Mastering Java: Tackling DecimalFormat Precision Issues
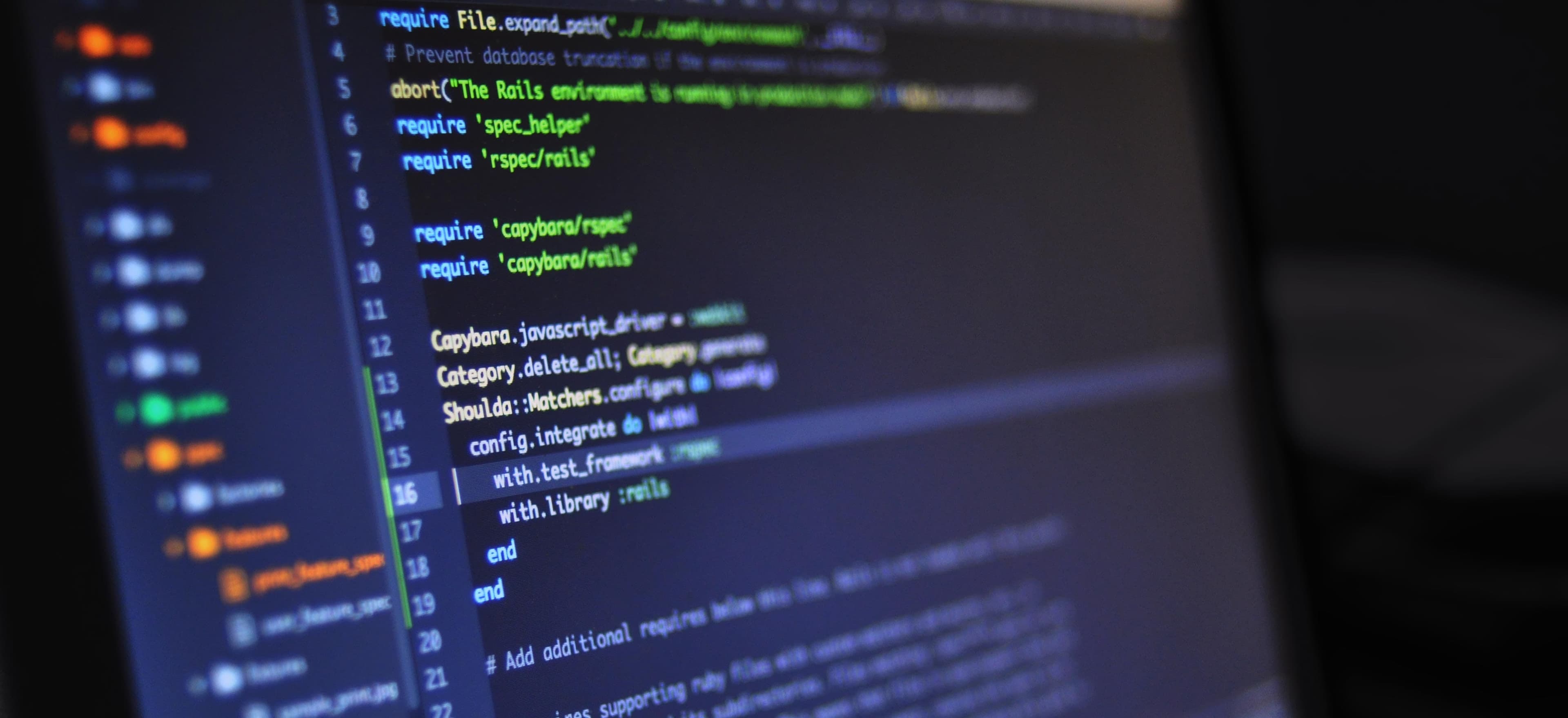
- Published on
Mastering Java: Tackling DecimalFormat Precision Issues
When working with decimal numbers in Java, precision issues can be a common headache for developers. This is especially true when dealing with financial applications or any scenario where accuracy is crucial. In this blog post, we'll dive into the world of DecimalFormat
in Java and explore how to tackle precision issues effectively.
Understanding DecimalFormat
DecimalFormat
is a class in Java that allows us to format decimal numbers according to a specific pattern. It provides numerous options for controlling the display of numerical data, such as setting the number of decimal places, specifying the grouping size, and defining how to handle rounding.
Let's start by looking at a basic example of using DecimalFormat
to format a decimal number:
import java.text.DecimalFormat;
public class DecimalFormatExample {
public static void main(String[] args) {
double number = 12345.6789;
DecimalFormat df = new DecimalFormat("#,##0.00");
String formatted = df.format(number);
System.out.println(formatted);
}
}
In this example, we create a DecimalFormat
instance with the pattern #,##0.00
, which specifies that the number should be formatted with thousands separators and rounded to two decimal places. When we call df.format(number)
, the output will be 12,345.68
.
Dealing with Precision Issues
One of the common challenges when working with decimal formatting in Java is precision loss due to the internal binary representation of floating-point numbers. Consider the following example:
double amount = 0.1 + 0.2;
DecimalFormat df = new DecimalFormat("#,##0.00");
String formatted = df.format(amount);
System.out.println(formatted);
The expected output would be 0.30
, but due to precision loss, the actual output is 0.30000000000000004
. This discrepancy can cause significant issues in applications dealing with financial calculations.
To address precision issues like these, one approach is to use BigDecimal
for accurate decimal arithmetic. Here's an example of how we can use BigDecimal
in conjunction with DecimalFormat
to handle precision effectively:
import java.math.BigDecimal;
import java.text.DecimalFormat;
public class BigDecimalExample {
public static void main(String[] args) {
BigDecimal amount = new BigDecimal("0.1").add(new BigDecimal("0.2"));
DecimalFormat df = new DecimalFormat("#,##0.00");
String formatted = df.format(amount);
System.out.println(formatted);
}
}
By using BigDecimal
, we ensure precise decimal arithmetic, and when we format the result using DecimalFormat
, we get the expected output of 0.30
.
Rounding Strategies
Another aspect of precision management in DecimalFormat
is rounding strategies. By default, DecimalFormat
uses the "round half up" strategy, but we can customize the rounding behavior as per our requirements.
Let's consider a scenario where we want to round a number to a specific number of decimal places using different rounding strategies:
import java.text.DecimalFormat;
import java.math.RoundingMode;
public class RoundingExample {
public static void main(String[] args) {
double number = 123.45678;
DecimalFormat df = new DecimalFormat("#,##0.00");
df.setRoundingMode(RoundingMode.HALF_UP);
String roundedUp = df.format(number);
df.setRoundingMode(RoundingMode.DOWN);
String roundedDown = df.format(number);
System.out.println("Rounded up: " + roundedUp);
System.out.println("Rounded down: " + roundedDown);
}
}
In this example, we demonstrate rounding the number
to two decimal places using both HALF_UP
and DOWN
rounding modes. This allows us to control how the number is rounded and formatted based on our specific needs.
A Final Look
In this blog post, we explored the intricacies of handling precision issues with DecimalFormat
in Java. We discussed the challenges of precision loss in floating-point arithmetic and how to mitigate them using BigDecimal
. Additionally, we delved into customizing rounding strategies to fine-tune the formatting of decimal numbers.
By mastering these techniques, Java developers can ensure accurate and precise decimal formatting, particularly in applications where precision is paramount.
Remember, precise decimal formatting not only ensures accuracy but also instills trust in your application's numerical representations - a crucial aspect of quality software engineering.
To deepen your understanding of number formatting in Java, check out the official Java Documentation and Java NumberFormatting Guide.
Keep coding with precision!
Checkout our other articles