Essential AWS Guide for Beginners: Start Your Cloud Journey
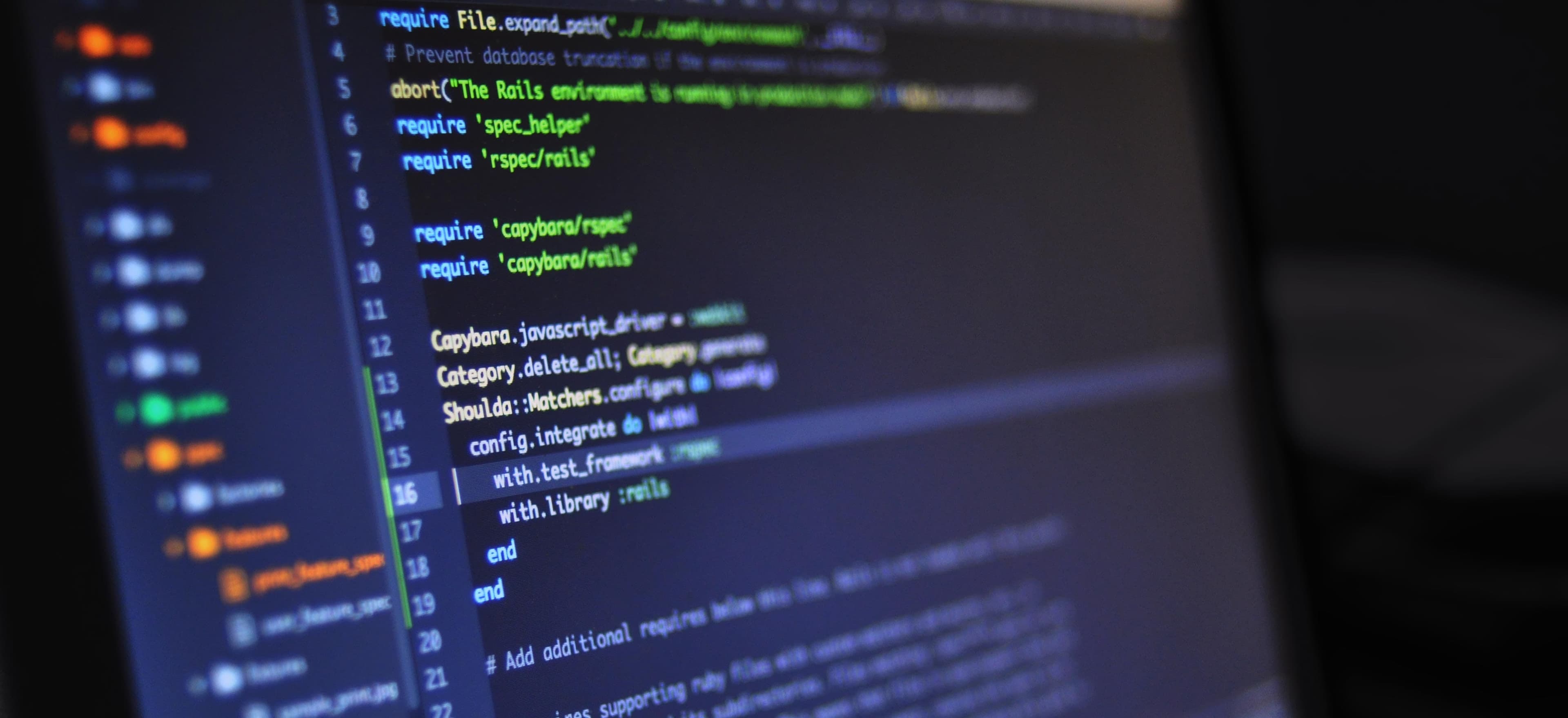
- Published on
A Beginner's Guide to AWS and Java: Getting Started with Cloud Computing
In today's digital age, cloud computing has become an integral part of modern software development. Among the various cloud service providers, Amazon Web Services (AWS) stands out as one of the leading platforms, offering a wide range of services and tools for developers. As a Java developer, leveraging AWS can significantly enhance your application's scalability, reliability, and performance. This guide aims to provide beginners with a foundational understanding of AWS and how to integrate it with Java applications.
