Folding in Scala: Simplifying Complex Data Processing
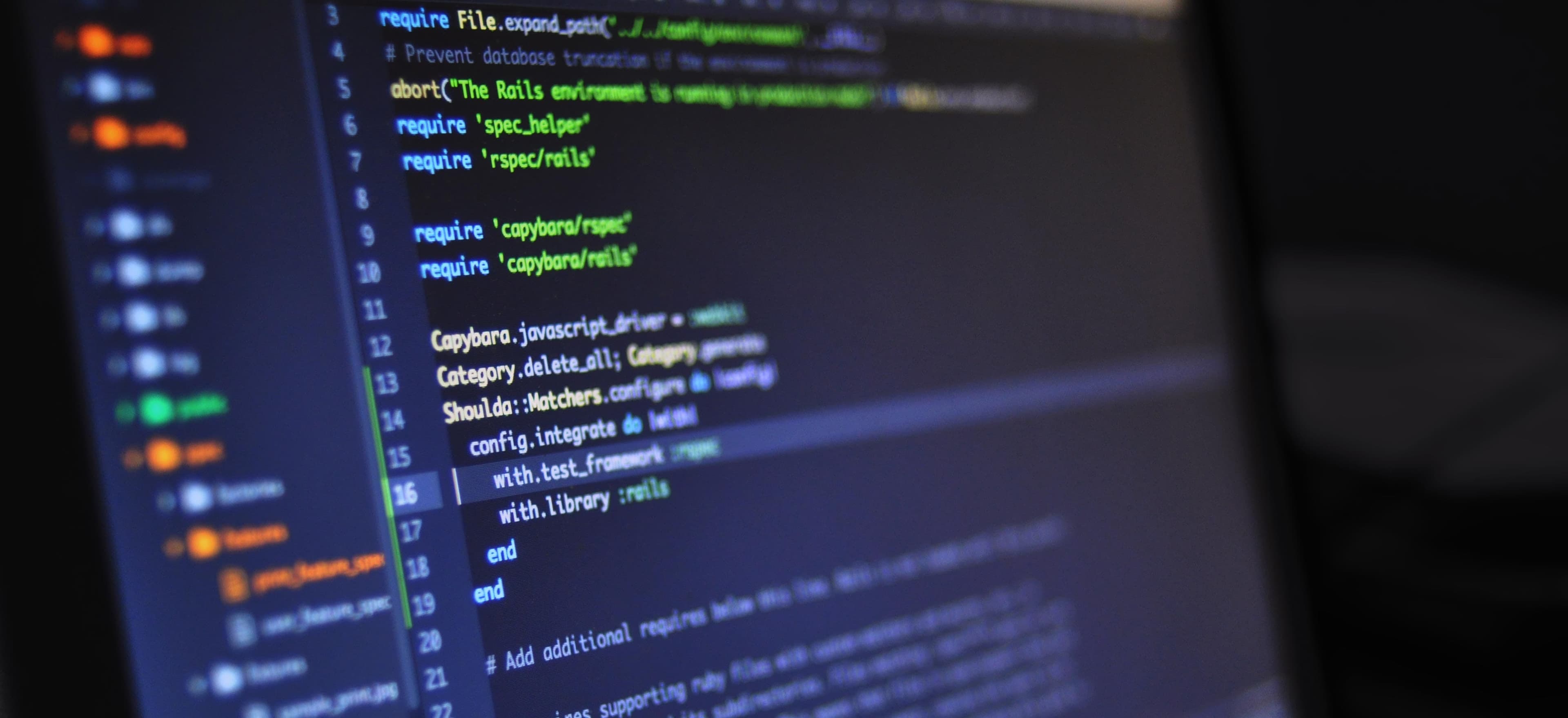
- Published on
Folding in Scala: Simplifying Complex Data Processing
In the world of Scala programming, handling complex data processing tasks is a common challenge. One powerful tool in the Scala arsenal for simplifying these tasks is the fold
function. This humble yet versatile function is often underutilized, but its impact on simplifying complex data processing tasks cannot be overstated. In this blog post, we will explore the concept of folding in Scala, understand its underlying principles, and demonstrate its practical application through examples.
Understanding Folding
At its core, folding is an operation that iterates through a collection, accumulating a result by applying a binary operation to each element in the collection. The binary operation takes two parameters: an accumulated value and an element from the collection. As the iteration progresses, the accumulated value is updated based on the result of applying the binary operation.
In Scala, the fold
function is defined for various collection types, including List
, Set
, and Array
. It takes an initial value and a binary operation as parameters. The initial value serves as the starting point for the accumulation, and the binary operation defines how the accumulation is performed.
Practical Application
To better understand the concept of folding, let's consider a practical example where folding can simplify complex data processing.
Example: Computing the Sum of Integers
Suppose we have a list of integers and we want to compute their sum. Traditionally, we might use a loop to iterate through the list and maintain a running total. However, using the fold
function, we can achieve the same result with elegant brevity.
val numbers = List(1, 2, 3, 4, 5)
val sum = numbers.fold(0)(_ + _)
println(s"The sum of the numbers is: $sum")
In this example, fold
starts with an initial value of 0 and adds each element in the list to the accumulated sum. The result is a concise and expressive way of computing the sum without explicit iteration or mutable state.
Example: Finding the Maximum Value
Let's take the example further by finding the maximum value in a list of integers using the foldLeft
function.
val numbers = List(5, 2, 8, 1, 9)
val max = numbers.foldLeft(Int.MinValue)(_ max _)
println(s"The maximum number is: $max")
Here, foldLeft
initializes the accumulation with Int.MinValue
and compares each element to update the maximum value. This approach elegantly abstracts away the iteration logic into a single function call.
Why Use Folding?
Folding offers several advantages that make it a valuable tool for simplifying complex data processing tasks:
Conciseness
Folding allows for concise and expressive code. By encapsulating the accumulation logic within a single function call, it reduces the need for explicit iteration and mutable state management.
Readability
The use of folding often leads to more readable code. It abstracts away the low-level details of accumulation, making the intent of the code more apparent to the reader.
Immutability
Folding helps in promoting immutability by encapsulating the accumulation within a purely functional operation. This aligns with functional programming principles and reduces the complexity of managing mutable state.
Composability
Folding can be combined with other higher-order functions, such as map
and filter
, to compose complex data processing pipelines. This composability leads to modular and maintainable code.
Lessons Learned
In this blog post, we've explored the concept of folding in Scala and its practical application in simplifying complex data processing tasks. By leveraging the power of folding, Scala developers can write concise, readable, and immutable code while effectively managing complex data transformations. Whether it's computing aggregates, finding extrema, or processing complex data structures, folding proves to be a versatile and indispensable tool in the Scala programmer's toolkit.
So, the next time you find yourself grappling with a complex data processing task in Scala, consider the humble fold
function and unlock its potential to simplify your code.
Happy folding!