Simplifying DSL for Graph Data Inputs in Java Programs
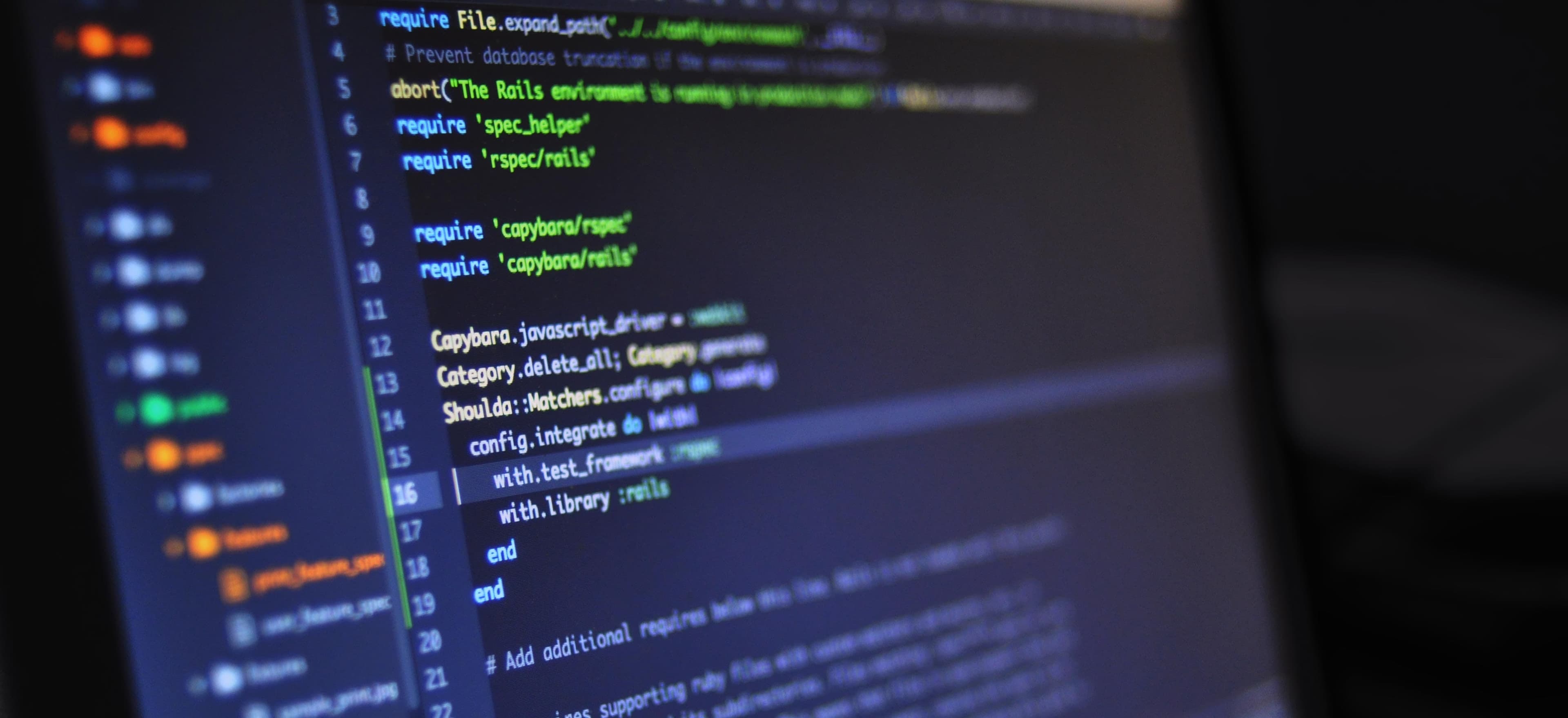
- Published on
Simplifying DSL for Graph Data Inputs in Java Programs
Graphs are fundamental data structures that are widely used in various applications, from social network analysis to computational biology. Managing graph data inputs can become cumbersome, especially in Java, where verbosity can sometimes stand in the way of clarity and simplicity. This is where Domain Specific Languages (DSL) come into play, offering a more intuitive and concise way to deal with graph data inputs. In this blog post, we'll explore how to simplify DSL for graph data inputs in Java, with a focus on making your code more readable and maintainable.
