Scaling Pains: Mastering Microservices on Heroku
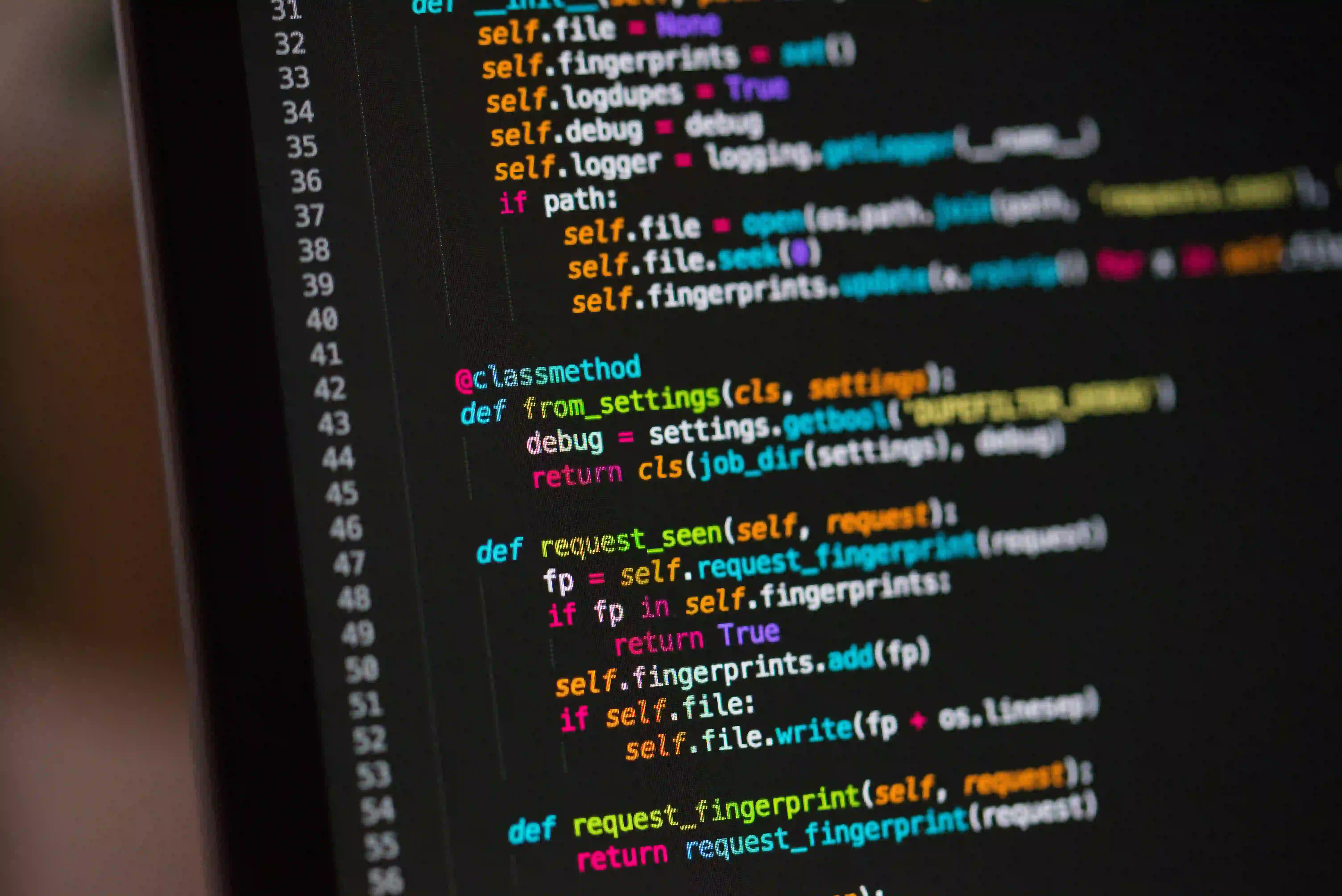
Mastering Microservices Scaling in Java on Heroku
Microservices have revolutionized the way we build and deploy applications by breaking down monolithic structures into smaller, independent services. While this approach offers various benefits such as flexibility, scalability, and resilience, it also introduces challenges, especially when it comes to scaling. In this blog post, we will delve into the world of microservices written in Java and explore the best practices for scaling them on Heroku, a popular platform for deploying cloud applications.
Understanding Microservices
Before we jump into scaling microservices, let's take a moment to understand what they are and why they are gaining widespread adoption. Microservices architecture involves breaking down a large application into smaller, self-contained services, each responsible for a specific set of functionalities. These services communicate with each other over well-defined APIs, enabling teams to work on them independently.
