Master Named Entity Graphs in JPA 2.1: A How-To Guide
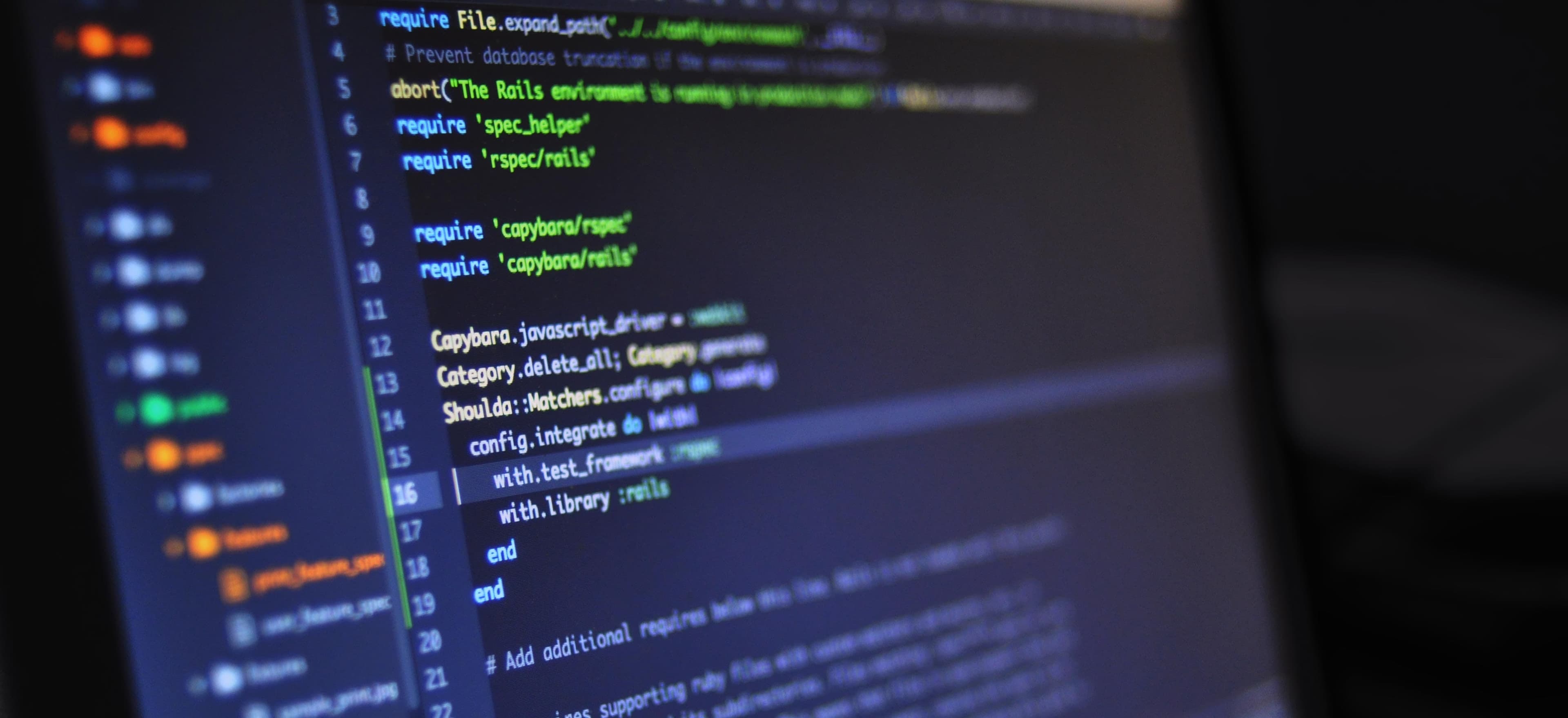
- Published on
Master Named Entity Graphs in JPA 2.1: A How-To Guide
When working with Java Persistence API (JPA), fine-tuning the fetching strategy for entities is crucial for optimizing performance. Named entity graphs, introduced in JPA 2.1, provide a powerful mechanism to define a graph of related entities and their fetch strategies. In this guide, we will delve deep into named entity graphs, exploring their benefits and demonstrating how to utilize them effectively in your Java applications.
