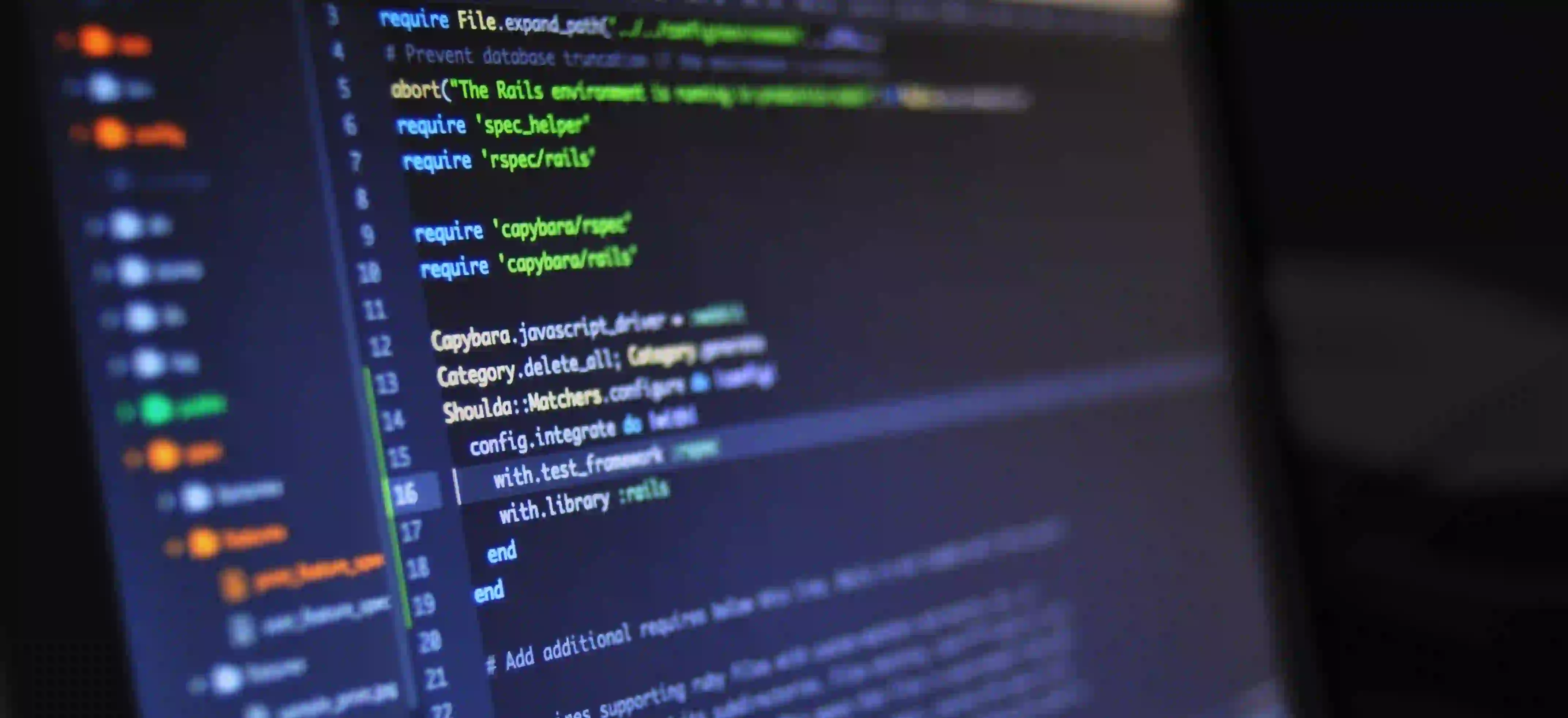
Reviving Google ClientLogin in Java: A Modern Approach
In this blog post, we'll dive into the process of reviving Google ClientLogin in Java, utilizing a modern and more secure approach. ClientLogin, a deprecated Google authentication method, has been replaced by more contemporary methods such as OAuth 2.0. However, there are instances where you may still encounter the need to work with legacy systems or APIs that rely on ClientLogin. We will explore the process of integrating ClientLogin into a Java application and discuss the potential pitfalls and security considerations involved.
What is Google ClientLogin?
Google ClientLogin was a deprecated authentication method that allowed users to access Google services using their username and password. This method involved sending HTTP requests to Google servers with the user's credentials to obtain an authentication token, which could then be used to make subsequent requests to Google APIs. However, due to security concerns such as the exposure of user credentials and lack of modern security features, Google deprecated ClientLogin in favor of OAuth 2.0.
Reviving Google ClientLogin using Java
Using the HttpURLConnection Class
We can initiate the ClientLogin process by sending an HTTP POST request to Google's authentication endpoint. Java provides the HttpURLConnection
class, which allows us to open a connection to a specified URL and perform the necessary HTTP operations. Let's take a look at a simplified example of sending a ClientLogin request using HttpURLConnection
:
import java.net.HttpURLConnection;
import java.net.URL;
import java.io.OutputStream;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class ClientLoginExample {
public static void main(String[] args) throws Exception {
URL url = new URL("https://www.google.com/accounts/ClientLogin");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
// Set the request method and properties
conn.setRequestMethod("POST");
conn.setDoOutput(true);
conn.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
// Construct the request body with user credentials
String requestBody = "Email=user@example.com&Passwd=secretpassword&service=cl";
// Send the request
try (OutputStream os = conn.getOutputStream()) {
byte[] input = requestBody.getBytes("utf-8");
os.write(input, 0, input.length);
}
// Read and print the response
try (BufferedReader br = new BufferedReader(new InputStreamReader(conn.getInputStream(), "utf-8"))) {
StringBuilder response = new StringBuilder();
String responseLine = null;
while ((responseLine = br.readLine()) != null) {
response.append(responseLine.trim());
}
System.out.println(response.toString());
}
conn.disconnect();
}
}
In this example, we create an HttpURLConnection
to Google's ClientLogin endpoint, set the request method to POST, and specify the request headers and body. Upon executing the request, we read and print the response from Google.
