Tuple Confusion: Navigating Subtype Polymorphism Pitfalls
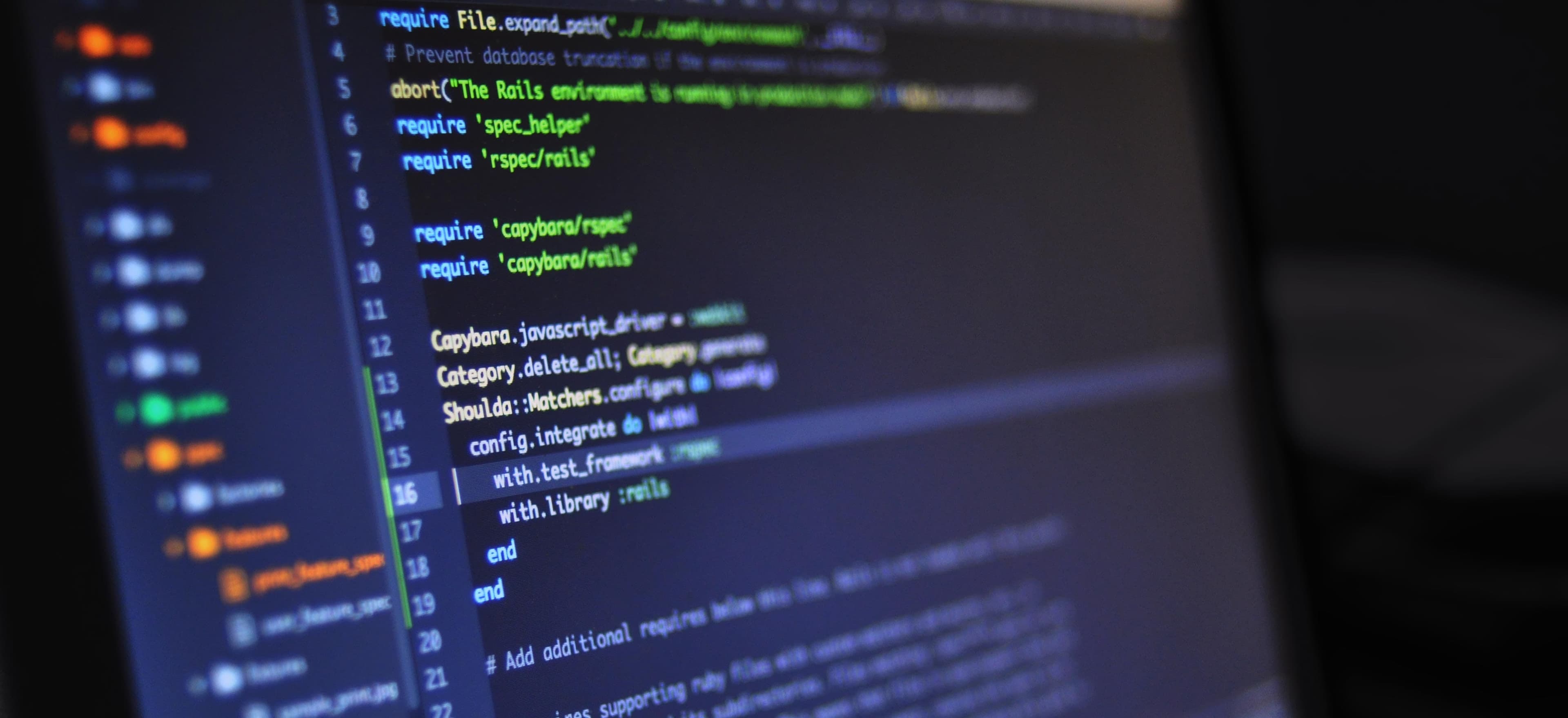
- Published on
Tuple Confusion: Navigating Subtype Polymorphism Pitfalls
Subtype polymorphism is a powerful mechanism in object-oriented programming, allowing a subclass to be treated as its superclass. However, when dealing with tuples and subtype polymorphism in Java, things can get confusing, leading to subtle bugs and unexpected behavior. In this article, we'll explore the pitfalls of using tuples with subtype polymorphism in Java and how to navigate them effectively.
Understanding Subtype Polymorphism
Subtype polymorphism, a fundamental concept in object-oriented programming, allows a subclass to be treated as its superclass. This means that an instance of a subclass can be used wherever an instance of its superclass is expected. This concept forms the basis of the Liskov Substitution Principle, a key tenet of object-oriented design.