The Java Microservices Landscape
Java remains a dominant force in the world of enterprise software development, and it has seamlessly adapted to the microservices paradigm. Leveraging frameworks and libraries like Spring Boot, Micronaut, and Quarkus, developers can rapidly build and deploy microservices in Java. However, as the number of microservices grows and the demand on these services increases, scaling them becomes a critical concern.
Heroku: A Platform for Scalable Microservices
Heroku provides a robust platform for deploying, managing, and scaling microservices. With its container-based architecture and seamless integration with popular deployment tools, Heroku simplifies the process of scaling applications. Additionally, Heroku offers a variety of add-ons and resources to support scaling, such as database services, caching, and monitoring tools.
Scaling Strategies for Java Microservices on Heroku
Horizontal Scaling
One of the fundamental scaling strategies for microservices involves horizontal scaling. This approach entails adding more instances of a service to distribute the load effectively. In a Java application, horizontal scaling can be achieved by deploying multiple instances of the microservice using a containerization technology like Docker and orchestrating them with a tool such as Kubernetes or Heroku's native container orchestration.
Caching for Performance
Caching is a powerful technique for improving the performance of microservices, especially when dealing with read-heavy workloads. By utilizing a distributed caching solution such as Redis or Memcached, Java microservices on Heroku can efficiently cache frequently accessed data, reducing the load on backend services and improving response times.
// Example of using Redis for caching with Jedis client in a Java microservice
Jedis jedis = new Jedis("redis-host", 6379);
String cachedData = jedis.get("cached_key");
if (cachedData != null) {
// Return cached data
} else {
// Fetch data from backend service
// Store data in cache
jedis.set("cached_key", "data");
}
In the above code, we demonstrate how a Java microservice can leverage Redis for caching to improve performance and reduce the load on backend services.
Auto-Scaling with Dynos
Heroku introduces the concept of dynos, which are lightweight containers that run the microservices. Leveraging Heroku's auto-scaling feature, developers can define scaling rules based on metrics such as HTTP response time or throughput. When the defined thresholds are met, Heroku automatically scales the number of dynos to handle the increased load, ensuring that the microservices can accommodate varying levels of traffic.
# Example of scaling dynos based on throughput using Heroku CLI
heroku ps:scale web=1:Performance-m
heroku ps:autoscale web --min=1 --max=10
The above commands showcase how Heroku's autoscaling feature can be utilized to dynamically adjust the number of dynos based on the specified performance metrics.
Database Scaling and Connection Pooling
As the number of microservices grows, managing database connections becomes crucial to ensure optimal performance and resource utilization. Leveraging connection pooling libraries like HikariCP or Tomcat JDBC Pool, Java microservices on Heroku can efficiently manage database connections and scale to handle increased database loads.
// Example of configuring HikariCP connection pool in a Java microservice
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:postgresql://host:port/database");
config.setUsername("username");
config.setPassword("password");
config.setMaximumPoolSize(20);
config.setMinimumIdle(5);
HikariDataSource dataSource = new HikariDataSource(config);
In the code snippet above, we demonstrate how HikariCP can be configured in a Java microservice on Heroku to manage database connections efficiently.
Monitoring and Alerting
To effectively scale microservices, it's crucial to monitor their performance and resource utilization. Heroku provides built-in monitoring tools and integrations with third-party services like DataDog and New Relic, enabling developers to gain insights into the behavior of their microservices and set up alerts for potential scaling issues.
The Closing Argument
Scaling Java microservices on Heroku involves a combination of architectural best practices, leveraging platform features, and utilizing third-party tools. By understanding the challenges of scaling microservices and implementing the strategies discussed in this blog post, developers can ensure that their Java microservices are equipped to handle varying workloads with ease.
In conclusion, mastering microservices scaling in Java on Heroku requires a holistic approach that encompasses architectural design, performance optimization, and effective utilization of platform capabilities. By embracing these principles, developers can build and scale resilient microservices that meet the demands of modern, cloud-native applications.
Remember, understanding the intricacies of scaling microservices is an ongoing journey, and staying abreast of the latest tools and techniques is key to mastering this evolving landscape. With Heroku as a robust platform and Java as a versatile language, developers have the building blocks to create scalable, resilient microservices that power the next generation of cloud applications.
Start mastering microservices scaling on Heroku today and elevate your cloud application game!
For further reading, check out this comprehensive guide to microservices architecture and how it applies to Heroku's platform.
Reference additional resources for optimizing Java applications on Heroku here.