Why Your Code Breaks: Liskov Substitution Principle Violations
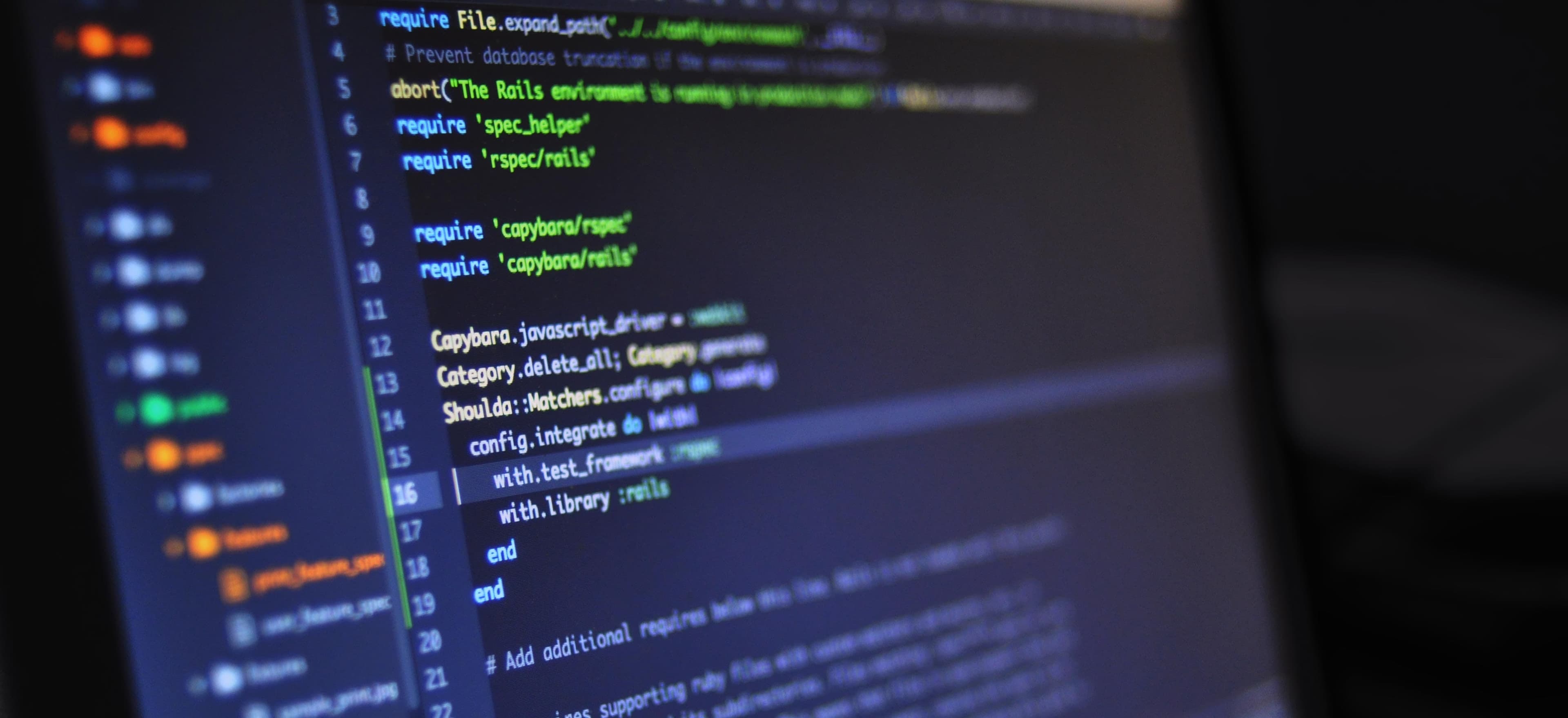
- Published on
Understanding Liskov Substitution Principle Violations in Java
When writing code in Java, developers often encounter situations where their code breaks unexpectedly. One of the key principles in object-oriented programming that can help avoid such issues is the Liskov Substitution Principle (LSP). Understanding LSP violations and how to address them is crucial for writing robust and maintainable Java code.
