Boost Your DevOps: Master Continuous Testing Optimization!
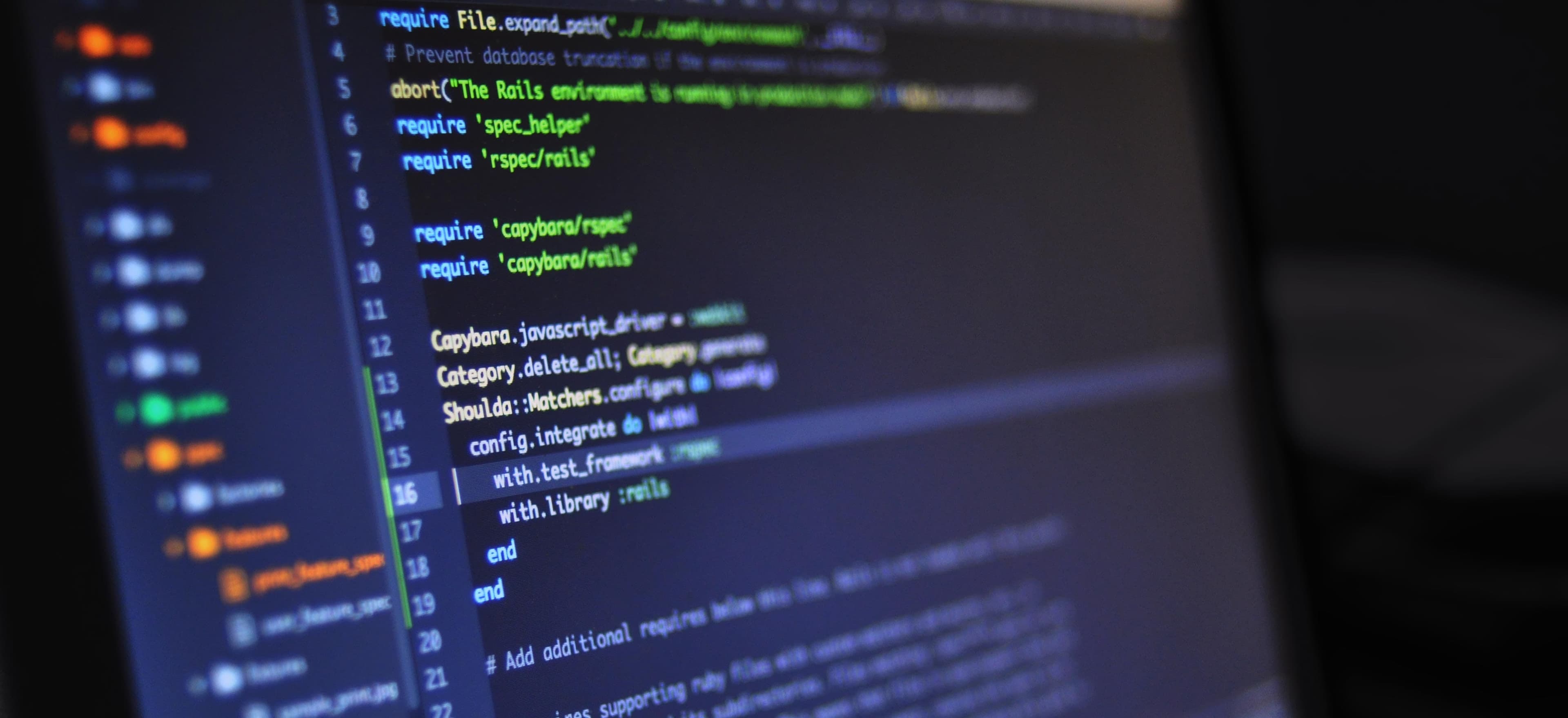
- Published on
Continuous testing is a critical component of the modern development process. As a Java developer, understanding how to optimize continuous testing can significantly improve your DevOps workflow. In this article, we will explore various strategies and best practices to master continuous testing optimization in Java.
Understanding Continuous Testing
Before diving into optimization techniques, let's take a moment to understand continuous testing. Continuous testing is the practice of running automated tests throughout the software development lifecycle. It ensures that code changes do not introduce new defects and integrates seamlessly with continuous integration and continuous delivery (CI/CD) pipelines.
In Java development, continuous testing typically involves the use of testing frameworks such as JUnit, TestNG, and Mockito, along with tools like Jenkins, Maven, and Gradle for automation and build management.
Setting the Foundation with Unit Tests
Unit tests form the foundation of continuous testing in Java. These tests validate the behavior of individual units or components of code in isolation. A robust suite of unit tests provides fast feedback to developers and helps catch bugs early in the development cycle.
