Mastering Java XMPP: Overcome Load Test Hurdles!
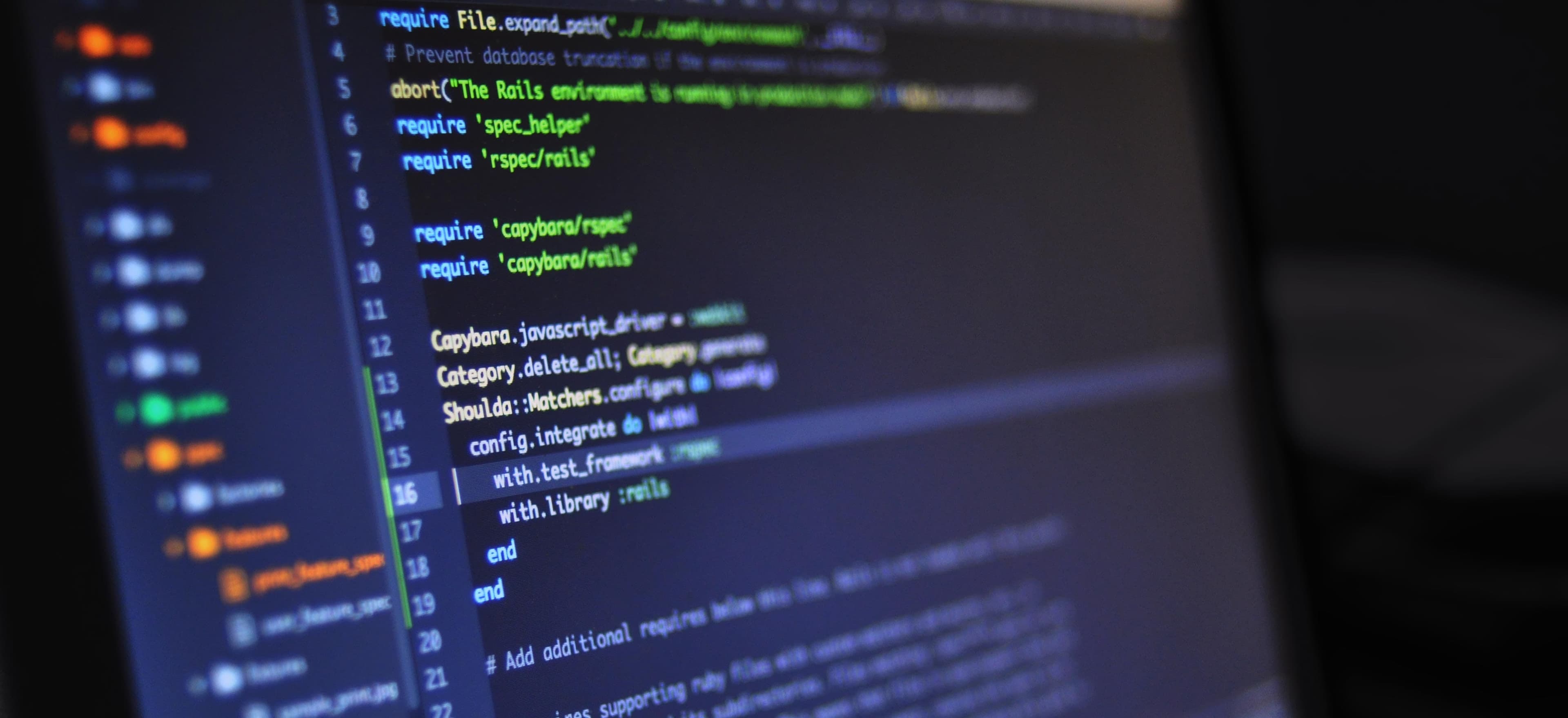
- Published on
Mastering Java XMPP: Overcome Load Test Hurdles!
When it comes to developing real-time messaging applications, Java has been a go-to language for many developers. Extensible Messaging and Presence Protocol (XMPP) is a powerful communication protocol that can be seamlessly integrated into Java applications to enable real-time messaging. However, ensuring that a Java XMPP application performs well under load is essential for delivering a seamless user experience.
In this guide, we will delve into some best practices for load testing Java XMPP applications, using popular tools and techniques to identify performance bottlenecks, and implementing optimizations to overcome load test hurdles.
Understanding the Importance of Load Testing
Load testing is a critical aspect of application development, especially for real-time messaging applications powered by XMPP. It involves simulating a specific level of load on the application to measure its performance under normal and peak usage conditions.
By conducting load tests, developers can:
- Identify performance bottlenecks.
- Validate the application's scalability.
- Determine the maximum capacity of the application.
- Uncover any potential issues before they impact users in a production environment.
Choosing the Right Tools for Load Testing
Selecting the right tools for load testing Java XMPP applications is crucial to obtaining accurate insights into the application's performance. Among the various tools available, JMeter and Gatling stand out as popular choices for load testing Java applications.
JMeter
JMeter is an open-source, Java-based tool that provides a user-friendly interface for load testing various types of applications, including XMPP-based applications. Its extensibility through plugins and a wide range of supported protocols make it an ideal choice for testing Java XMPP applications.
