Optimizing JXLS for Efficient Excel File Parsing
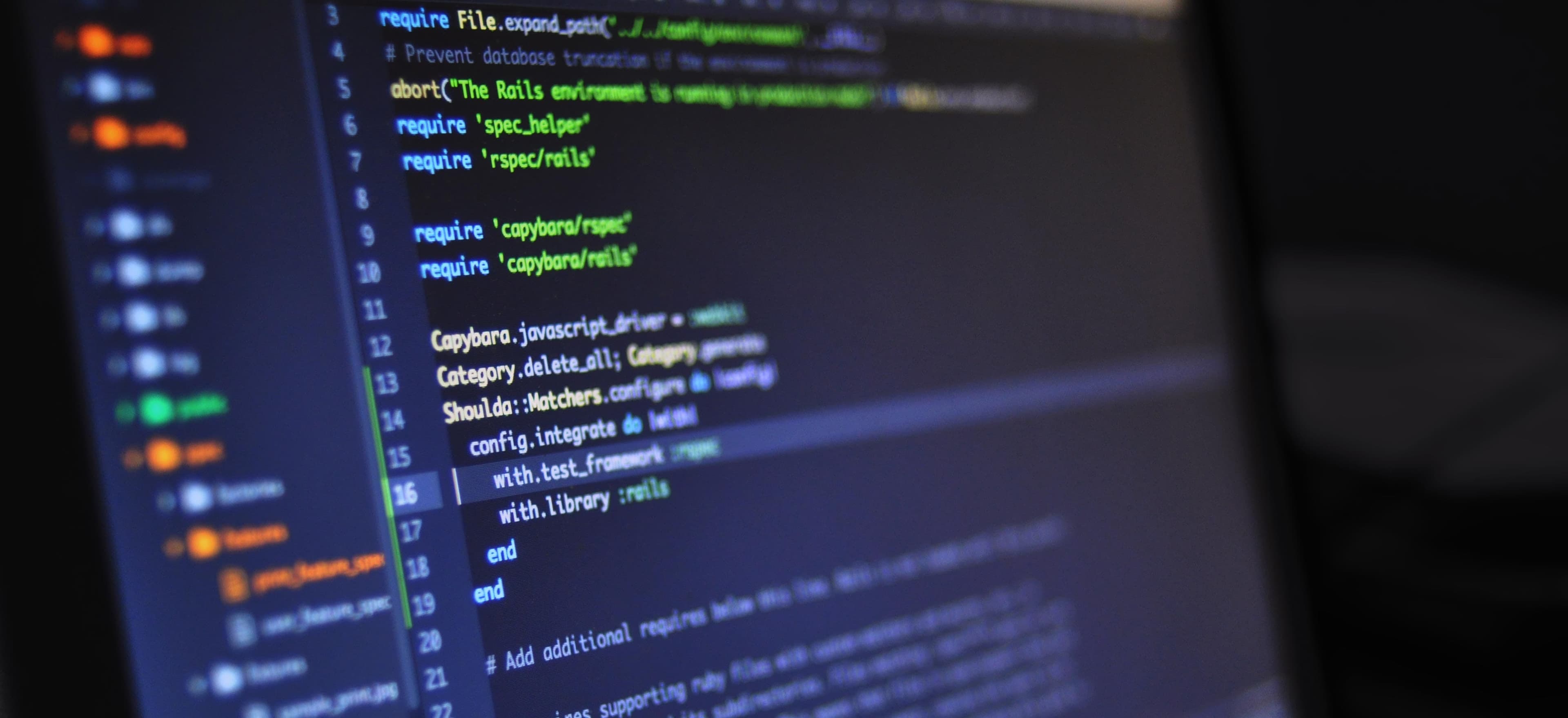
- Published on
Optimizing JXLS for Efficient Excel File Parsing
Working with Excel files in Java can be a challenging task, especially when dealing with large or complex spreadsheets. The JXLS library is a powerful tool that can help simplify the process of reading, writing, and manipulating Excel files using Java. However, as with any library, there are strategies and optimizations that can be employed to ensure efficient file parsing. In this post, we will explore some tips and best practices for optimizing JXLS for efficient Excel file parsing.
