Optimizing Java Application Performance with Buffer Pool Management
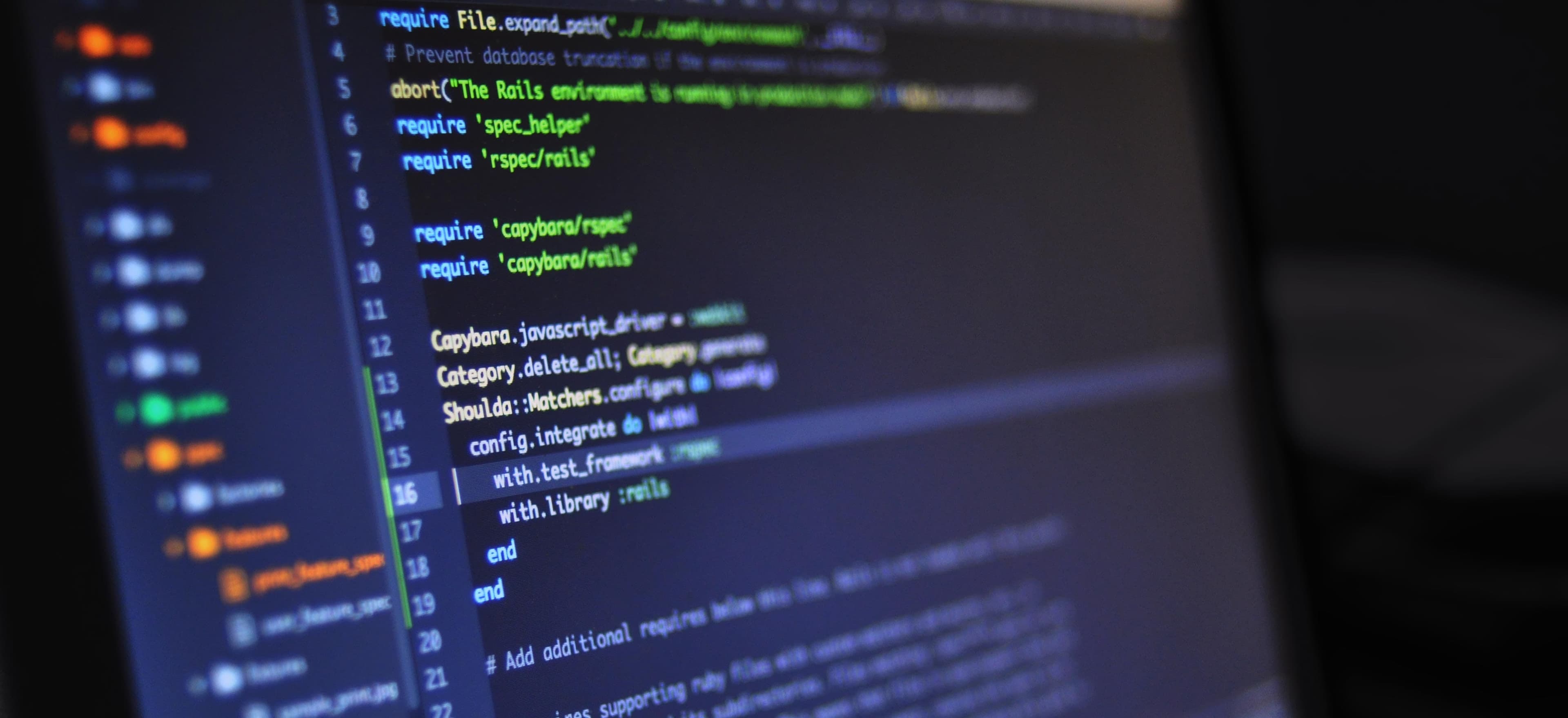
- Published on
Optimizing Java Application Performance with Buffer Pool Management
In today's world, where digital expectations are higher than ever, optimizing the performance of Java applications has become crucial. Java's automatic memory management relieves developers from manual memory management concerns, but efficiently managing the buffer pool can significantly enhance the performance of Java applications, especially for I/O intensive operations.
In this article, we'll delve into buffer pool management in Java, explore its significance, and discuss strategies for optimizing Java application performance through effective buffer pool management.
Understanding Buffer Pool Management
Before diving into optimization techniques, let's understand what buffer pool management is and why it's important for Java applications.
What is a Buffer Pool?
A buffer pool is a block of memory within the Java Virtual Machine (JVM) reserved for caching data retrieved from external sources, such as disks or network connections. When a Java application performs I/O operations, it reads data from these sources into memory buffers, known as the buffer pool, for faster access. Proper management of the buffer pool is crucial for optimal I/O performance and efficient memory utilization.
Significance of Buffer Pool Management
Efficient buffer pool management is critical for the following reasons:
-
I/O Performance: Well-optimized buffer pool management can significantly reduce the I/O wait times by minimizing disk or network reads, thereby improving application performance.