Why Using HttpURLConnection?
HttpURLConnection
provides a straightforward way to perform HTTP operations in Java without the need for external libraries. While it may not offer the same level of abstraction and convenience as dedicated HTTP client libraries like Apache HttpClient or OkHttp, using HttpURLConnection
allows for a minimal setup and is suitable for simple request/response scenarios such as ClientLogin.
Security Considerations
When reviving ClientLogin, it's crucial to address the security concerns associated with the method. Since ClientLogin involves sending user credentials over the network, it is susceptible to interception, especially if the communication is not encrypted. It's strongly recommended to use HTTPS when communicating with Google's authentication endpoint to ensure the confidentiality and integrity of the exchanged data.
Furthermore, storing user credentials within the application code poses a significant security risk. It's essential to follow best practices for handling sensitive information, such as utilizing secure storage mechanisms like Java's KeyStore for storing credentials and employing measures to protect against unauthorized access.
Modern Alternatives: OAuth 2.0
While reviving ClientLogin may be necessary in certain scenarios, adopting modern authentication mechanisms like OAuth 2.0 is highly encouraged for accessing Google services. OAuth 2.0 provides a more secure and flexible approach to authorization, allowing users to grant limited access to their resources without exposing their credentials. Google's OAuth 2.0 implementation supports various grant types such as authorization code, implicit, client credentials, and refresh token grants, catering to different use cases and security requirements.
Using Google's OAuth 2.0 Client Library for Java
Google offers a dedicated client library for integrating OAuth 2.0 into Java applications, the Google OAuth Client Library for Java. This library provides a set of APIs and utilities for handling OAuth 2.0 authorization flows, making it easier to authenticate and interact with Google APIs.
Let's take a brief look at how to initiate the OAuth 2.0 authorization code flow using the Google OAuth Client Library for Java:
import com.google.api.client.googleapis.auth.oauth2.GoogleAuthorizationCodeFlow;
import com.google.api.client.googleapis.auth.oauth2.GoogleClientSecrets;
import com.google.api.client.googleapis.javanet.GoogleNetHttpTransport;
import com.google.api.client.http.GenericUrl;
import com.google.api.client.json.JsonFactory;
import com.google.api.client.json.jackson2.JacksonFactory;
public class OAuth2Example {
public static void main(String[] args) throws Exception {
JsonFactory jsonFactory = JacksonFactory.getDefaultInstance();
GoogleClientSecrets clientSecrets = GoogleClientSecrets.load(jsonFactory, new FileReader("client_secret.json"));
GoogleAuthorizationCodeFlow flow = new GoogleAuthorizationCodeFlow.Builder(
GoogleNetHttpTransport.newTrustedTransport(),
jsonFactory,
clientSecrets,
Collections.singleton("https://www.googleapis.com/auth/drive.metadata.readonly"))
.build();
String authorizationUrl = flow.newAuthorizationUrl().setRedirectUri("urn:ietf:wg:oauth:2.0:oob").build();
System.out.println("Open the following URL in your browser: ");
System.out.println(authorizationUrl);
// Handle the authorization code exchange
// ...
}
}
In this example, we configure an AuthorizationCodeFlow
using the Google OAuth Client Library for Java, specifying the required client secrets, scopes, and the authorization URL. We then initiate the authorization process by generating the authorization URL and prompting the user to visit the URL for granting access.
Why Using Google's OAuth Client Library for Java?
Integrating OAuth 2.0 into Java applications without a dedicated library can be complex and error-prone, involving tasks such as handling token refreshment, managing authorization flows, and verifying received tokens. By utilizing Google's OAuth Client Library for Java, developers can leverage pre-built functionality and abstractions, reducing the complexity involved in implementing and maintaining OAuth 2.0-based authentication.
The Closing Argument
In this blog post, we explored the process of reviving Google ClientLogin in Java, offering a modern approach to integrating the deprecated authentication method into a Java application. We discussed the use of HttpURLConnection
to initiate ClientLogin requests and highlighted the importance of addressing security considerations when working with legacy authentication methods. Additionally, we emphasized the benefits of adopting OAuth 2.0 as a more secure and contemporary alternative for accessing Google services and introduced the Google OAuth Client Library for Java as a valuable tool for simplifying OAuth 2.0 integration.
While ClientLogin may still be relevant in certain contexts, it's essential to weigh the trade-offs and consider the security implications before opting for its usage. Embracing modern authentication mechanisms such as OAuth 2.0 not only enhances the security posture of applications but also aligns with industry best practices and standards.
By staying informed about the evolving landscape of authentication and authorization mechanisms, developers can make informed decisions about the most suitable approach for their applications, ensuring robust security and seamless integration with Google's APIs and services.
In conclusion, while the revival of Google ClientLogin in Java may address immediate needs, the long-term perspective favors the adoption of OAuth 2.0 for secure and compliant integration with Google services.