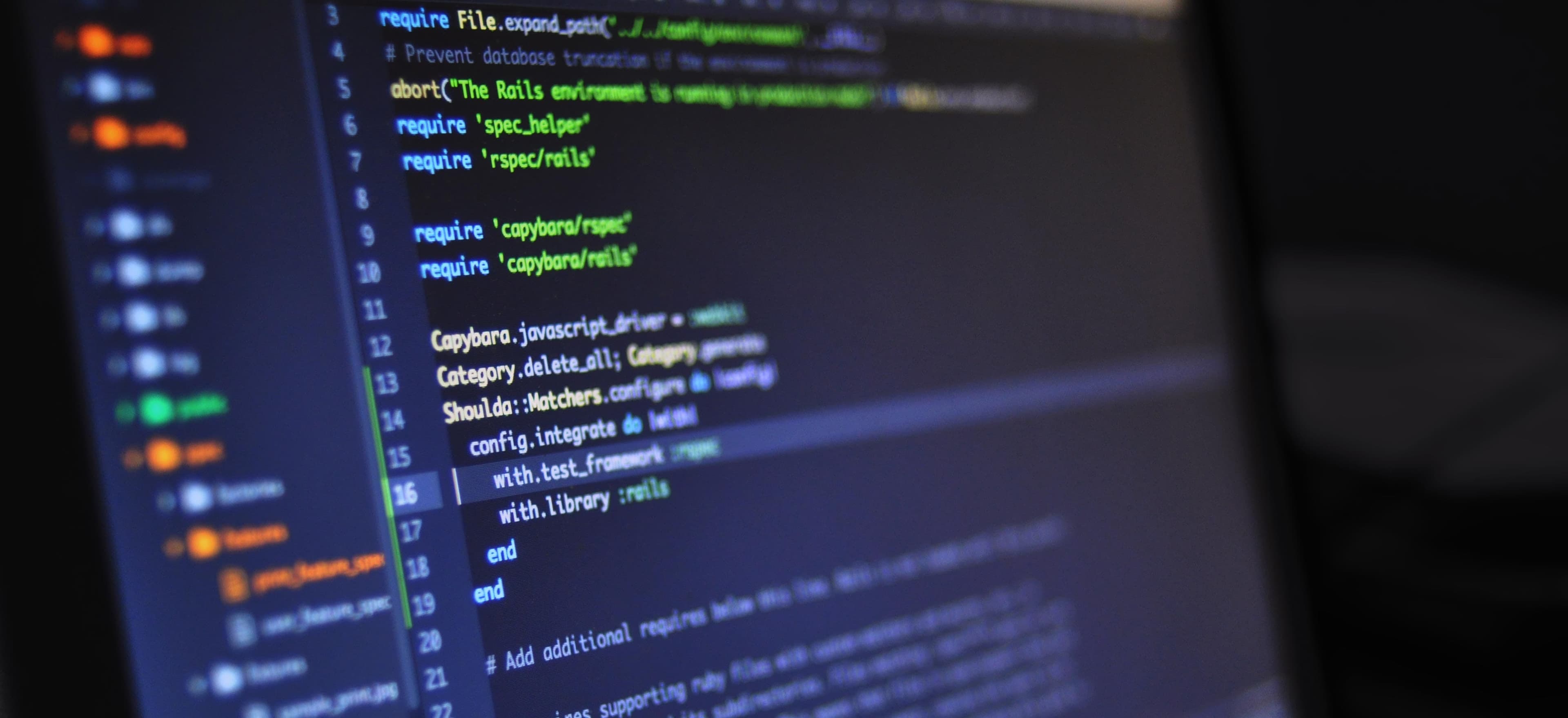
- Published on
Securing SOAP Messages with Apache Camel
In today's interconnected world, securing data exchange between systems is of utmost importance. When dealing with SOAP-based web services, ensuring the confidentiality and integrity of messages is a critical aspect of the system's design. In this article, we will explore how to secure SOAP messages in a Java environment using Apache Camel, a versatile integration framework that provides support for various communication protocols, including SOAP.