-
Memory Utilization: Effective management ensures optimal utilization of memory, preventing issues such as excessive garbage collection and out-of-memory errors.
-
Scalability: Proper buffer pool management supports the scalability of Java applications, enabling them to handle increasing workloads efficiently.
Optimizing Java Application Performance through Buffer Pool Management
Now that we understand the importance of buffer pool management, let's explore some strategies to optimize Java application performance through effective buffer pool management.
1. Using Direct Memory for I/O Operations
By default, Java performs I/O operations using the JVM's heap memory, which involves an additional memory copy from the buffer pool to the native memory. However, using direct memory (off-heap) for I/O operations eliminates this additional copy, thereby reducing memory overhead and improving I/O performance.
// Sample code using direct memory for I/O operations
ByteBuffer buffer = ByteBuffer.allocateDirect(1024); // Allocate direct (off-heap) memory
// Perform I/O operations using the direct memory buffer
2. Adjusting Buffer Pool Size
The default size of the buffer pool in Java may not be optimal for all applications. By adjusting the buffer pool size based on the specific I/O requirements and available memory, you can prevent excessive memory allocation or underutilization of the buffer pool, leading to improved performance.
// Sample code for adjusting buffer pool size
int bufferSize = 8192; // Custom buffer size based on specific requirements
ByteBuffer buffer = ByteBuffer.allocate(bufferSize); // Allocate buffer with the custom size
3. Implementing LRU (Least Recently Used) Caching
Implementing a custom LRU cache for the buffer pool can help in efficiently managing memory by discarding the least recently used data when the buffer pool reaches its limits. This can prevent unnecessary memory consumption and ensure that the most relevant data is retained in the buffer pool for quicker access.
// Sample code for implementing LRU caching for buffer pool
import java.util.LinkedHashMap;
import java.util.Map;
int maxEntries = 100; // Maximum entries for the LRU cache
Map<String, ByteBuffer> bufferPool = new LinkedHashMap<>(maxEntries, 0.75f, true) {
protected boolean removeEldestEntry(Map.Entry<String, ByteBuffer> eldest) {
return size() > maxEntries;
}
};
4. Utilizing Asynchronous I/O
Java provides support for asynchronous I/O operations through the AsynchronousFileChannel
class, which enables non-blocking I/O and efficient utilization of the buffer pool, especially for high-throughput applications.
// Sample code for asynchronous I/O using AsynchronousFileChannel
AsynchronousFileChannel fileChannel = AsynchronousFileChannel.open(Paths.get("file.txt"), StandardOpenOption.READ);
ByteBuffer buffer = ByteBuffer.allocate(1024); // Allocate buffer for asynchronous I/O
fileChannel.read(buffer, 0, null, new CompletionHandler<Integer, Object>() {
@Override
public void completed(Integer result, Object attachment) {
// Handle completion of asynchronous read operation
}
@Override
public void failed(Throwable exc, Object attachment) {
// Handle failure of asynchronous read operation
}
});
5. Monitoring and Tuning the Buffer Pool
Monitoring the buffer pool utilization and tuning its parameters based on runtime metrics can significantly impact the overall performance of Java applications. Tools like Java Mission Control and VisualVM provide insights into memory usage and can aid in fine-tuning the buffer pool for optimal performance.
// Sample code for monitoring buffer pool utilization (using JMX)
MBeanServer mbs = ManagementFactory.getPlatformMBeanServer();
ObjectName name = ObjectName.getInstance("java.lang:type=BufferPool,name=direct");
MemoryPoolMXBean pool = ManagementFactory.newPlatformMXBeanProxy(mbs, name, MemoryPoolMXBean.class);
long usedMemory = pool.getUsage().getUsed();
// Perform actions based on buffer pool utilization metrics
Final Considerations
Java applications often rely on efficient I/O operations for optimal performance. By focusing on effective buffer pool management, developers can significantly enhance the I/O performance and overall scalability of their applications. Implementing strategies such as using direct memory for I/O operations, adjusting buffer pool size, implementing LRU caching, utilizing asynchronous I/O, and monitoring/tuning the buffer pool can lead to substantial improvements in application performance.
Optimizing buffer pool management not only boosts the performance of existing applications but also lays a strong foundation for handling future scalability and performance challenges. By understanding the significance of buffer pool management and implementing the discussed optimization strategies, developers can unleash the full potential of their Java applications, providing an enhanced user experience and greater efficiency.
In conclusion, efficient buffer pool management is a key aspect of optimizing Java application performance, and by incorporating the discussed strategies, developers can elevate the I/O performance and scalability of their applications, meeting the demands of today's dynamic digital landscape.
Remember, optimizing buffer pool management is just one piece of the performance puzzle, and combining it with other best practices and performance tuning techniques can further elevate the overall efficiency of Java applications.
So, start optimizing your Java application's buffer pool management today and unleash its full potential!
Checkout our other articles