Understanding JXLS
JXLS is an open-source Java library that provides a set of tools for working with Excel files. It allows developers to generate Excel files from templates, as well as parse and manipulate existing Excel files. By using JXLS, developers can automate the process of filling data into Excel templates, making it a versatile tool for generating reports, exports, and other data-driven documents.
Tip 1: Use Streaming
When working with large Excel files, it's important to consider memory usage and performance. One way to optimize file parsing with JXLS is to utilize streaming for reading and writing Excel files. By using a streaming approach, data is processed in chunks, reducing memory overhead and improving overall performance.
// Reading Excel file with JXLS using streaming
try (InputStream input = new FileInputStream("input.xlsx")) {
List<CustomObject> data = // retrieve data to be written to the Excel file
try (OutputStream output = new FileOutputStream("output.xlsx")) {
Context context = new Context();
context.putVar("data", data);
JxlsHelper.getInstance().processGridTemplateAtCell(input, output, context, "Sheet1!A1");
}
}
In the example above, we use the processGridTemplateAtCell
method from JxlsHelper
to process the Excel file using streaming. This approach is particularly useful when dealing with large datasets, as it reduces the memory footprint by processing data in smaller chunks.
Tip 2: Optimize Template Design
Another important aspect of optimizing JXLS for efficient Excel file parsing is to carefully design the Excel templates. By following best practices for template design, such as minimizing the use of complex formulas, reducing the number of merged cells, and organizing data in a structured format, the parsing and processing of Excel files can be significantly improved.
// Define a simple Excel template for JXLS
Cell A1: ${data.header1}
Cell A2: ${data.value1}
Cell A3: ${data.header2}
Cell A4: ${data.value2}
In the template example above, we use simple cell references to map the data to specific cells in the Excel file. By avoiding complex formulas and cell manipulations, we can enhance the parsing efficiency when using JXLS to process the template.
Tip 3: Utilize Parallel Processing
For scenarios where high-performance Excel file parsing is crucial, leveraging parallel processing can be beneficial. JXLS provides the flexibility to parallelize the processing of Excel files, thereby improving the overall throughput and efficiency.
// Parallel processing of Excel files using JXLS
List<CustomObject> data = // retrieve and organize data for parallel processing
ExecutorService executor = Executors.newFixedThreadPool(4);
for (CustomObject obj: data) {
executor.submit(() -> {
// Process each data object and generate Excel file using JXLS
});
}
executor.shutdown();
executor.awaitTermination(Long.MAX_VALUE, TimeUnit.NANOSECONDS);
In the code snippet above, we utilize the ExecutorService
to parallelize the processing of data and Excel file generation. By allocating tasks to multiple threads, we can take advantage of multi-core architectures and accelerate the file parsing process.
Tip 4: Cache Template Processing
To further optimize the performance of JXLS for Excel file parsing, consider caching the processed templates. By caching the intermediate representation of the processed template, subsequent parsing operations can benefit from reduced overhead and improved speed.
// Cache processed Excel template for efficient file parsing
InputStream template = new FileInputStream("template.xlsx");
Transformer transformer = TransformerFactory.createTransformer(template, output);
transformer.setCache(true);
transformer.transform();
In the example above, we set the cache
property of the Transformer
to true
, enabling the caching of the processed template. This approach is especially useful when repeatedly parsing Excel files based on the same template, as it eliminates the need to reprocess the template for each parsing operation.
My Closing Thoughts on the Matter
Optimizing JXLS for efficient Excel file parsing is essential when working with large or complex datasets. By leveraging streaming, optimizing template design, utilizing parallel processing, and caching template processing, developers can significantly improve the performance and throughput of file parsing operations using JXLS. Incorporating these tips and best practices will not only enhance the efficiency of parsing Excel files but also contribute to a more reliable and scalable application.
In conclusion, understanding the nuances of JXLS and implementing optimization techniques can streamline the process of working with Excel files in Java, ultimately leading to improved performance and a better user experience.
By following the tips and best practices outlined in this post, developers can optimize JXLS for efficient Excel file parsing, leading to improved performance and reliability in their Java applications.