Balancing Act: Choosing Between Microservices and Monoliths
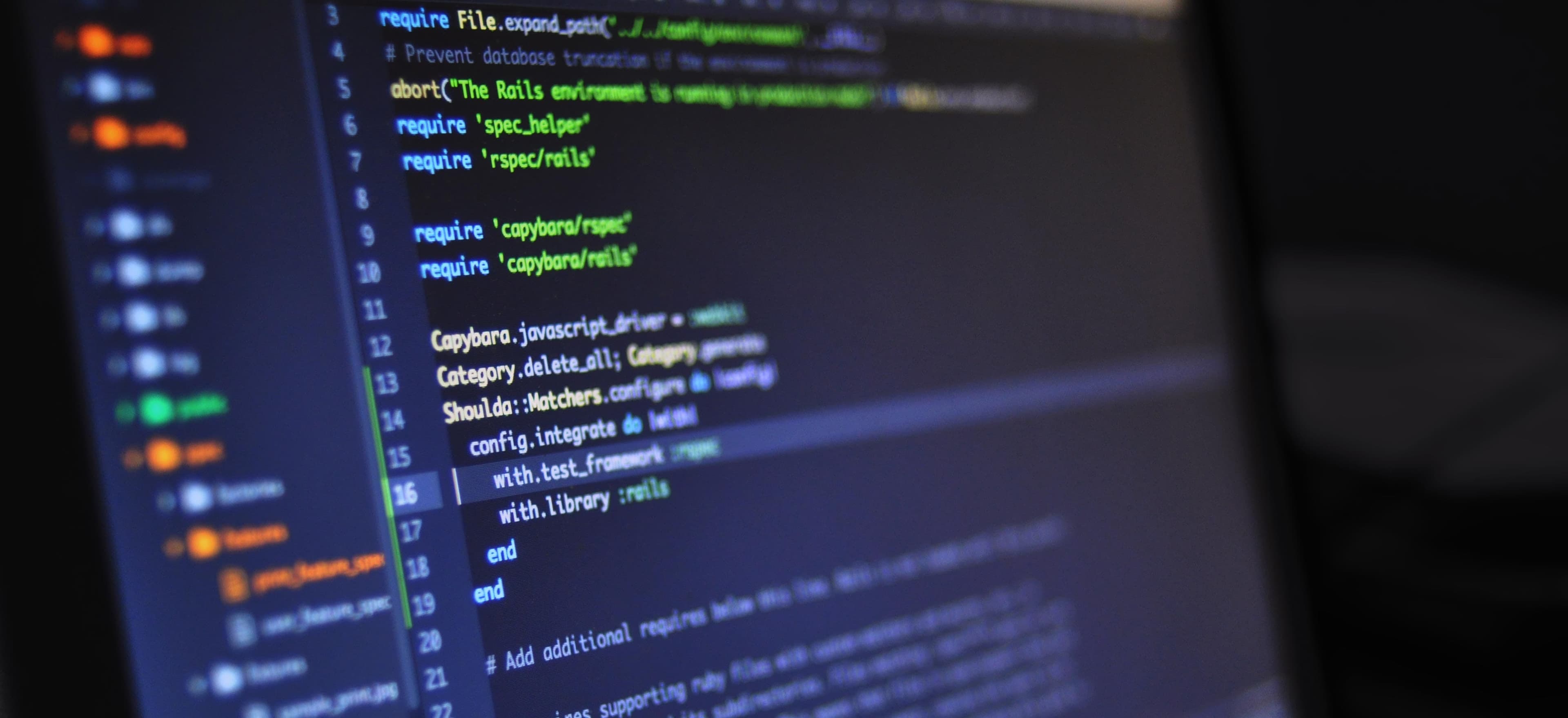
- Published on
Balancing Act: Choosing Between Microservices and Monoliths
In the realm of Java software development, the decision between using a monolithic architecture versus a microservices architecture is often a critical one. Each approach has its own set of advantages and disadvantages, and understanding the nuances of these architectures is essential for making an informed decision.
Understanding Monolithic Architecture
The Monolith Mindset
In a monolithic architecture, all the components of a software application are interconnected and interdependent. This means that the entire application is built as a single unit, with its components tightly coupled together. While this can make the initial development process more straightforward, it can also lead to challenges when it comes to scalability, maintainability, and resilience.
Pros and Cons
Pros
- Simplicity: Monolithic architectures are simpler to develop and test due to their centralized nature.
- Performance: In some cases, monolithic applications can have better performance due to reduced network communication between components.
Cons
- Scalability: Scaling a monolithic application can be challenging as the entire application needs to be replicated to handle increased load.
- Maintenance: As the application grows, it becomes increasingly difficult to maintain and evolve, often leading to a tangled and difficult-to-maintain codebase.
