Tackling Downtime in Azure Microservices Architecture
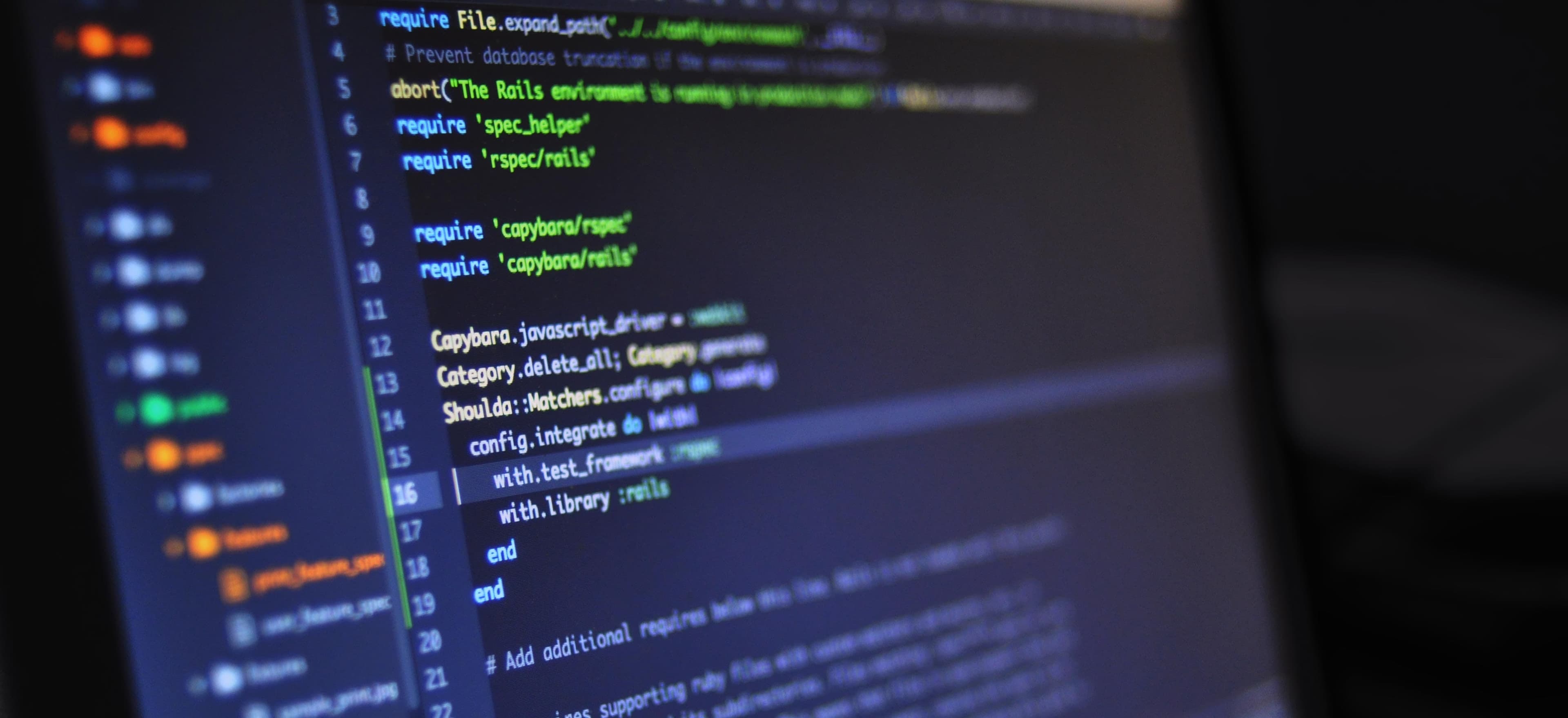
- Published on
Tackling Downtime in Azure Microservices Architecture
In today's digital age, downtime is one of the most dreaded occurrences for any online service. It not only disrupts the user experience but also impacts the revenue and reputation of a business. In a microservices architecture hosted on the Azure cloud platform, maintaining high availability is crucial. In this article, we'll explore strategies and best practices for tackling downtime in Azure microservices architecture, using Java applications as a primary focus.
Understanding Microservices Architecture
Microservices architecture is a design approach where a single application is composed of small, independent services that communicate over well-defined APIs. Each microservice is responsible for a specific business function and can be developed, deployed, and scaled independently. This architecture promotes agility, scalability, and resilience.
In an Azure environment, microservices are often implemented using Azure Kubernetes Service (AKS), Azure Service Fabric, or Azure Functions, managed by Azure Monitor and Azure Load Balancer to ensure high availability and fault tolerance.
Implementing Resilience in Java Microservices
In a microservices ecosystem, resilience is a key factor in preventing downtime. This is where the Resilience4J library comes into play for Java applications. Resilience4J is a lightweight fault tolerance library inspired by Netflix Hystrix, but designed for functional programming. It provides several resilience strategies such as circuit breakers, rate limiters, retries, and bulkheads, which help in containing and recovering from failures.