Gatling
Gatling is another powerful open-source load testing tool designed for testing web-based, HTTP, and WebSocket applications. Its user-friendly DSL for writing test scenarios and real-time reports make it a valuable tool for load testing Java XMPP applications with complex real-time messaging requirements.
Writing Effective Load Test Scenarios
Before diving into load testing, it's essential to define realistic test scenarios that simulate the expected user behavior and load on the Java XMPP application. Understanding the typical usage patterns and message exchange frequencies within the application is crucial for crafting effective load test scenarios.
Let's consider a basic example of a load test scenario using Gatling's DSL to simulate a chat application's activity:
package simulations
import io.gatling.core.Predef._
import io.gatling.http.Predef._
import scala.concurrent.duration._
class XMPPChatSimulation extends Simulation {
val httpProtocol = http
.baseUrl("http://your-xmpp-application.com")
.wsBaseUrl("ws://your-xmpp-application.com")
val scn = scenario("XMPP Chat Simulation")
.exec(ws("Connect").connect("/chat"))
.pause(1)
.exec(ws("Send Message").sendText("Hello, world!"))
.pause(1)
.exec(ws("Close").close)
setUp(
scn.inject(
rampUsers(100) during (10 seconds)
).protocols(httpProtocol)
)
}
In this example, we define a scenario to simulate users connecting to the XMPP chat, sending a message, and then disconnecting. The rampUsers
setting gradually increases the number of concurrent users over a specified duration.
Identifying Performance Bottlenecks
Once the load tests are executed, it is crucial to analyze the results to identify any performance bottlenecks within the Java XMPP application. Common areas to focus on include:
- Connection Management: Evaluate how the application handles a large number of simultaneous connections, especially in scenarios with rapid connection creation and teardown.
- Message Throughput: Measure the application's ability to handle a high volume of messages and concurrent message exchanges.
- Resource Utilization: Monitor CPU, memory, and network utilization to pinpoint any resource-related bottlenecks under load.
Optimizing Java XMPP Applications for Performance
After identifying performance bottlenecks, it's time to implement optimizations to enhance the performance of the Java XMPP application under load. Let's explore some key optimization strategies.
Connection Pooling
Implementing a connection pooling mechanism can significantly improve the application's scalability and performance by reusing established connections rather than creating a new connection for each user interaction. Apache Commons Pool provides an efficient pooling library for managing resources such as XMPP connections in Java applications.
Here's a snippet demonstrating the usage of Apache Commons Pool for XMPP connection pooling:
GenericObjectPoolConfig<JabberConnection> poolConfig = new GenericObjectPoolConfig<>();
JabberConnectionFactory factory = new JabberConnectionFactory(...);
ObjectPool<JabberConnection> connectionPool = new GenericObjectPool<>(factory, poolConfig);
In this example, we configure a connection pool using the GenericObjectPool
class and a custom JabberConnectionFactory
to manage XMPP connections efficiently.
Asynchronous Message Processing
Utilizing asynchronous message processing techniques, such as Java's CompletableFuture or reactive programming libraries like RxJava, can improve the application's responsiveness and throughput by handling incoming messages concurrently. By leveraging asynchronous processing, the application can effectively utilize available resources to handle a large number of message exchanges without blocking threads.
CompletableFuture<Void> sendMessageAsync(String recipient, String message) {
return CompletableFuture.runAsync(() -> {
// Send message logic
});
}
In this code snippet, the sendMessageAsync
method uses CompletableFuture
to asynchronously send a message to the specified recipient without blocking the calling thread.
Resource Cleanup and Garbage Collection Optimization
Proper resource cleanup, especially in the context of XMPP connections and associated resources, is crucial for maintaining optimal application performance. Additionally, optimizing garbage collection settings and reducing the impact of garbage collection pauses can contribute to better overall application responsiveness under load.
// Ensure resource cleanup
try {
// Clean up resources
} catch (Exception e) {
// Handle cleanup errors
} finally {
// Release resources
}
In this example, resource cleanup is performed within a try-finally
block to ensure proper release of resources, minimizing the risk of resource leaks affecting the application's performance.
Utilizing NIO for Non-blocking I/O
Java's Non-blocking I/O (NIO) capabilities, offered through classes like Selector
and Channel
, enable efficient handling of numerous simultaneous connections and message exchanges without the need for a separate thread per connection. This can significantly enhance the application's ability to scale and handle high loads while maintaining responsiveness.
Selector selector = Selector.open();
Channel channel = Channel.open();
channel.configureBlocking(false);
In this snippet, a Selector
is used to multiplex non-blocking I/O operations, allowing efficient handling of multiple incoming connections and messages within a single thread.
My Closing Thoughts on the Matter
Mastering Java XMPP load testing and optimization is crucial for delivering high-performance, real-time messaging applications. By choosing the right load testing tools, crafting effective test scenarios, identifying performance bottlenecks, and implementing targeted optimizations, developers can ensure that their Java XMPP applications can handle the demands of real-world usage with ease.
As real-time communication continues to play a pivotal role in the digital landscape, the ability to overcome load test hurdles and optimize Java XMPP applications is a valuable skill that can elevate the quality of user experiences and the overall success of communication-driven software solutions.
Checkout our other articles