Embracing Microservices Architecture
The Microservices Paradigm
Microservices architecture breaks down an application into a set of loosely coupled services, each running as an independent process and communicating with lightweight mechanisms, often HTTP or messaging protocols. This architectural style promotes the idea of developing a suite of small services, each focused on a specific business capability.
Pros and Cons
Pros
- Scalability: Each service can be scaled independently, allowing for greater flexibility in handling varying levels of demand.
- Maintainability: Services can be maintained, updated, and replaced without affecting the entire application, leading to a more agile development process.
- Technology Diversity: Different services can be implemented using different technologies, enabling teams to select the best tool for each specific task.
Cons
- Complexity: Managing and coordinating a large number of services can result in increased complexity, especially regarding deployment and orchestration.
- Testing: Testing a distributed system introduces new challenges in terms of integration testing and managing the testing environment.
Choosing the Right Path
Factors to Consider
When deciding between a monolithic or microservices architecture, there are several key factors to consider:
-
Size of the Project: For small to medium-sized projects with limited complexity, a monolithic architecture may be more suitable, as the overhead of microservices may outweigh the benefits.
-
Scalability Requirements: If the application requires the ability to scale specific features or components independently, microservices might be the more appropriate choice.
-
Team Structure: The team's expertise and the organization's infrastructure will play a significant role in determining which architecture is more suitable.
-
Future Growth: Consider the long-term vision for the project. Will it grow in complexity and scale? Anticipating future needs is essential in making an informed decision.
Case in Point
Let's consider a hypothetical scenario to illustrate the nuances of this decision-making process. Suppose a startup is developing a new e-commerce platform.
Monolithic Approach: If the team aims to build and iterate quickly, focusing on a single, well-defined product, a monolithic architecture might be a pragmatic choice. It allows the team to minimize operational overhead and concentrate on delivering core functionality.
Microservices Approach: On the other hand, if the team anticipates rapid growth and requirements for flexibility and resilience, a microservices architecture could provide the necessary foundation. The ability to independently scale the ordering, inventory, and payment services, among others, will be crucial for handling potential spikes in demand.
In this example, the decision would hinge on the anticipated business trajectory, resource considerations, and technical capabilities of the team.
Java and Microservices: A Natural Fit
When it comes to implementing microservices in Java, several frameworks and tools cater to this architectural style:
-
Spring Boot: With its emphasis on rapid development and microservices, Spring Boot provides a powerful and flexible framework for building and deploying Java-based microservices.
-
MicroProfile: An open forum to optimize Enterprise Java for a microservices architecture, MicroProfile offers a set of APIs for building microservices-based applications.
-
Kubernetes: While not specific to Java, Kubernetes plays a crucial role in orchestrating and managing containers, making it an essential component in deploying and managing Java-based microservices at scale.
Practical Implementation
Let's delve into a practical example to highlight the contrast between a monolithic and microservices approach using Java.
Monolith: Building a Simple E-Commerce System
public class ShoppingCartService {
public ShoppingCart getShoppingCart(int userId) {
// Logic to fetch and return the shopping cart for the user
}
public void checkout(ShoppingCart cart) {
// Logic to process the checkout and place the order
}
}
In a monolithic approach, the shopping cart and checkout functionality are tightly coupled within a single service. While this may simplify the initial development, scaling and maintaining this service as the system grows could become challenging.
Microservices: Decomposing the E-Commerce System
// Shopping Cart Service
@RestController
public class ShoppingCartController {
@GetMapping("/users/{userId}/cart")
public ShoppingCart getShoppingCart(@PathVariable int userId) {
// Logic to fetch and return the shopping cart for the user
}
}
// Checkout Service
@RestController
public class CheckoutController {
@PostMapping("/checkout")
public void checkout(@RequestBody ShoppingCart cart) {
// Logic to process the checkout and place the order
}
}
In a microservices approach, the shopping cart and checkout functionality are separated into distinct services. This allows for independent scaling, maintenance, and deployment of each service, paving the way for future growth and flexibility.
The Last Word
In the realm of Java software development, the decision between a monolithic and microservices architecture requires a careful evaluation of various factors. While monolithic architectures offer simplicity and performance benefits, microservices architectures provide scalability, maintainability, and flexibility advantages.
Ultimately, the choice between the two architectures depends on the specific needs, goals, and context of the project. Leveraging the right architecture for the right scenario can lay the foundation for a robust and future-proof system.
As the landscape of technology continues to evolve, the importance of making informed architectural decisions has never been more crucial. Whether it's a monolith or microservices, the key lies in understanding the trade-offs and selecting the approach that aligns best with the project's requirements.
Checkout our other articles