Mastering OOPs: Solving Common Polymorphism Pitfalls
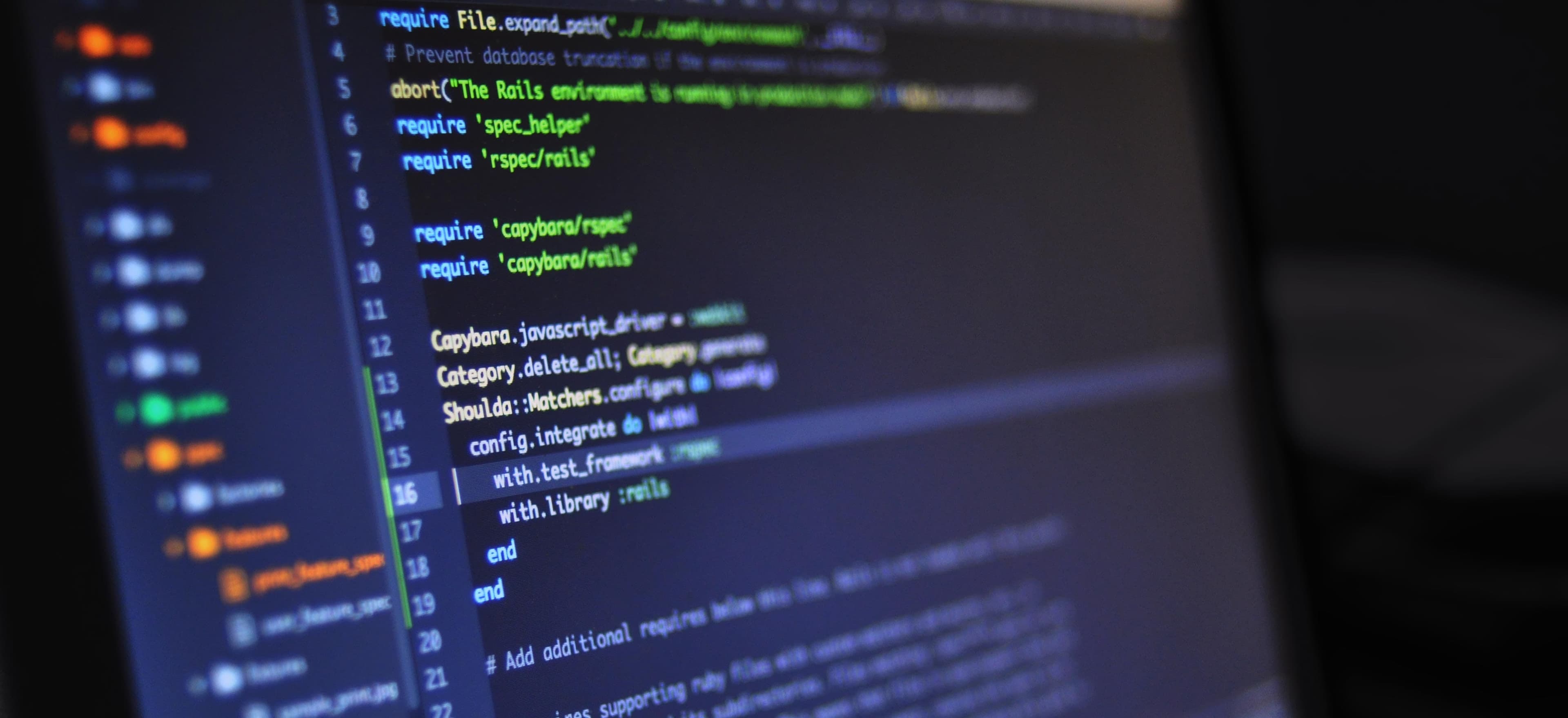
- Published on
Mastering OOPs: Solving Common Polymorphism Pitfalls
Object-oriented programming (OOP) is a powerful paradigm that enables developers to create modular, reusable, and maintainable code. One of the key features of OOP is polymorphism, which allows objects to be treated as instances of their parent class. While polymorphism is a powerful tool, it comes with its own set of pitfalls that can lead to unexpected behavior and bugs if not handled properly.
In this article, we will explore some common polymorphism pitfalls and discuss how to solve them effectively in Java. We will dive into real-world examples and provide code snippets to illustrate our points. By the end of this article, you will have a solid understanding of how to master polymorphism and avoid common pitfalls in your Java projects.
Understanding Polymorphism in Java
Before we delve into the pitfalls of polymorphism, let's briefly recap what polymorphism is in the context of Java. Polymorphism allows objects of different classes to be treated as objects of a common superclass. This means that a reference variable of a superclass can point to a subclass object.