Understanding the Basics of Graph DSL
Before diving into the complexities of DSL in Java, it's essential to grasp what DSL is. DSLs are specialized mini languages designed for a specific domain. They offer a higher level of abstraction, making it easier to express solutions in terms related to the problem domain rather than the technicalities of code implementation. When applied to graph data inputs, DSL allows developers to describe graphs in a more natural and intuitive way.
Why Use DSL for Graphs?
The primary benefit of using DSL for graph data inputs is readability. Graphs can naturally be described in terms of nodes and edges, and a well-designed DSL allows you to express these concepts directly in your code. This not only makes the code more readable but also reduces the likelihood of errors.
Implementing DSL in Java
Java is not inherently designed for creating DSLs, given its strong typing and verbose syntax. However, with some clever programming techniques, we can achieve a DSL-like experience that simplifies working with graph data inputs. Here's an example of how you can implement a simple DSL for creating a directed graph:
Graph graph = GraphBuilder.directed()
.node("A").node("B").node("C")
.edge("A", "B").edge("B", "C")
.build();
In this example, we're using a fluent API style to create a directed graph. This style allows us to chain method calls in a way that reads almost like a sentence, significantly improving the readability of the code.
Breaking Down the Code
Let's break down the key components of our DSL implementation:
- Fluent API: By returning the object itself from each method, we enable the chaining of method calls. This is a crucial aspect of making the DSL fluent.
- Builder Pattern: The use of a builder (
GraphBuilder
) allows us to assemble a graph step by step. It acts as the entry point of our DSL.
Implementing the GraphBuilder
For our DSL to work, we need to implement the GraphBuilder
class. Here's a simplified version:
public class GraphBuilder {
private Graph graph;
private GraphBuilder(boolean directed) {
this.graph = new Graph(directed);
}
public static GraphBuilder directed() {
return new GraphBuilder(true);
}
public static GraphBuilder undirected() {
return new GraphBuilder(false);
}
public GraphBuilder node(String label) {
this.graph.addNode(new Node(label));
return this;
}
public GraphBuilder edge(String from, String to) {
this.graph.addEdge(new Edge(from, to));
return this;
}
public Graph build() {
return this.graph;
}
}
In this implementation, GraphBuilder
provides methods to define whether the graph is directed or undirected, add nodes and edges, and finally build the graph. The node
and edge
methods return the GraphBuilder
instance, enabling the fluent API style.
Advantages of Using DSL
The advantage of this approach is multifold:
- Readability: The DSL makes the code easier to read and understand. It's closer to the domain language of graphs.
- Maintainability: It encapsulates the complexity of graph creation, making it easier to maintain and modify.
- Reduced Boilerplate: The DSL reduces the amount of boilerplate code, making the codebase cleaner and more concise.
Resources for Further Learning
Interested in diving deeper into DSLs in Java? Here are a couple of resources to get you started:
- DSLs in Action by Debasish Ghosh. This book provides a comprehensive guide to understanding and implementing DSLs across various programming languages, including Java.
- Martin Fowler's guide on DSLs. Martin Fowler is a renowned software engineer who has written extensively on the topic of domain-specific languages.
Closing Remarks
Implementing a DSL for graph data inputs in Java might seem daunting due to the language's verbosity. However, as we've seen, with some clever design patterns and programming techniques, it's possible to create a DSL that simplifies graph construction, making your code more readable, maintainable, and expressive. By embracing DSLs, you can bring a new level of clarity and simplicity to your Java programs, especially when dealing with complex data structures like graphs.
Remember, the key to a successful DSL is understanding the domain you're aiming to simplify. Spend time designing your DSL to be as intuitive as possible, and don't be afraid to refactor as you gain insights into how best to express your domain concepts. Happy coding!