Avoiding Burnout: 5 Key Strategies for Wise Development
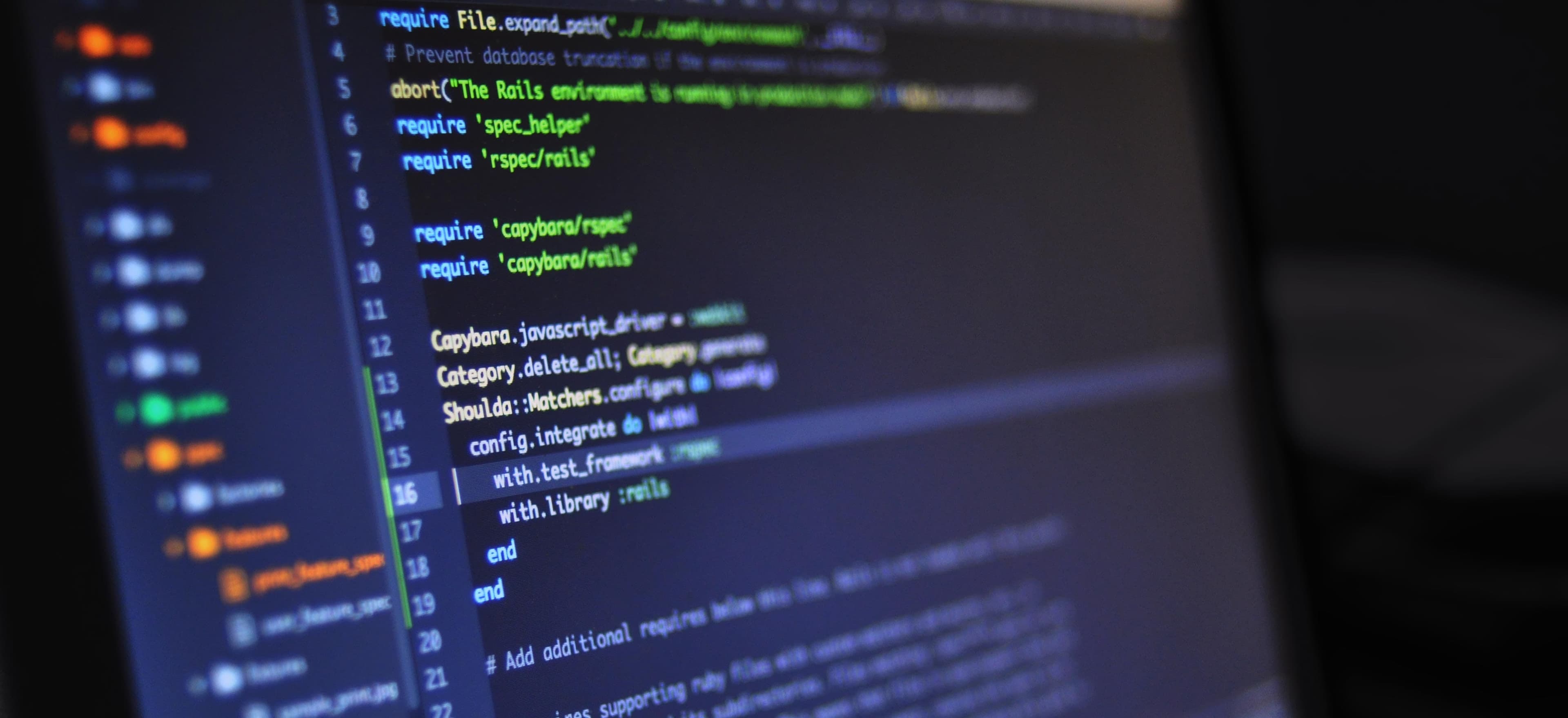
- Published on
Avoiding Burnout: 5 Key Strategies for Wise Development
In the competitive world of software development, burnout is a common issue that many developers face due to the high-pressure environment, long hours, and constant need to stay updated with the latest technologies. As a Java developer, it's crucial to find ways to prevent burnout and maintain a healthy work-life balance. In this article, we'll explore five key strategies for wise development that can help Java developers avoid burnout and stay productive.
1. Prioritize Code Quality Over Quantity
It's easy to fall into the trap of writing as much code as possible to meet deadlines or impress colleagues. However, prioritizing code quality over quantity is essential for long-term success. Writing clean, maintainable code not only reduces the chances of bugs and errors but also makes it easier for you and your team to understand and modify the code in the future.
// Bad practice: Prioritizing code quantity
for (int i = 0; i < 1000; i++) {
// Write complex logic here
}
// Good practice: Prioritizing code quality
public void fetchDataAndUpdateUI() {
// Implement clear and concise logic here
}
When you focus on writing high-quality code, you'll spend less time fixing bugs and more time delivering value to your project. Tools like Checkstyle and SonarQube can help you maintain code quality standards and identify areas for improvement.
2. Embrace Test-Driven Development (TDD)
Test-Driven Development (TDD) is a software development approach where you write tests before you write the corresponding code. Embracing TDD not only leads to better code coverage and fewer bugs but also promotes a more structured and deliberate approach to programming.
// TDD approach
@Test
public void shouldReturnSumOfTwoNumbers() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertEquals(5, result);
}
By following TDD practices, you can write code with more confidence, knowing that it's backed by a comprehensive suite of tests. These tests act as a safety net, allowing you to refactor and modify code without the fear of introducing unexpected issues.
3. Practice Regular Refactoring
Refactoring is the process of restructuring existing code to improve its readability, maintainability, and performance, without changing its external behavior. As a Java developer, it's crucial to make refactoring a regular part of your development process.
// Before refactoring
public void calculateTotalPrice(List<Product> products) {
double totalPrice = 0;
for (Product product : products) {
totalPrice += product.getPrice();
}
return totalPrice;
}
// After refactoring
public double calculateTotalPrice(List<Product> products) {
return products.stream().mapToDouble(Product::getPrice).sum();
}
By consistently refactoring your code, you can prevent technical debt from accumulating and ensure that your codebase remains clean and efficient, leading to a more enjoyable development experience.
4. Cultivate a Healthy Work-Life Balance
In the fast-paced world of software development, it's easy to get caught up in long work hours and tight deadlines. However, maintaining a healthy work-life balance is essential for preventing burnout and sustaining long-term productivity.
Taking regular breaks, setting realistic work goals, and engaging in non-work-related activities are crucial for recharging your mind and avoiding the negative effects of prolonged stress. Remember, a rested mind is a more productive mind.
5. Stay Abreast of Latest Java Trends
Java, being a constantly evolving language, requires developers to stay updated with the latest trends, best practices, and tools. Keeping abreast of industry developments not only enhances your skills but also prevents you from feeling overwhelmed by unexpected changes in the ecosystem.
Following influential Java blogs like Baeldung and JavaWorld and participating in Java communities like r/java can help you stay informed and connected with the broader Java community.
Final Considerations
In the world of Java development, avoiding burnout and maintaining a sustainable pace is essential for long-term success. Prioritizing code quality, embracing TDD, practicing regular refactoring, cultivating a healthy work-life balance, and staying updated with the latest Java trends are key strategies that can help you navigate the demanding landscape of software development while avoiding burnout.
By integrating these strategies into your development routine, you can not only enhance your productivity and technical expertise but also build a more fulfilling and sustainable career in Java development. So, start implementing these strategies today to foster a wise and balanced approach to your Java development journey.
Checkout our other articles