Maven Nightmares: Top Mistake to Avoid for Success
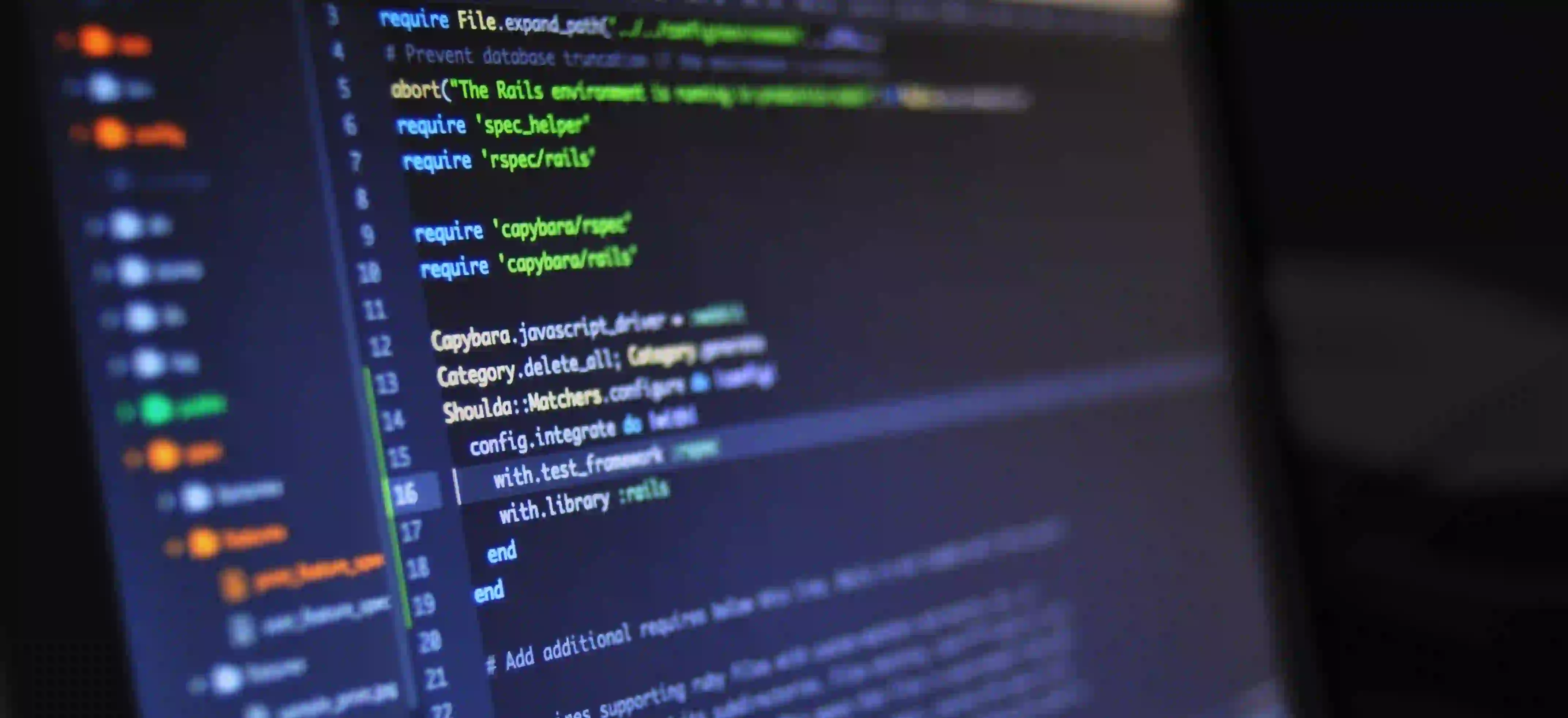
Maven Nightmares: Top Mistakes to Avoid for Success
Introduction
In the dynamic world of Java development, Maven stands out as a powerful build tool that streamlines the build process, fosters dependency management, and enforces project standardization. Its significance in modern Java development cannot be overstated. However, despite its capabilities, developers often find themselves entangled in common yet critical mistakes that can turn Maven into a nightmare. In this article, we will unravel these issues and provide practical solutions for ensuring a seamless Maven experience.