Understanding SOAP Message Security
Before diving into the implementation details, let's briefly discuss the fundamentals of securing SOAP messages. When two systems communicate using SOAP, the messages exchanged between them need to be protected from unauthorized access, tampering, and eavesdropping. This is typically achieved through the use of security mechanisms such as encryption, digital signatures, and message authentication codes (MAC).
Encryption
Encryption ensures that the contents of the SOAP message are transformed into an unreadable format using cryptographic algorithms. Only authorized parties possessing the necessary decryption key can revert the message to its original form.
Digital Signatures
Digital signatures provide a means of verifying the authenticity and integrity of the SOAP message. By attaching a digital signature to the message, the sender can prove its identity, and the recipient can validate that the message has not been altered during transit.
Message Authentication Codes (MAC)
Message Authentication Codes (MAC) are used to detect any modifications to the SOAP message during transmission. By calculating a MAC based on the message content and including it with the message, the recipient can verify that the message has not been tampered with.
Implementing Secure SOAP Communication with Apache Camel
Apache Camel provides a robust platform for integrating different systems while offering extensive support for message transformation and routing. By leveraging Camel's capabilities, we can easily implement security measures to protect SOAP messages as they flow through our integration.
Adding Encryption to SOAP Messages
To encrypt SOAP messages in Apache Camel, we can take advantage of the XML Security
component, which offers support for XML encryption. When defining a Camel route for sending a SOAP message, we can use the marshal
and to
DSLs to encapsulate the encryption logic. Let's take a look at an example of how to encrypt a SOAP message using Apache Camel:
from("direct:sendOrder")
.marshal().secureXML()
.to("cxf:bean:someWebServiceEndpoint")
.end();
In this example, the marshal().secureXML()
call instructs Camel to encrypt the outgoing SOAP message before sending it to the specified web service endpoint. By configuring the encryption parameters, such as the encryption algorithm and the recipient's public key, we can ensure that the message remains secure during transmission.
Adding Digital Signatures to SOAP Messages
In addition to encryption, adding digital signatures to SOAP messages is crucial for ensuring message integrity and authenticity. Apache Camel makes it straightforward to incorporate digital signatures into SOAP communication. Let's take a look at how we can attach a digital signature to a SOAP message using Camel:
from("cxf:bean:someWebServiceEndpoint")
.marshal().secureXML()
.to("direct:processResponse")
.end();
from("direct:processResponse")
.marshal().secureXML("http://www.w3.org/2000/09/xmldsig#dsa-sha1")
.to("someProcessorBean")
.end();
In this example, the first route receives the SOAP response from a web service endpoint and applies the encryption using the marshal().secureXML()
call. Subsequently, the response is routed to a processor bean. Within the processor bean, we apply the digital signature to the message using the marshal().secureXML()
with the specified signature algorithm.
Verifying Message Integrity with MAC
To verify the integrity of SOAP messages, we can employ Message Authentication Codes (MAC) in Apache Camel. This ensures that the message has not been tampered with during transmission. Let's consider an example of how to include a MAC with a SOAP message using Camel:
from("direct:sendOrder")
.marshal().secureXML("http://www.w3.org/2000/09/xmldsig#hmac-md5")
.to("cxf:bean:someWebServiceEndpoint")
.end();
In this example, the marshal().secureXML()
call includes a MAC using the specified hashing algorithm with the outgoing SOAP message. This enables the recipient to verify the message's integrity upon receipt.
My Closing Thoughts on the Matter
In this article, we've explored the essential aspects of securing SOAP messages and demonstrated how to implement message security using Apache Camel. By leveraging the encryption, digital signatures, and MAC capabilities provided by Camel's DSL, we can ensure the confidentiality, integrity, and authenticity of SOAP communication in a Java environment.
With Apache Camel's comprehensive support for message transformation and routing, integrating these security measures into SOAP-based systems becomes a seamless process. Whether you're dealing with legacy systems requiring SOAP communication or modernizing your integration architecture, Apache Camel provides a robust foundation for implementing secure web service communication.
If you're interested in learning more about Apache Camel and its capabilities for integrating and securing enterprise systems, be sure to check out the official Apache Camel documentation for detailed insights into its features and usage.
Secure your SOAP messages with confidence using Apache Camel!