Handling Websocket Timeout in JDeveloper 12.1.3
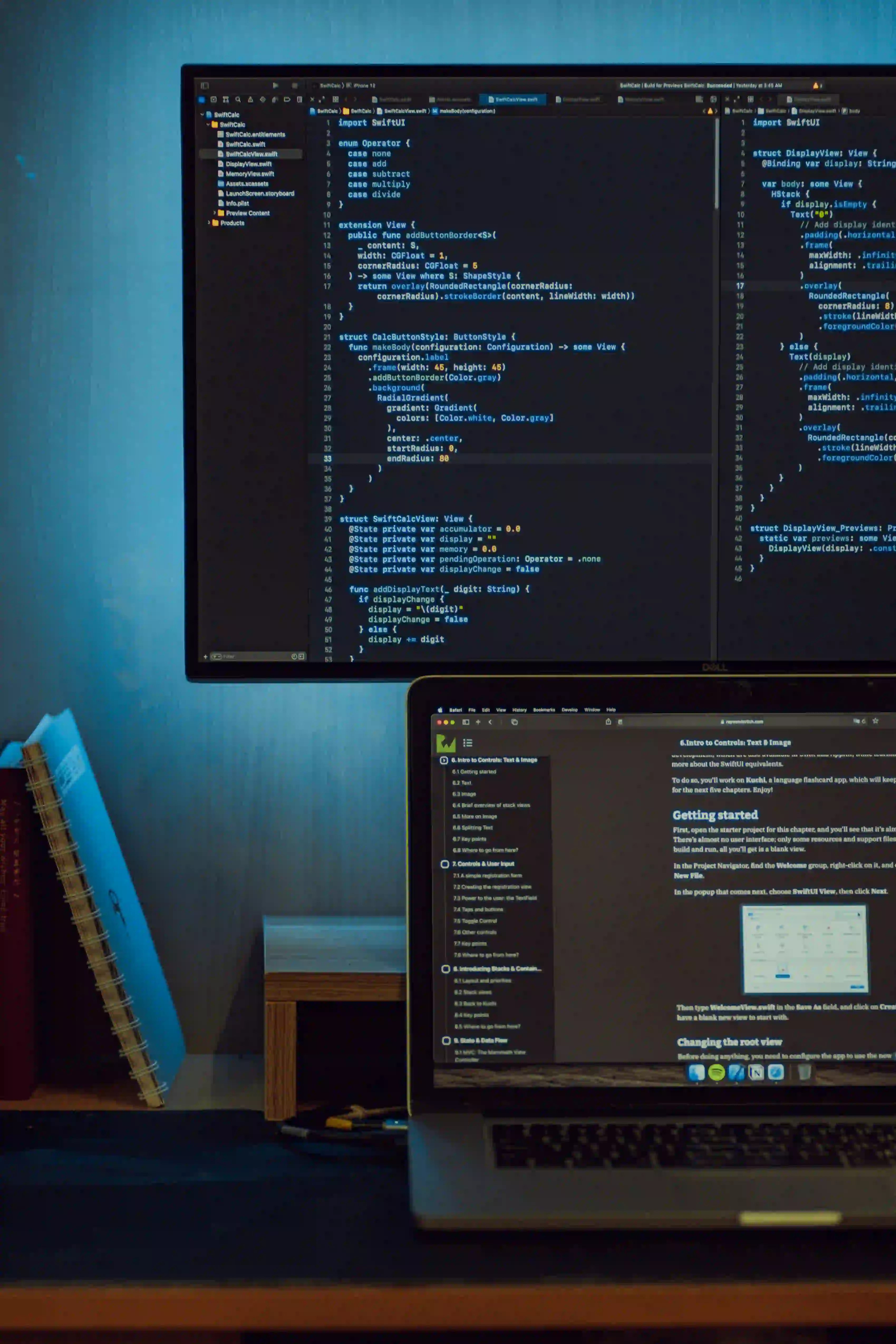
Handling Websocket Timeout in JDeveloper 12.1.3
In the world of web development, WebSockets have become an essential part of real-time communication between a client and a server. However, when integrating WebSockets into applications, developers often encounter challenges such as timeouts. In this blog post, we will explore how to handle Websocket timeouts in JDeveloper 12.1.3, ensuring a seamless and reliable real-time communication experience for users.
Understanding Websocket Timeout
Before diving into the technical aspects of handling Websocket timeouts, it's crucial to understand what a timeout is in the context of WebSockets. A timeout occurs when a client or server does not receive any communication from the other party within a specified period. In the case of WebSockets, timeouts can disrupt real-time data transfer and lead to an inconsistent user experience.
Configuring Websocket Timeout in JDeveloper
In JDeveloper 12.1.3, you can configure Websocket timeouts to ensure robust and uninterrupted communication. Let's take a closer look at the steps involved in handling Websocket timeouts effectively.
Step 1: Setting Connection Timeout
When establishing a Websocket connection in JDeveloper, it's important to set an appropriate connection timeout. This timeout determines the maximum time the client will wait for the connection to be established before considering it a failure.
WebSocketContainer container = ContainerProvider.getWebSocketContainer();
container.setDefaultMaxSessionIdleTimeout(60000); // Set timeout to 60 seconds
In this example, we use setDefaultMaxSessionIdleTimeout
to set the maximum session idle timeout to 60 seconds. Adjust the timeout value based on the specific requirements of your application.
Step 2: Handling Timeout Events
Handling timeout events is essential for gracefully managing Websocket timeouts. In JDeveloper 12.1.3, you can register a timeout handler to take proactive actions when a timeout occurs.
session.addMessageHandler(new MessageHandler.Whole<String>() {
@Override
public void onTimeout(WebSocketSession session) {
// Handle timeout event
}
});
By implementing a timeout handler, you can perform tasks such as logging the timeout event, notifying the user, or attempting to re-establish the connection.
Step 3: Implementing Retry Mechanism
To enhance the reliability of Websocket communication, implementing a retry mechanism is a best practice. In JDeveloper, you can encapsulate the connection logic within a retry mechanism to automatically attempt reconnection in the event of a timeout.
private void establishWebSocketConnection() {
try {
// Attempt to establish Websocket connection
} catch (TimeoutException e) {
// Retry the connection
}
}
By encapsulating the connection logic within a retry mechanism, you can effectively handle and recover from Websocket timeouts, ensuring minimal disruption to the user experience.
The Bottom Line
In conclusion, handling Websocket timeouts in JDeveloper 12.1.3 is crucial for ensuring robust and reliable real-time communication within web applications. By configuring connection timeouts, handling timeout events, and implementing a retry mechanism, developers can effectively mitigate the impact of timeouts and deliver a seamless user experience.
With the techniques and best practices discussed in this blog post, you are now equipped to proactively manage Websocket timeouts in your JDeveloper projects, enhancing the overall reliability and performance of real-time communication features.
For more in-depth information on WebSockets and best practices for managing timeouts, consider exploring the Oracle WebSockets documentation, which provides valuable insights into the intricacies of Websocket communication in JDeveloper.
Now, it's time to put your newfound knowledge into practice and elevate the real-time communication capabilities of your JDeveloper applications!