Let's consider an example where a Java microservice makes calls to external dependencies. By integrating Resilience4J into the service, we can implement a circuit breaker to avoid cascading failures and fallback mechanisms to provide graceful degradation when dependent services are unavailable.
import io.github.resilience4j.circuitbreaker.CircuitBreaker;
import io.github.resilience4j.circuitbreaker.CircuitBreakerConfig;
CircuitBreakerConfig config = CircuitBreakerConfig.custom()
.failureRateThreshold(50)
.ringBufferSizeInClosedState(5)
.ringBufferSizeInHalfOpenState(3)
.waitDurationInOpenState(Duration.ofMillis(1000))
.build();
CircuitBreaker circuitBreaker = CircuitBreaker.of("backendService", config);
Try.ofSupplier(CircuitBreaker.decorateSupplier(circuitBreaker,
() -> externalServiceCall()))
.recover(throwable -> {
// Fallback mechanism
return fallbackResponse;
});
In this code snippet, we configure a circuit breaker with a failure rate threshold and specific buffer sizes, then decorate the external service call with the circuit breaker. If the failure threshold is reached, the circuit breaker will open and the fallback response will be returned, preventing further calls to the failing service.
Leveraging Azure Traffic Manager
Azure Traffic Manager is a DNS-based traffic load balancer that enables the distribution of user traffic across multiple Azure regions, ensuring high availability and responsiveness of applications. By using Traffic Manager in conjunction with Azure Application Gateway or Azure Front Door, Java microservices can achieve global load balancing, automatic failover, and low-latency routing based on the health of the endpoints.
When configuring Traffic Manager for microservices, it's essential to define the appropriate routing method (such as Priority, Weighted, or Performance) based on the specific requirements of the application. Additionally, implementing endpoint monitoring and utilizing Azure Monitor to track the health of microservices are critical for proactive detection and mitigation of potential downtime issues.
Container Orchestration with Azure Kubernetes Service (AKS)
Azure Kubernetes Service (AKS) is a fully managed Kubernetes container orchestration service that simplifies the deployment, management, and scaling of containerized applications using Kubernetes. With AKS, Java microservices can be organized into pods, services, and deployments, providing high availability through automatic scaling, self-healing, and rolling updates.
When deploying Java microservices on AKS, it's crucial to configure liveness and readiness probes to maintain service availability. Liveness probes determine if a pod should be restarted, while readiness probes indicate if the pod is ready to serve traffic. Properly configured probes contribute to the overall resilience of microservices by preventing traffic from being directed to unhealthy instances.
apiVersion: v1
kind: Pod
metadata:
name: example-pod
spec:
containers:
- name: example-container
image: example/image:latest
readinessProbe:
httpGet:
path: /health
port: 8080
initialDelaySeconds: 5
periodSeconds: 10
livenessProbe:
httpGet:
path: /health
port: 8080
initialDelaySeconds: 15
periodSeconds: 20
In the above YAML configuration for a Kubernetes pod, readiness and liveness probes are defined to periodically check the '/health' endpoint of the Java microservice. If the service becomes unresponsive or enters an unhealthy state, AKS will handle the pod according to the probe results, minimizing downtime and ensuring seamless user experience.
Implementing Chaos Engineering
Chaos Engineering is a discipline that helps uncover weaknesses in a system by proactively testing its resilience to turbulent conditions. By intentionally introducing controlled disruptions, Java microservices running on Azure can be evaluated for their ability to withstand unpredictable failures and recover gracefully.
Utilizing tools such as Azure Chaos Studio or Chaos Monkey for Spring Boot, engineers can inject chaos scenarios like network latency, instance termination, or resource exhaustion into the microservices environment to observe and validate their behavior under adverse conditions. This proactive approach not only identifies potential points of failure but also strengthens the overall resilience of the microservices architecture.
Lessons Learned
In the realm of Azure microservices architecture, downtime is a formidable adversary that demands proactive and reactive measures to thwart its impact. By integrating resilience patterns like circuit breakers, leveraging Azure Traffic Manager for global load balancing, orchestrating microservices with AKS, and embracing Chaos Engineering, Java applications can fortify their defenses against downtime and deliver consistent, uninterrupted service to users.
Ensuring high availability and resilience in microservices is an ongoing journey that requires continuous monitoring, testing, and refinement. By adopting the strategies discussed in this article and staying abreast of evolving best practices, businesses can confidently navigate the complexities of Azure microservices architecture while mitigating the risks associated with downtime.
Implementing resilient Java microservices in Azure is a pivotal step towards safeguarding the stability and reliability of modern cloud-based applications. With the fusion of robust architecture, sound implementation practices, and vigilant maintenance, the battle against downtime can be waged effectively in the dynamic landscape of microservices.
Remember, resilience is not just about withstanding adversity – it's about thriving in the face of it.