Let's take a look at a simple unit test using JUnit:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(3, 5);
assertEquals(8, result);
}
}
In this example, we have a unit test for the add
method of a Calculator
class. The test validates that the addition functionality works as expected. Running these tests automatically upon code changes ensures that new developments do not break existing functionality.
Integration Testing for Comprehensive Validation
While unit tests focus on individual units, integration tests verify the interactions between different parts of the system. In Java, frameworks like Spring Boot provide excellent support for writing integration tests for web applications.
Consider the following integration test for a REST API endpoint using Spring Boot and MockMvc:
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.web.servlet.MockMvc;
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
@SpringBootTest
@AutoConfigureMockMvc
public class UserControllerIntegrationTest {
@Autowired
private MockMvc mockMvc;
@Test
public void testGetUserById() throws Exception {
mockMvc.perform(get("/users/1"))
.andExpect(status().isOk());
}
}
This integration test uses MockMvc to perform a GET request to the /users/1
endpoint and asserts that the response status is 200 OK
. By including integration tests in your continuous testing strategy, you ensure comprehensive validation of your Java applications.
Test Parallelization for Faster Feedback
As your codebase grows, your test suite can become a bottleneck in the CI/CD pipeline. Test parallelization is a technique to run tests concurrently, significantly reducing the overall test execution time. Tools like TestNG provide built-in support for parallel test execution in Java.
Let's consider a parallel test execution example using TestNG:
import org.testng.annotations.Test;
public class ParallelTestExample {
@Test
public void testMethod1() {
// Test logic
}
@Test
public void testMethod2() {
// Test logic
}
}
By annotating test methods with @Test
and configuring TestNG to run tests in parallel, you can take advantage of multi-threading to execute tests faster, especially when dealing with large test suites.
Continuous Testing Optimization with Mocking
Mocking is a powerful technique to isolate components for testing by replacing dependencies with controlled objects. In Java, Mockito is a popular mocking framework that simplifies the creation of mock objects.
Consider the following example of using Mockito to mock a database service:
import org.junit.jupiter.api.Test;
import static org.mockito.Mockito.*;
public class UserServiceTest {
@Test
public void testCreateUser() {
// Create a mock database service
DatabaseService mockDatabaseService = mock(DatabaseService.class);
// Inject the mock service into the user service
UserService userService = new UserService(mockDatabaseService);
// Define the behavior of the mock
when(mockDatabaseService.saveUser(any(User.class))).thenReturn(true);
// Test the user creation functionality
User user = new User("John Doe");
boolean result = userService.createUser(user);
// Verify the interaction with the mock
verify(mockDatabaseService, times(1)).saveUser(user);
assertTrue(result);
}
}
In this example, we use Mockito to create a mock DatabaseService
and define its behavior to simulate the successful creation of a user. Mocking allows us to focus on testing the logic within the UserService
class without depending on a real database service, improving test reliability and speed.
Embracing Test Coverage Analysis
To ensure the effectiveness of your tests, it's essential to measure test coverage. Test coverage analysis helps identify areas of code that are not adequately covered by tests, enabling you to improve the quality of your test suite.
Tools like JaCoCo (Java Code Coverage) provide detailed insights into test coverage. Let's integrate JaCoCo into a Maven project to analyze test coverage:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<configuration>
<argLine>${argLine} -javaagent:${org.jacoco.agent.path}=destfile=target/jacoco.exec</argLine>
</configuration>
</plugin>
<plugin>
<groupId>org.jacoco</groupId>
<artifactId>jacoco-maven-plugin</artifactId>
<version>0.8.7</version>
<executions>
<execution>
<goals>
<goal>prepare-agent</goal>
</goals>
</execution>
<execution>
<id>report</id>
<phase>test</phase>
<goals>
<goal>report</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
By configuring the Surefire and JaCoCo plugins in your Maven project, you can generate test coverage reports to identify areas of improvement in your test suites.
Emphasizing Feedback Loop and Automation
A key principle of continuous testing optimization is to emphasize the feedback loop. Fast and actionable feedback from tests empowers developers to make informed decisions and iterate quickly. This feedback loop is further enhanced through automation, ensuring that tests run consistently and reliably with each code change.
Integrating continuous testing into CI/CD pipelines using tools like Jenkins, GitLab CI, or CircleCI enables automated test execution and seamless feedback integration. This automation significantly accelerates the development cycle and helps maintain a high level of code quality.
Final Considerations
Continuous testing optimization is a journey that involves refining unit tests, incorporating integration tests, leveraging parallelization, embracing mocking, analyzing test coverage, and automating the feedback loop. By mastering continuous testing optimization in Java, you can enhance the efficiency, reliability, and quality of your DevOps workflow. Through the effective use of testing frameworks, tools, and best practices, Java developers can elevate their continuous testing strategy and deliver exceptional software with confidence.
Optimize your continuous testing today and elevate your DevOps game to the next level!