Java's Missed Chance: Why It Needs Kotlin's Top 10 Features
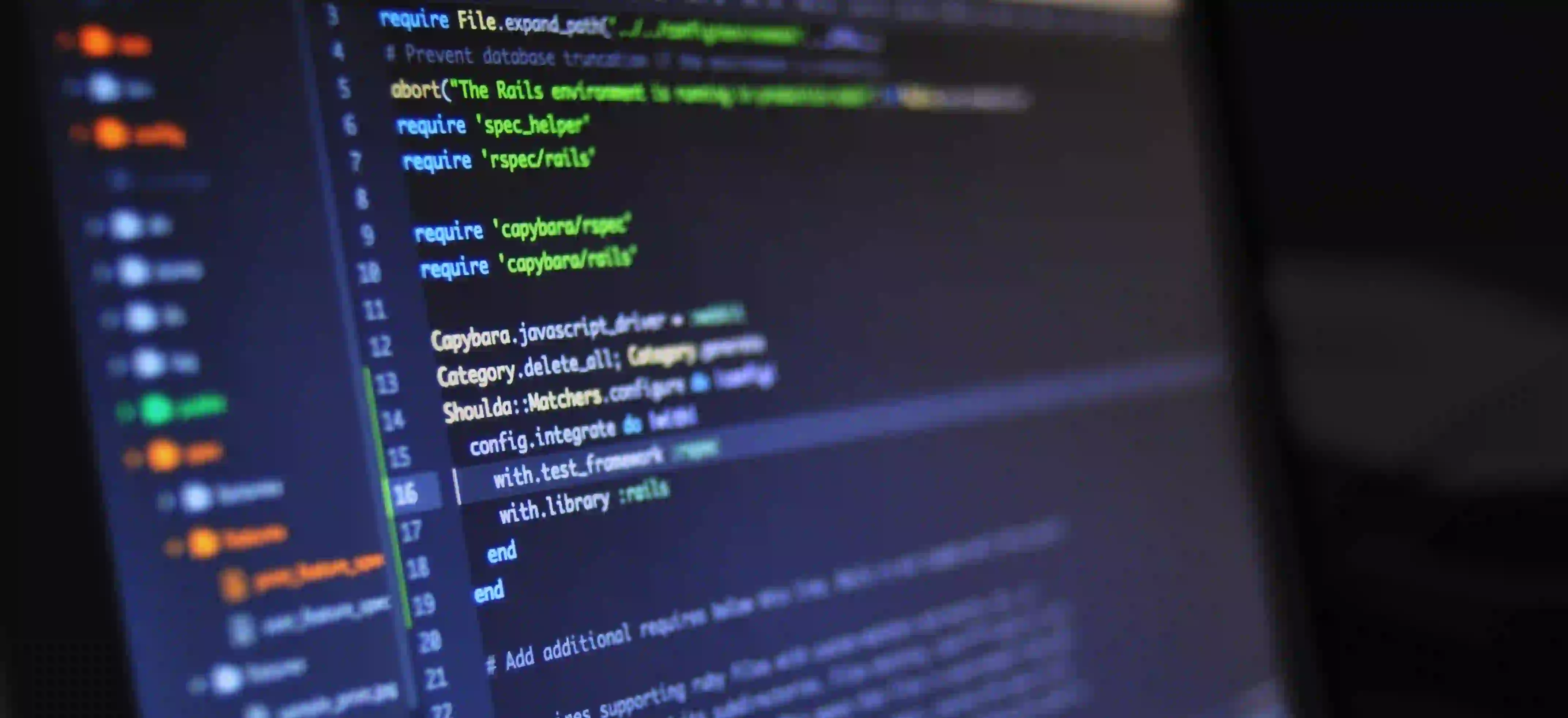
Java's Missed Chance: Why It Needs Kotlin's Top 10 Features
When it comes to programming languages, Java has long been a dominant force in the world of software development. However, with the emergence of Kotlin as a modern alternative, Java's shortcomings are becoming more apparent. In this article, we'll explore 10 key features of Kotlin that Java lacks and why incorporating these features is essential for Java to remain competitive in today's fast-paced development environment.
