Overcoming Data Consistency Hurdles in Microservices
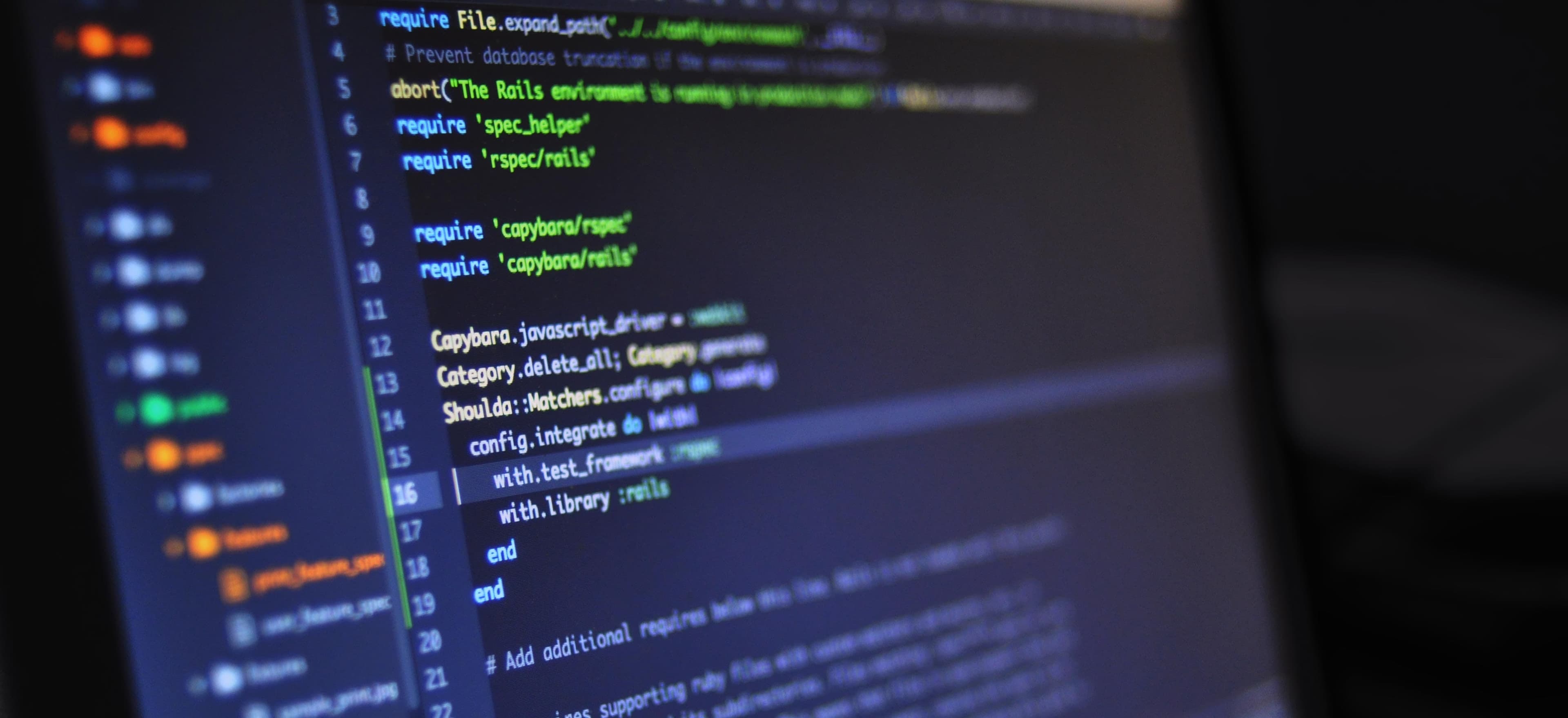
- Published on
Overcoming Data Consistency Hurdles in Microservices
Microservices architecture has gained immense popularity due to its ability to break down complex applications into smaller, easily manageable services. However, with the benefits of microservices also come various challenges, one of which is ensuring data consistency across multiple services. In this blog post, we'll delve into the intricacies of maintaining data consistency in a microservices environment, explore different strategies to tackle this challenge, and how Java plays a pivotal role in overcoming these hurdles.
Understanding Data Consistency in Microservices
In a microservices architecture, each service manages its own database. This autonomy, while beneficial for scalability and independent deployment, presents a significant challenge in ensuring that data remains consistent across multiple services. For example, consider a scenario where two services need to update related data concurrently. In a monolithic architecture, this would typically be managed within a single database transaction. In a microservices environment, however, coordinating such transactions becomes more complex.