What is the Liskov Substitution Principle?
The Liskov Substitution Principle, named after Barbara Liskov, is one of the five SOLID principles of object-oriented programming. It states that objects of a superclass should be replaceable with objects of its subclass without affecting the functionality of the program. In simpler terms, if S is a sub-type of T, then objects of type T should be replaceable with objects of type S without altering any desirable properties of the program.
Detecting LSP Violations
LSP violations often occur when a subclass alters the behavior of the superclass in a way that is incompatible with the expected behavior. Let's consider an example:
public class Rectangle {
protected int width;
protected int height;
public void setWidth(int width) {
this.width = width;
}
public void setHeight(int height) {
this.height = height;
}
public int area() {
return width * height;
}
}
public class Square extends Rectangle {
@Override
public void setWidth(int width) {
this.width = width;
this.height = width;
}
@Override
public void setHeight(int height) {
this.height = height;
this.width = height;
}
}
In the above example, the Square
class extends the Rectangle
class, but it violates the LSP since setting the width and height of a square should result in equal sides, whereas setting the width and height of a rectangle should allow different lengths and widths. This violates the expected behavior of the superclass, leading to potential issues when substituting objects.
LSP violations can result in unexpected behavior, especially in scenarios where the code relies on polymorphism and substitutability. It’s crucial to detect and address such violations to ensure the correctness and maintainability of the codebase.
Addressing LSP Violations
To address LSP violations, it’s essential to revisit the design of the classes and ensure that the subclass maintains the expected behavior defined by the superclass. In some cases, it may require redefining the relationships between classes or introducing new abstractions to adhere to the LSP.
In the case of the Rectangle
and Square
example, an appropriate solution would be to create a more abstract shape class, allowing for specific implementations for the Rectangle
and Square
while maintaining the expected behavior for each type.
public abstract class Shape {
public abstract int area();
}
public class Rectangle extends Shape {
// remaining code
}
public class Square extends Shape {
// remaining code
}
By introducing the Shape
superclass and defining specific behaviors for Rectangle
and Square
, we ensure that each subclass honors the LSP, and objects can be replaced without altering the expected program behavior.
Best Practices to Avoid LSP Violations
To prevent LSP violations in your Java code, consider the following best practices:
1. Follow the "is-a" relationship
When creating subclasses, ensure that they adhere to the "is-a" relationship with the superclass. This means that a subclass should be a more specialized version of its superclass without altering the fundamental behavior.
2. Use abstract classes and interfaces effectively
Utilize abstract classes and interfaces to define common behavior and characteristics while allowing subclasses to provide specific implementations. This promotes adherence to LSP by defining clear contracts for subclasses to follow.
3. Test substitutability
Regularly test substitutability of objects by substituting instances of subclasses in places where instances of the superclass are expected. This helps ensure that the LSP is not violated during the evolution of the codebase.
4. Document expected behaviors
Clearly document the expected behaviors and contracts of classes and their methods, especially when designing reusable components or libraries. This helps developers understand the intended behavior, reducing the likelihood of unintentional LSP violations.
Final Thoughts
Understanding and upholding the Liskov Substitution Principle is essential for writing maintainable and robust Java code. By detecting and addressing LSP violations, adhering to best practices, and continually testing code substitutability, developers can ensure that their codebase remains resilient to unexpected issues stemming from violations of LSP.
In the context of Java development, LSP violations can lead to subtle bugs and unexpected behavior, making it imperative for developers to grasp the principle and its implications thoroughly. By incorporating the Liskov Substitution Principle into their coding practices, developers can build more reliable and maintainable Java applications.
For additional insights into SOLID principles, including the Liskov Substitution Principle, and its importance in writing high-quality software, consider exploring the comprehensive resources available on Oracle's Java documentation.