Simplifying Scala: Type Classes & Implicits in Design Patterns
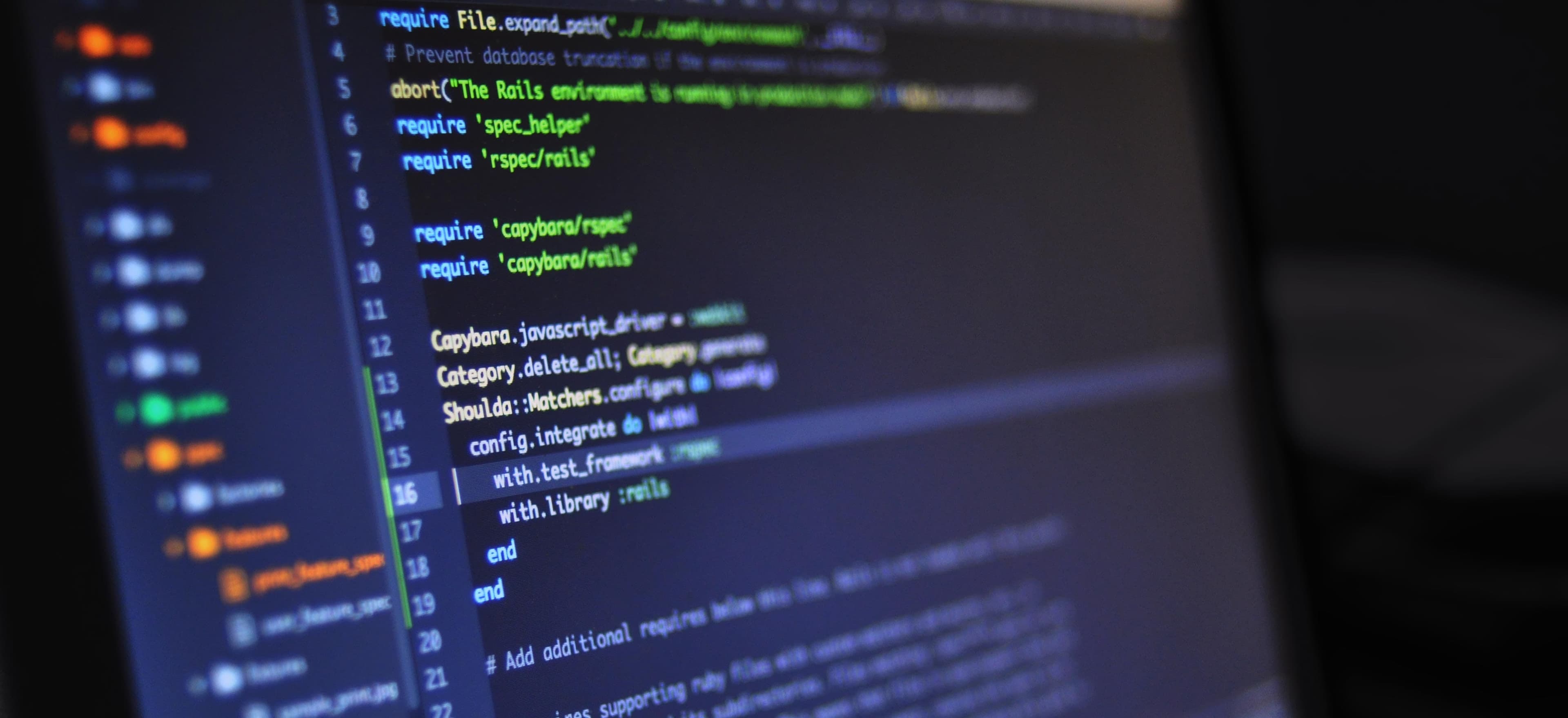
- Published on
Author: [Your Name]
As programmers, we are constantly exploring ways to write robust code that is maintainable and easily understandable. Design patterns play a crucial role in achieving these goals, and in the world of functional programming, Scala offers a powerful feature set to implement these design patterns. One such feature is the use of type classes and implicits. In this article, we will dive into the concept of type classes and implicits in Scala, and explore how they can be leveraged to simplify and enhance the design patterns in your codebase.
Understanding Type Classes
In the context of Scala, type classes are a powerful tool for implementing ad-hoc polymorphism – a cornerstone of functional programming. At its core, a type class defines a set of behaviors or functionality that can be applied to a particular type. Unlike traditional polymorphism using inheritance and subtyping, type classes allow you to add new behaviors to existing types without modifying their definitions.
Let’s illustrate this with a simple example. Consider a scenario where you have different types such as Int
, String
, and Double
, and you want to define a common serialize
behavior for each of these types. Using type classes, you can define a Serializable
type class and then provide instances for Int
, String
, and Double
to implement the serialize
behavior for each type.
trait Serializable[T] {
def serialize(value: T): String
}
object SerializableInstances {
implicit val intSerializable: Serializable[Int] = (value: Int) => value.toString
implicit val stringSerializable: Serializable[String] = (value: String) => value
implicit val doubleSerializable: Serializable[Double] = (value: Double) => value.toString
}
In the example above, we define a type class Serializable
with a single method serialize
. We then provide implicit instances of this type class for Int
, String
, and Double
. This allows us to use the serialize
method on instances of these types without modifying their original definitions.
