Conquering Complexity: Java Meets Boxing Analytics
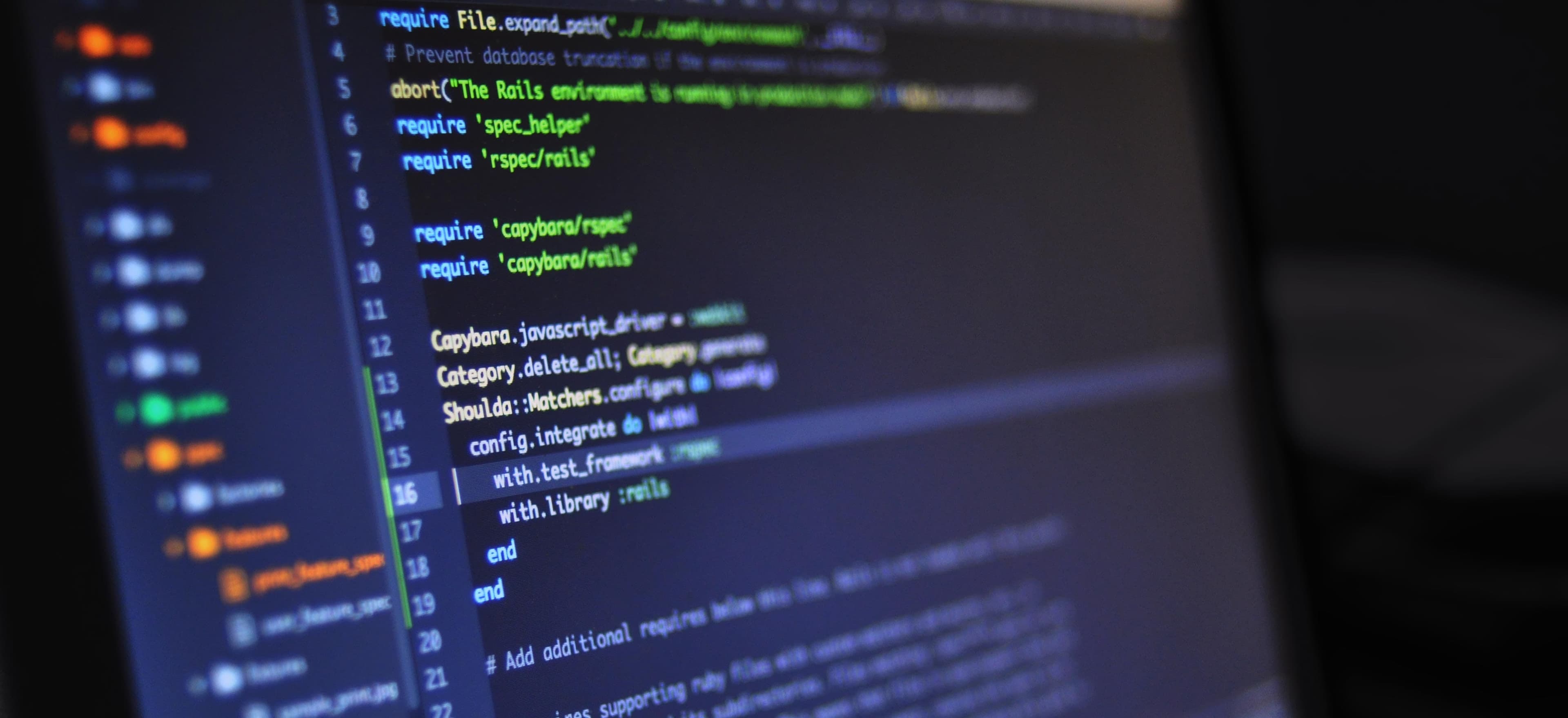
- Published on
Conquering Complexity: Java Meets Boxing Analytics
In the world of sports analytics, the combination of Java and boxing may seem unlikely at first glance. However, the power and flexibility of Java make it an ideal choice for handling the complex data and computational requirements of boxing analytics. In this post, we'll explore how Java can be leveraged to analyze and gain insights from boxing data, shedding light on the technical aspects and the practical implications.
The Challenge of Boxing Analytics
Boxing, known as the sweet science, involves intricate movements, precise striking techniques, and strategic decisions, making it a rich field for data analysis. From punch statistics to fighter movement patterns, there's a wealth of data to be processed and analyzed. However, handling and making sense of this data poses a significant challenge, requiring robust computational tools and algorithms.
Why Java?
Java's popularity and versatility in handling large-scale data processing make it an excellent choice for boxing analytics. Its rich ecosystem of libraries, strong support for parallel processing, and platform independence are crucial factors in tackling the complexity of boxing data. Additionally, Java's static typing and object-oriented approach promote maintainability and scalability, essential for building robust analytics applications.
Leveraging Java for Boxing Analytics
Data Ingestion and Processing
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class BoxingDataProcessor {
public static void main(String[] args) {
try {
File dataFile = new File("boxing_data.csv");
Scanner scanner = new Scanner(dataFile);
while (scanner.hasNextLine()) {
String data = scanner.nextLine();
// Process the data
// ...
}
scanner.close();
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e.getMessage());
}
}
}
Java's file I/O capabilities, combined with its exception handling, provide a robust foundation for ingesting and processing large volumes of boxing data. The above snippet demonstrates how Java can read a CSV file containing boxing data, paving the way for subsequent analysis.
Statistical Analysis with Apache Commons Math
import org.apache.commons.math3.stat.StatUtils;
public class BoxingStatistics {
public static void main(String[] args) {
double[] punchData = {73, 65, 82, 78, 70}; // Example punch data
double mean = StatUtils.mean(punchData);
double variance = StatUtils.variance(punchData);
// Perform more statistical computations
// ...
}
}
By leveraging the Apache Commons Math library, Java empowers boxing analysts to perform sophisticated statistical computations. The snippet above showcases how Java can easily calculate the mean and variance of punch data, laying the groundwork for deeper statistical insights.
Machine Learning with Weka
import weka.classifiers.Classifier;
import weka.classifiers.functions.SMO;
import weka.core.Attribute;
import weka.core.FastVector;
import weka.core.Instance;
import weka.core.Instances;
public class BoxingPredictor {
public static void main(String[] args) {
// Data preparation
FastVector attributes = new FastVector();
// Add attributes
// ...
Instances dataset = new Instances("boxing_dataset", attributes, 0);
// Build and train the classifier
Classifier classifier = new SMO();
classifier.buildClassifier(dataset);
// Make predictions
Instance newBoxer = new Instance(4);
// Set attribute values
// ...
double prediction = classifier.classifyInstance(newBoxer);
// Handle the prediction
// ...
}
}
Integrating the Weka machine learning library into Java opens the door to building predictive models for boxing analytics. The provided snippet illustrates the process of preparing a boxing dataset, training a Support Vector Machine (SMO) classifier, and making predictions based on new data, demonstrating Java's prowess in machine learning.
A Final Look
Java's combination of robust data processing, statistical analysis, and machine learning capabilities makes it a potent tool for conquering the complexity of boxing analytics. By harnessing Java's strengths, analysts and developers can unlock valuable insights from boxing data, ultimately shaping the future of the sport.
In this post, we've only scratched the surface of Java's potential in the realm of boxing analytics. Its adaptability, performance, and extensive ecosystem make it a formidable contender in the arena of sports data analysis. As boxing continues to evolve, Java stands ready to wield its analytical might, unraveling the intricacies of the sport and driving it to new heights.
To delve deeper into the world of sports analytics, including boxing and beyond, check out SportTechie and ESPN's sports analytics coverage.
Checkout our other articles