In Java, subtype polymorphism is achieved through class inheritance and method overriding. When a subclass overrides a method from its superclass, it provides a specialized implementation while still adhering to the contract defined by the superclass. This flexibility allows different subclasses to be used interchangeably with their superclass, providing a high degree of flexibility and extensibility in the codebase.
Tuples in Java
In Java, tuples are not a built-in language feature, but they can be simulated using arrays, collections, or third-party libraries such as Apache Commons Lang or Guava. Tuples provide a convenient way to group multiple elements into a single object, often used to return multiple values from a method or as a lightweight data structure.
Let's consider a simple example of a tuple in Java using an array to represent a pair of values:
public class Pair<T1, T2> {
private T1 first;
private T2 second;
public Pair(T1 first, T2 second) {
this.first = first;
this.second = second;
}
public T1 getFirst() {
return first;
}
public T2 getSecond() {
return second;
}
}
In this example, the Pair
class represents a tuple of two elements, where the types of the elements are generic and can be specified at runtime. This type of generic tuple can be useful in various scenarios where returning multiple values is necessary.
Pitfalls of Subtype Polymorphism with Tuples
When working with tuples in the context of subtype polymorphism, it's essential to be aware of the potential pitfalls that may arise. One common pitfall is the loss of type information when using tuples in conjunction with subtype polymorphism.
Consider the following scenario:
public class SubtypePolymorphism {
public Pair<String, String> process() {
// ...
return new Pair<>("Hello", "World");
}
}
In this example, the process
method returns a Pair
of strings. Now, let's assume we have a subclass of Pair
called SubPair
:
public class SubPair<T1, T2, T3> extends Pair<T1, T2> {
private T3 third;
public SubPair(T1 first, T2 second, T3 third) {
super(first, second);
this.third = third;
}
public T3 getThird() {
return third;
}
}
If we attempt to use the SubPair
in a context where a Pair
is expected due to subtype polymorphism, it can lead to confusion and potential runtime errors.
Pair<String, String> pair = new SubPair<>("Hello", "World", "!");
String firstElement = pair.getFirst(); // Potential ClassCastException
In this scenario, although the SubPair
class extends Pair
, it introduces a third element, which may not align with the assumptions made by the code expecting a standard pair. This type of situation can result in unexpected behavior and violate the principle of least astonishment.
Navigating the Pitfalls
To navigate the pitfalls of using tuples with subtype polymorphism in Java, it's essential to consider the following strategies:
Mindful Design
When working with subtype polymorphism and tuples, it's crucial to design classes and interfaces with a clear understanding of their intended use. Avoid introducing unexpected changes in behavior by subclassing tuples with additional elements. If a new type is needed, consider creating a distinct class rather than subtyping an existing tuple.
Use of Interfaces
Instead of directly relying on concrete tuple implementations, consider using interfaces to define tuple-like structures. This allows for a more abstract and flexible approach, enabling different implementations to coexist without the risk of subtype polymorphism conflicts.
Defensive Programming
When dealing with tuples and subtype polymorphism, defensive programming becomes crucial. Always validate the types and structures of tuples before using them in polymorphic contexts to prevent unexpected runtime errors. Type checking and validation can help catch potential issues early in the development process.
Documentation and Communication
Clear documentation and communication regarding the expected behavior of tuples and their subtype relationships can greatly reduce confusion and errors. By explicitly stating the constraints and assumptions surrounding tuple usage and subtype polymorphism, developers can make informed decisions and avoid unintended consequences.
The Bottom Line
Subtype polymorphism is a powerful concept in Java, enabling flexible and extensible code design. When combined with tuples, however, it introduces potential pitfalls that require careful navigation. By understanding the limitations of tuples in the context of subtype polymorphism and following best practices in design and defensive programming, developers can effectively navigate the complexities and ensure the reliability of their code.
In summary, while tuples and subtype polymorphism can coexist in Java, it's crucial to approach their combination with diligence and a clear understanding of the potential pitfalls. By doing so, developers can leverage the benefits of both concepts while minimizing the risks associated with their intersection.
Understanding the potential pitfalls when using tuples with subtype polymorphism in Java can help developers navigate those complexities effectively, ensuring the reliability and maintainability of their code.