Leveraging Implicits to Enable Type Class Resolution
Implicits play a crucial role in enabling type class instances to be automatically resolved and provided wherever they are needed. By marking the instances of type classes as implicit
, Scala's compiler can automatically look up and provide the necessary instances at compile time.
Let’s revisit the Serializable
type class example to see how implicits are used to resolve the instances for different types:
object Serialization {
def serialize[T](value: T)(implicit serializer: Serializable[T]): String = {
serializer.serialize(value)
}
}
In the Serialization
object, the serialize
method takes a value of type T
and an implicit parameter of type Serializable[T]
. When invoking the serialize
method, Scala's compiler will look for the appropriate implicit instance of Serializable
for the given type T
and inject it into the method call.
Simplifying Design Patterns with Type Classes and Implicits
Now that we have a solid understanding of type classes and implicits, let’s examine how these concepts can simplify the implementation of design patterns in Scala. We will explore two popular design patterns – the Strategy Pattern and the Factory Pattern – and demonstrate how type classes and implicits can be leveraged to streamline their implementation.
Strategy Pattern
The Strategy Pattern is a behavioral design pattern that enables defining a family of algorithms, encapsulating each one, and making them interchangeable. By using type classes and implicits, we can abstract the strategy implementations and make the usage concise and elegant.
Let's consider a scenario where we want to implement a text formatter that supports different formatting strategies such as UpperCase
, LowerCase
, and CamelCase
. We can define a type class TextFormatter
and provide instances for each formatting strategy using implicits:
trait TextFormatter {
def format(text: String): String
}
object TextFormatters {
implicit object UpperCaseFormatter extends TextFormatter {
override def format(text: String): String = text.toUpperCase
}
implicit object LowerCaseFormatter extends TextFormatter {
override def format(text: String): String = text.toLowerCase
}
implicit object CamelCaseFormatter extends TextFormatter {
override def format(text: String): String = text.split(" ").map(_.capitalize).mkString
}
}
With the type class instances defined, we can now use the strategy pattern by simply passing the required formatting strategy as an implicit parameter to the formatter:
object TextFormatterUtil {
def formatText(text: String)(implicit formatter: TextFormatter): String = {
formatter.format(text)
}
}
By doing so, the strategy for formatting the text is automatically resolved based on the implicit parameter, making the usage of different formatting strategies seamless and extensible.
Factory Pattern
The Factory Pattern is another widely used design pattern that provides an interface for creating objects, allowing the subclasses to alter the type of objects that will be created. Leveraging type classes and implicits, we can enhance the flexibility and maintainability of factory implementations.
Consider a scenario where we need to create different shapes such as Circle
, Rectangle
, and Triangle
using a factory. We can define a type class Shape
and provide instances for each shape using implicits:
trait Shape {
def draw(): Unit
}
object Shapes {
implicit object CircleShape extends Shape {
override def draw(): Unit = println("Drawing Circle")
}
implicit object RectangleShape extends Shape {
override def draw(): Unit = println("Drawing Rectangle")
}
implicit object TriangleShape extends Shape {
override def draw(): Unit = println("Drawing Triangle")
}
}
With the type class instances in place, we can create a factory that leverages implicits to dynamically provide the required shape based on the implicit parameter:
object ShapeFactory {
def createShape()(implicit shape: Shape): Unit = {
shape.draw()
}
}
Through the use of implicits, the factory method can automatically resolve the appropriate shape based on the implicit parameter, making the process of creating different shapes seamless and extensible.
Final Thoughts
In this article, we have explored the powerful combination of type classes and implicits in Scala and how they can be used to simplify the implementation of design patterns, such as the Strategy Pattern and the Factory Pattern. By leveraging type classes and implicits, we can achieve a more concise, modular, and extensible codebase, ultimately leading to better maintainability and scalability of our applications. Understanding these concepts and incorporating them into your Scala projects can greatly enhance your ability to write elegant and maintainable code.
In conclusion, type classes and implicits are essential tools in the functional programmer’s arsenal, enabling the implementation of powerful design patterns with ease and elegance. Embracing these concepts can open up new avenues for writing expressive, modular, and reusable code in Scala, ultimately leading to more resilient and maintainable software systems.
We hope this article has provided you with valuable insights into the use of type classes and implicits in simplifying design patterns, and we encourage you to explore and experiment with these concepts in your own Scala projects for a more robust and elegant codebase.
Happy coding!
Disclaimer: This blog post is for informational purposes only and does not constitute professional advice. Keep in mind that the usage of the provided code examples and patterns should be carefully evaluated and adapted to specific project requirements and best practices. Always strive to write clear, maintainable, and efficient code, and review the latest documentation and community guidelines for the technologies you are working with.
Checkout our other articles