Challenges of Data Consistency in Microservices
1. Distributed Transactions
Ensuring data consistency across services in a distributed system often involves coordinating transactions between multiple databases. Traditional ACID transactions, which are relatively straightforward in a monolithic architecture, become challenging to implement in a microservices environment due to the distributed nature of the data.
2. Eventual Consistency
Microservices often rely on asynchronous communication and eventual consistency to propagate changes across the system. This means that in certain situations, data may not be immediately consistent across services, leading to potential inconsistencies during the propagation period.
3. Polyglot Persistence
With each microservice having its own database, the choice of databases could vary across services, leading to data storage in different formats and requiring synchronization mechanisms to maintain data consistency.
Strategies for Ensuring Data Consistency
1. Synchronous Communication and Saga Pattern
In scenarios where immediate consistency is crucial, synchronous communication between services can be employed to coordinate transactions. The Saga pattern, which involves breaking down a distributed transaction into a series of local transactions and compensating transactions, helps in maintaining data consistency across services.
// Example of Saga pattern in Java using Spring Boot and REST
@PostMapping("/order")
@Transactional
public void placeOrder(@RequestBody Order order) {
// Local transaction to reserve inventory
inventoryService.reserveInventory(order);
// Local transaction to process payment
paymentService.processPayment(order);
// If any step fails, trigger compensating transactions
// ...
}
In the above code, the placeOrder
method demonstrates the use of the Saga pattern by coordinating multiple local transactions within a single service.
2. Asynchronous Event-Driven Communication
Utilizing asynchronous event-driven communication, such as with Apache Kafka or RabbitMQ, enables services to communicate and react to events without the need for immediate consistency. This approach allows for eventual consistency while ensuring that eventual states converge to a consistent state.
// Example of asynchronous event-driven communication in Java with Apache Kafka
@KafkaListener(topics = "order-events")
public void handleOrderEvent(OrderEvent orderEvent) {
// Process order event and update local database
}
In this example, the handleOrderEvent
method demonstrates how a service can react to order events asynchronously, maintaining eventual consistency across services.
3. Compensating Transactions
When inconsistencies occur in a microservices environment, compensating transactions can be utilized to rollback or correct the effects of a preceding transaction. This approach is particularly useful in scenarios where maintaining immediate consistency is challenging.
// Example of compensating transaction in Java
public void processOrder(Order order) {
try {
// Process order
// ...
} catch (Exception e) {
// Compensating transaction to rollback or correct effects
// ...
}
}
The processOrder
method showcases how compensating transactions can be used in Java to handle inconsistencies that may arise during the processing of an order.
Java's Role in Overcoming Data Consistency Hurdles
Java, with its robust ecosystem and libraries, plays a vital role in addressing data consistency challenges in microservices. Frameworks such as Spring Boot provide comprehensive support for implementing patterns like Saga and managing distributed transactions, while libraries like Hibernate facilitate ORM (Object-Relational Mapping) to handle polyglot persistence.
Java's support for multithreading and asynchronous programming also enables seamless integration with event-driven communication systems, allowing for the effective handling of eventual consistency.
In addition, Java's strong type system and exception handling capabilities make it well-suited for implementing compensating transactions, providing a reliable foundation for managing inconsistencies within microservices.
The Bottom Line
Ensuring data consistency in a microservices environment is a complex yet crucial aspect of designing and maintaining distributed systems. By employing strategies such as Saga pattern, asynchronous event-driven communication, and compensating transactions, along with leveraging the capabilities of Java and its ecosystem, developers can effectively overcome the data consistency hurdles that arise in microservices architecture.
By understanding the challenges and implementing appropriate strategies supported by Java, organizations can harness the benefits of microservices without compromising on data consistency, ultimately leading to robust and reliable distributed systems.
In conclusion, Java's extensive capabilities and libraries make it an ideal choice for tackling data consistency challenges in microservices, empowering developers to build resilient and consistent distributed systems.
Through the adoption of robust architecture and leveraging Java's features, organizations can ensure that their microservices-based applications maintain data consistency, allowing them to fully realize the benefits of this architectural approach.
Incorporating an appropriate combination of architectural patterns, along with Java's capabilities, ultimately results in the development of agile, responsive, and consistent microservices-based solutions.
Remember, the key to overcoming data consistency hurdles in microservices lies in understanding the challenges and employing the right strategies, supported by the robust capabilities of Java and its ecosystem.
To delve deeper into the world of microservices and gain a comprehensive understanding of the strategies and tools available for overcoming data consistency challenges, explore this article from NGINX.
For insights into the latest trends and best practices in microservices architecture, take a look at TechBeacon's guide on understanding microservices and legacy data strategies.