What are Named Entity Graphs?
In JPA, fetching strategies define how related entities are loaded from the database. By default, associations are lazily fetched, causing additional queries to be executed when accessing related entities. Named entity graphs allow developers to explicitly specify the fetch strategies for related entities, enabling the preloading of these associations in a single database query.
Benefits of Named Entity Graphs
Named entity graphs offer several advantages, including:
- Performance Optimization: By fetching related entities in a single query, named entity graphs help reduce the number of database round trips, thereby improving overall performance.
- Fine-grained Control: Developers can precisely define which attributes and associations to eagerly fetch, avoiding the issues of over-fetching or under-fetching data.
- Reusability: Once defined, named entity graphs can be reused across multiple queries, promoting code reusability and maintainability.
Defining Named Entity Graphs
To demonstrate the use of named entity graphs, let's consider an example where we have Author
and Book
entities with a one-to-many relationship. We want to eagerly fetch the author details when querying for books. To achieve this, we'll define a named entity graph for the Book
entity.
@Entity
@Table(name = "book")
@NamedEntityGraph(
name = "graph.BookWithAuthor",
attributeNodes = @NamedAttributeNode("author")
)
public class Book {
// Other attributes and methods
@ManyToOne
@JoinColumn(name = "author_id")
private Author author;
// Getters and setters
}
In the above snippet, we annotate the Book
entity with @NamedEntityGraph
and specify the name of the graph (BookWithAuthor
) along with the attribute to be eagerly fetched (author
).
Using Named Entity Graphs in Queries
Once we have defined the named entity graph, we can utilize it in a JPA query to ensure that the specified attributes are eagerly fetched. Here's an example of using the named entity graph in a JPA query:
@Entity
@Table(name = "book")
@NamedEntityGraph(
name = "graph.BookWithAuthor",
attributeNodes = @NamedAttributeNode("author")
)
public class Book {
// Other attributes and methods
}
The above code snippet shows the usage of named entity graphs in a JPA query, where the named entity graph graph.BookWithAuthor
is referenced in the query to ensure that the author
attribute is eagerly fetched along with the Book
entity.
Combining Named Entity Graphs with Criteria API
In addition to JPQL queries, named entity graphs can also be used with the JPA Criteria API. This allows for the programmatic construction of queries using a type-safe and object-oriented approach.
public List<Book> getBooksAndAuthors(EntityManager em) {
CriteriaBuilder cb = em.getCriteriaBuilder();
CriteriaQuery<Book> cq = cb.createQuery(Book.class);
Root<Book> book = cq.from(Book.class);
book.fetch("author", JoinType.INNER);
EntityGraph<Book> graph = em.createEntityGraph(Book.class);
graph.addAttributeNodes("author");
TypedQuery<Book> typedQuery = em.createQuery(cq);
typedQuery.setHint("javax.persistence.loadgraph", graph);
return typedQuery.getResultList();
}
In the code snippet above, we use the Criteria API to construct a query for fetching books along with their authors. We create an entity graph for the Book
entity and specify the attribute author
to be eagerly fetched. This approach provides a flexible and type-safe way to utilize named entity graphs.
The Last Word
Named entity graphs in JPA 2.1 offer a powerful solution for optimizing data fetching strategies, providing enhanced control over the retrieval of related entities. By defining and utilizing named entity graphs, developers can achieve significant performance improvements and ensure efficient data retrieval. Additionally, the reusability and flexibility of named entity graphs make them a valuable tool in the JPA arsenal.
In summary, mastering named entity graphs in JPA 2.1 is not just a best practice, but a fundamental skill for crafting high-performance, efficient Java applications with robust data access layers.
To deepen your understanding, you can explore the official documentation on JPA 2.1 Entity Graphs.
Start leveraging the power of named entity graphs today and elevate the performance of your JPA-based applications!