Understanding Maven
Maven, at its core, is a build automation tool that emphasizes convention over configuration. By adhering to standardized project structures and configurations, Maven enables developers to focus on writing code rather than grappling with build complexities. At the heart of Maven is the Project Object Model (POM) file, which serves as the cornerstone for defining project configurations, dependencies, and build settings. Additionally, Maven's robust system of repositories efficiently manages project dependencies, freeing developers from the burden of manual library management.
What is Maven?
Maven, developed by the Apache Software Foundation, offers a comprehensive set of build processes and project management capabilities. It simplifies the build lifecycle, handles project dependencies, and provides uniform build standards.
The Role of the POM File
The POM file, typically named pom.xml
, orchestrates the entire Maven build process. It contains vital information about the project, such as its dependencies, plugins, and configurations, and serves as the blueprint for the build.
Maven Repositories and Dependency Management
Maven repositories, including the central repository and custom repositories, are instrumental in managing project dependencies. By leveraging these repositories, developers can effortlessly incorporate external libraries into their projects without the hassle of manual management.
This foundational understanding of Maven sets the stage for unraveling the top mistakes that can hinder the efficacy of this powerful tool.
Mistake #1: Ignoring Maven Conventions
A prevalent mistake that developers make is deviating from Maven's convention over configuration principle. Maven places a strong emphasis on adhering to standardized project structures and a set of default behaviors. Ignoring these conventions can lead to confusion and unnecessary complexities in the build process. For instance, an incorrectly structured project may lead to difficulties in identifying resources, running tests, or packaging artifacts.
When developers choose to align with Maven's conventions, the build process becomes inherently streamlined and predictable. By adopting the standard directory layout and following naming conventions, developers can seamlessly integrate their projects with Maven's build lifecycle, resulting in a more manageable and predictable development experience.
<!-- Non-Maven Standard Project Structure -->
src/
- main/
- com/
- example/
- MyApp.java
- test/
- com/
- example/
- MyAppTest.java
<!-- Maven Standard Project Structure -->
src/
- main/
- java/
- com/
- example/
- MyApp.java
- test/
- java/
- com/
- example/
- MyAppTest.java
By comparing the above non-standard project structure with the Maven standard project structure, it becomes evident that adhering to Maven's standards significantly simplifies the organization of project resources and source code.
Mistake #2: Overcomplicating the POM File
The POM file, being the heart of a Maven project, can easily spiral into a convoluted configuration mess if not managed prudently. One common mistake is overcomplicating the POM file, leading to excessive redundancy, poor readability, and maintenance challenges. To avoid this, developers should strive to keep their POM files clean and comprehensible.
Utilizing properties for version management, defining dependencies in a manner that promotes readability, and judiciously incorporating parent POMs for inheritance are effective strategies to streamline POM files. By carefully structuring and organizing the POM file, developers can efficiently manage project configurations and dependencies, fostering simplicity and maintainability.
<!-- Bloated POM File -->
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>example-lib</artifactId>
<version>1.0.0</version>
</dependency>
<!-- Many more dependencies... -->
</dependencies>
<!-- Refactored POM File Using Properties and Inheritance -->
<properties>
<example-lib.version>1.0.0</example-lib.version>
</properties>
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>example-lib</artifactId>
<version>${example-lib.version}</version>
</dependency>
</dependencies>
<parent>
<groupId>com.example</groupId>
<artifactId>parent-pom</artifactId>
<version>1.0.0</version>
</parent>
The refactored POM file, which incorporates properties for version management and a parent POM for inheritance, remarkably enhances readability and maintainability, preventing the POM file from transforming into an unwieldy and perplexing artifact.
Mistake #3: Not Using Maven Profiles
Maven profiles offer a flexible approach to effectively manage different build environments and scenarios. However, neglecting to utilize profiles can lead to complications when dealing with, for example, varying configurations for development, testing, and production environments. Embracing Maven profiles empowers developers to efficiently tailor build processes to suit diverse requirements.