Understanding AWS
What is AWS?
Amazon Web Services (AWS) is a secure cloud services platform that offers computing power, database storage, content delivery, and various other functionalities to help businesses scale and grow. AWS provides a comprehensive suite of infrastructure services, including computing power, storage options, and networking, as well as the tools for developer productivity and the ability to deploy and manage applications.
Why Use AWS for Java Development?
AWS offers several advantages for Java developers, including:
- Scalability: AWS provides the ability to scale resources based on application demand, allowing Java applications to handle varying workloads without investing in physical infrastructure.
- Reliability: With AWS's infrastructure, Java applications can achieve high availability and fault tolerance.
- Security: AWS adheres to stringent security standards, allowing Java developers to build secure and compliant applications.
- Broad Ecosystem: AWS offers a broad set of services and tools that can be seamlessly integrated with Java applications, such as AWS Lambda for serverless computing and Amazon RDS for database management.
Getting Started with AWS for Java Development
Setting Up an AWS Account
Before diving into AWS services, you need to create an AWS account. Follow the official guide to create your account, and ensure you have the necessary access credentials for interacting with AWS programmatically.
Using the AWS SDK for Java
The AWS SDK for Java provides a set of APIs for integrating Java applications with various AWS services. You can include the AWS SDK for Java in your project using Maven or Gradle, making it easier to interact with AWS services programmatically.
// Maven
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>s3</artifactId>
<version>2.17.82</version>
</dependency>
// Gradle
implementation 'software.amazon.awssdk:s3:2.17.82'
By including the AWS SDK for Java, you can interact with AWS services such as Amazon S3, Amazon DynamoDB, Amazon EC2, and more directly from your Java code.
Interacting with Amazon S3 Using AWS SDK for Java
Let's consider an example of interacting with Amazon S3, a scalable object storage service offered by AWS, using the AWS SDK for Java.
import software.amazon.awssdk.services.s3.S3Client;
import software.amazon.awssdk.services.s3.model.*;
public class S3Example {
public static void main(String[] args) {
S3Client s3 = S3Client.create();
// Create a bucket
String bucketName = "my-java-bucket";
s3.createBucket(CreateBucketRequest.builder().bucket(bucketName).build());
// Upload an object
String key = "my-object-key";
s3.putObject(PutObjectRequest.builder().bucket(bucketName).key(key)
.build());
// List objects in a bucket
ListObjectsResponse listObjects = s3.listObjects(ListObjectsRequest.builder().bucket(bucketName).build());
listObjects.contents().forEach(obj -> System.out.println(obj.key()));
}
}
In this example, we used the AWS SDK for Java to create a bucket, upload an object, and list objects within a bucket in Amazon S3. This demonstrates how seamlessly Java applications can interact with AWS services using the AWS SDK for Java.
Deploying Java Applications on AWS
AWS offers multiple options for deploying Java applications, such as using Amazon EC2 for virtual servers, AWS Lambda for serverless computing, or Elastic Beanstalk for easy application deployment and scaling. Each approach has its own advantages, and choosing the right deployment option depends on your specific application requirements and architecture.
Key Takeaways
In conclusion, AWS provides a powerful platform for Java developers to build, deploy, and scale their applications. By leveraging the AWS SDK for Java and the myriad of AWS services, Java developers can harness the full potential of cloud computing to deliver robust and scalable solutions. As you continue your journey with AWS and Java, exploring additional services like Amazon RDS for database management and Amazon ECS for containerized applications can further enhance your skill set and the capabilities of your Java applications in the cloud.
Embrace the power of AWS and Java to unlock a world of possibilities in cloud-based application development. Get started today and embark on a rewarding journey into the world of cloud computing with AWS. Happy coding!