1. Null Safety
One of the most common sources of bugs in Java is null pointer exceptions. Kotlin addresses this issue by making null safety a first-class citizen in the language. By introducing nullable and non-nullable types, Kotlin ensures that null pointer exceptions are caught at compile time rather than runtime. This not only makes code safer but also reduces the need for defensive programming.
// Java
String value = null;
if (value != null) {
int length = value.length(); // May throw NullPointerException
}
// Kotlin
val value: String? = null
val length = value?.length // No NullPointerException
2. Extension Functions
Kotlin allows developers to add new functions to existing classes without modifying their source code. This feature, known as extension functions, promotes a more modular and readable codebase. In Java, achieving similar functionality often requires the use of utility classes or static methods, leading to a less elegant and more verbose solution.
// Java
StringUtils.capitalize("hello");
// Kotlin
"hello".capitalize()
3. Data Classes
Data classes in Kotlin are concise and focused solely on holding data. They automatically generate equals()
, hashCode()
, and toString()
implementations, making them ideal for representing simple data types. In Java, achieving the same level of conciseness and functionality requires writing repetitive boilerplate code.
// Java
public class Person {
private String name;
private int age;
// Getters, setters, equals(), hashCode(), and toString() - tedious to write
}
// Kotlin
data class Person(val name: String, val age: Int)
4. Coroutines
Kotlin introduces coroutines as a more efficient and readable way to write asynchronous code. With Java's reliance on callbacks and complicated threading models, managing asynchronous operations can quickly become convoluted. Coroutines make asynchronous code more readable and maintainable by allowing developers to write sequential code that suspends and resumes execution as needed.
// Java
CompletableFuture.supplyAsync(() -> fetchData())
.thenAcceptAsync(data -> processData(data));
// Kotlin
val data = withContext(Dispatchers.IO) { fetchData() }
processData(data)
5. Smart Casts
Kotlin's smart casts eliminate the need for explicit type casting by intelligently recognizing type checks and casts within conditional blocks. This feature not only reduces redundancy but also enhances code readability by leveraging the compiler's knowledge to simplify the code.
// Java
if (shape instanceof Circle) {
Circle circle = (Circle) shape;
circle.calculateArea();
}
// Kotlin
if (shape is Circle) {
shape.calculateArea()
}
6. Immutability by Default
In Kotlin, variables are immutable by default, promoting a more functional programming style. This encourages developers to write code that is less error-prone and easier to reason about. While Java supports immutability through the use of the final
keyword, Kotlin's default approach simplifies the process and reduces boilerplate code.
// Java
final List<String> colors = Arrays.asList("red", "green", "blue");
// Kotlin
val colors = listOf("red", "green", "blue")
7. Named Arguments
Kotlin allows developers to specify argument names when calling functions, providing better clarity and readability, especially when dealing with functions that have many parameters. In Java, the order of arguments is crucial, and methods with several parameters can lead to confusion.
// Java
drawRectangle(10, 20, 30, 40);
// Kotlin
drawRectangle(width = 10, height = 20, x = 30, y = 40)
8. Property Delegation
Kotlin's property delegation allows for the effective reuse of property accessors and modifiers. This feature simplifies code maintenance and promotes reusability, reducing the need for boilerplate code in Java.
val lazyValue: String by lazy {
// Initialization code
"initialized"
}
9. Ranges
Kotlin's range expressions provide a concise and readable way to define ranges of values, making code more expressive and easier to understand, especially when working with collections and loops. Java lacks built-in range support, resulting in more verbose range definitions and iterations.
// Java
for (int i = 1; i <= 10; i++) {
// Do something
}
// Kotlin
for (i in 1..10) {
// Do something
}
10. Type Inference
In Kotlin, type inference allows developers to omit explicit type declarations when the compiler can infer the type from the context. This reduces verbosity and improves readability without sacrificing type safety. While Java has made strides in enhancing type inference in recent versions, Kotlin's approach remains more concise and intuitive.
// Java
Map<String, List<String>> map = new HashMap<>();
// Kotlin
val map = HashMap<String, List<String>>()
In conclusion, Kotlin's top 10 features address many of the pain points experienced by Java developers, offering a more modern and concise language with enhanced safety and readability. While Java continues to evolve, incorporating these features would undoubtedly bolster its position in the ever-changing landscape of software development.
To delve deeper into the world of Java and Kotlin, check out these insightful resources on Kotlin's official website and the Java Magazine.