// Superclass
class Animal {
void makeSound() {
System.out.println("Some sound");
}
}
// Subclasses
class Dog extends Animal {
void makeSound() {
System.out.println("Woof!");
}
}
class Cat extends Animal {
void makeSound() {
System.out.println("Meow!");
}
}
// Polymorphism in action
Animal dog = new Dog();
Animal cat = new Cat();
dog.makeSound(); // Output: Woof!
cat.makeSound(); // Output: Meow!
In the code snippet above, both dog
and cat
are treated as objects of the Animal
superclass, despite actually being instances of the Dog
and Cat
subclasses, respectively. This is the essence of polymorphism in Java.
Common Polymorphism Pitfalls
1. Overriding vs. Overloading
One common mistake when dealing with polymorphism in Java is confusing method overriding with method overloading. Method overriding occurs when a subclass provides a specific implementation for a method that is already defined in its superclass. On the other hand, method overloading happens when multiple methods with the same name are defined in the same class, but with different parameters.
class Animal {
void makeSound() {
System.out.println("Some sound");
}
}
class Dog extends Animal {
void makeSound() {
System.out.println("Woof!");
}
// Method overloading, not overriding
void makeSound(String type) {
System.out.println("Woof! " + type);
}
}
In the above example, the makeSound
method in the Dog
class is overloading the method, not overriding it. This is a common pitfall that can lead to unexpected behavior when dealing with polymorphism.
2. The Superclass Trap
Another common pitfall is falling into the superclass trap, where changes to a superclass can have unintended consequences on its subclasses. Consider the following example:
class Animal {
void makeSound() {
System.out.println("Some sound");
}
}
class Dog extends Animal {
void makeSound() {
System.out.println("Woof!");
}
}
class Cat extends Animal {
void makeSound() {
System.out.println("Meow!");
}
}
Animal animal = new Dog();
animal.makeSound(); // Output: Woof!
animal = new Cat();
animal.makeSound(); // Output: Meow!
// Now, let's modify the Animal class
class Animal {
void makeSound() {
System.out.println("New sound"); // Modified behavior
}
}
In this scenario, modifying the makeSound
method in the Animal
class affects all its subclasses. This can lead to unexpected behavior in the subclasses, violating the principle of least astonishment.
3. Static Methods and Polymorphism
Static methods in Java belong to the class, not the instance. Therefore, they are not overridden like instance methods, leading to a common misconception when dealing with polymorphism.
class Animal {
static void eat() {
System.out.println("Animal is eating");
}
}
class Dog extends Animal {
static void eat() {
System.out.println("Dog is eating");
}
}
public class Main {
public static void main(String[] args) {
Animal animal = new Dog();
animal.eat(); // Output: Animal is eating
}
}
In the above example, despite animal
being an instance of Dog
, the eat
method called is from the Animal
class, not the Dog
class. This is due to the fact that static methods are not subject to polymorphism.
Solutions to Polymorphism Pitfalls
1. Use the @Override
Annotation
When overriding methods in Java, using the @Override
annotation can help prevent mistakes by letting the compiler check if the annotated method is indeed overriding a method in the superclass.
class Animal {
void makeSound() {
System.out.println("Some sound");
}
}
class Dog extends Animal {
@Override
void makeSound() {
System.out.println("Woof!");
}
}
By using @Override
, you can catch errors such as misspelled method names, improper parameters, or attempting to override a method that does not exist in the superclass.
2. Favor Composition over Inheritance
To mitigate the superclass trap, favoring composition over inheritance can be a valuable approach. Instead of relying heavily on class inheritance, consider using composition to build flexible and maintainable code.
3. Avoid Using Static Methods for Polymorphic Behavior
To ensure polymorphic behavior with methods, avoid using static methods. Instead, rely on instance methods that can be overridden by subclasses to achieve the desired polymorphic behavior.
In conclusion, polymorphism is a powerful concept in OOP that allows for flexibility and extensibility in our code. But with great power comes great responsibility, as the saying goes. By understanding the common pitfalls of polymorphism and applying the solutions provided in this article, you can master the art of polymorphism in Java and write more robust and maintainable code.
Remember, the key to mastering polymorphism lies in practicing good design principles, understanding the nuances of method overriding and overloading, and being mindful of the superclass trap. With these tools in your arsenal, you can navigate the world of polymorphism with confidence and create elegant, efficient, and bug-free Java applications.
And that's it for today's blog post. For more in-depth knowledge about Java programming and other related topics, subscribe to our newsletter and stay updated with the latest industry trends. Happy coding!