Defining a Maven profile within the POM file allows developers to switch between different build configurations seamlessly. Whether it's altering resource directories, specifying different plugins, or activating specific build behaviors, Maven profiles offer a powerful solution for managing diverse build scenarios.
<!-- Maven Profile Definition -->
<profiles>
<profile>
<id>dev</id>
<properties>
<build.environment>development</build.environment>
</properties>
</profile>
<profile>
<id>prod</id>
<properties>
<build.environment>production</build.environment>
</properties>
</profile>
</profiles>
By defining Maven profiles for different environments and build scenarios, developers can maintain clean and adaptable build configurations, effectively steering clear of potential impediments arising from not harnessing this powerful Maven feature.
Mistake #4: Neglecting Maven Plugins
Maven's extensible architecture allows for the integration of a wide array of plugins that automate and enhance various aspects of the build lifecycle. However, negligence towards leveraging Maven plugins to their full potential is a noteworthy mistake that can hamper the efficiency of the build process. Notably, popular plugins like the Maven Surefire Plugin for running tests and the Maven Failsafe Plugin for integration testing can significantly enhance a project's quality and reliability.
Adding plugins to the POM file sets in motion a cascade of automated tasks that can encompass anything from compiling source code and executing tests to packaging artifacts and deploying applications. By incorporating relevant plugins into the build process, developers can streamline key phases of the build lifecycle, thus fostering a more productive development environment.
<!-- Maven Surefire Plugin for Test Execution -->
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M5</version>
<configuration>
<skipTests>true</skipTests>
<!-- Additional configuration... -->
</configuration>
</plugin>
</plugins>
</build>
The addition of the Maven Surefire Plugin to the POM file exemplifies the efficiency and automation that can be harnessed to optimize the build workflow, making it imperative to avoid the neglect of valuable Maven plugins.
Mistake #5: Poor Dependency Management
Effective management of project dependencies is fundamental to the success of any Maven project. Neglecting this aspect can lead to version conflicts, redundant dependencies, and the misuse of dependency scopes, ultimately causing build instabilities. By thoroughly comprehending and adhering to best practices in dependency management, developers can sidestep the potential complexities that arise from inadequately managed project dependencies.
By specifying dependency scopes accurately and leveraging tools like the Maven Dependency Plugin to analyze and eliminate unused dependencies, developers can establish a robust foundation for hassle-free dependency management within their projects, fostering stability and reliability.
<!-- Specifying Dependency Scopes -->
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>example-lib</artifactId>
<version>1.0.0</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>com.example</groupId>
<artifactId>test-lib</artifactId>
<version>1.0.0</version>
<scope>test</scope>
</dependency>
</dependencies>
By ensuring that dependencies are scoped appropriately and pruning redundant libraries, developers can cultivate a robust foundation for seamless and efficient dependency management within their Maven projects.
A Final Look
In conclusion, steering clear of these common yet critical Maven mistakes is pivotal for ensuring a successful and stress-free project build process. By embracing Maven's conventions, streamlining POM file configurations, harnessing Maven profiles and plugins, and excelling in dependency management, developers can harness the true potential of Maven while mitigating potential impediments.
SEO Tips for the Article
- Focus Keywords: Include focus keywords such as "Maven mistakes," "Maven project," and "dependency management in Maven."
- Internal Links: Incorporate internal links to previous blog posts related to Java development or Maven to encourage site exploration.
- External Links: Include links to Maven's official documentation and reputable sources for tools and libraries mentioned.
- Meta Description: Craft a compelling meta description using focus keywords.
- Subheadings for Readability: Use subheadings for better readability and scanability.
- Engagement: Encourage reader engagement by asking readers to share their own Maven experiences in the comments.
For a comprehensive understanding of Maven and to stay abreast of best practices, visit the Maven Official Documentation and engage with the Maven community through forums and Q&A sites for real-world advice and tips.
By acknowledging these key aspects and avoiding common pitfalls, developers can pave the way for a smooth, efficient, and prosperous journey with Maven, ensuring that it remains a powerful enabler rather than a source of nightmares in their Java development endeavors.