Unlocking the Power of JSON Binding Customization
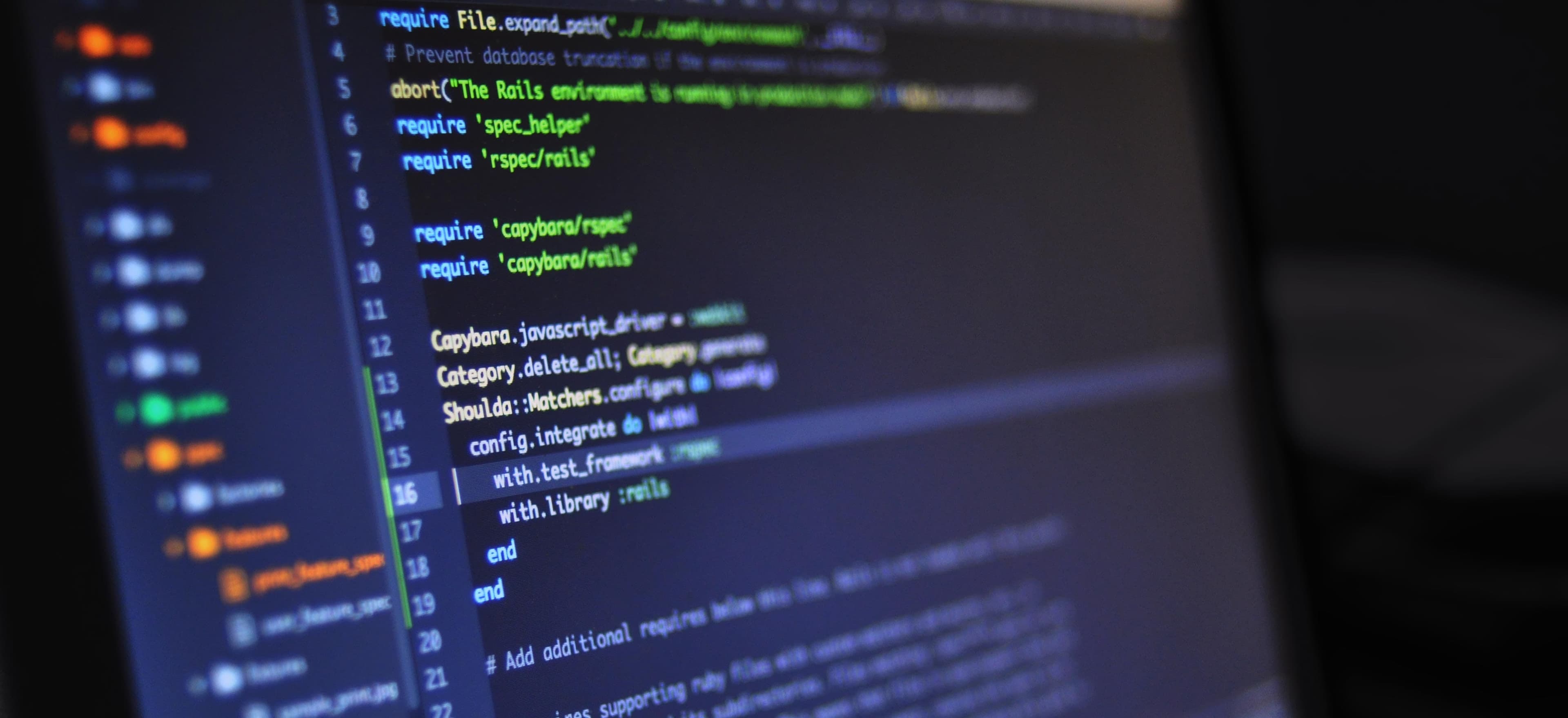
- Published on
Unlocking the Power of JSON Binding Customization
When working with Java and JSON, the ability to customize the serialization and deserialization process is crucial for handling complex data structures and ensuring seamless data exchange between applications. In this blog post, we will explore the power of JSON binding customization in Java, using the Jakarta JSON Processing API (JSON-P) to illustrate how to tailor the JSON processing to meet specific application requirements.
What is JSON Binding Customization?
JSON binding customization refers to the ability to modify the default behavior of JSON serialization and deserialization processes. This customization can include handling custom data formats, ignoring specific fields, customizing property names, and more. By leveraging JSON binding customization, developers can exert fine-grained control over how Java objects are represented in JSON format and vice versa.
Getting Started with JSON-P
Jakarta JSON Processing (JSON-P) is a standard API for JSON processing in Java, providing a convenient way to parse, generate, and manipulate JSON data. JSON-P forms the basis for JSON-B, the JSON binding API in Jakarta EE, allowing developers to customize the mapping between Java objects and JSON data.
To get started with JSON-P, you can add the following Maven dependency to your project:
<dependency>
<groupId>javax.json</groupId>
<artifactId>javax.json-api</artifactId>
<version>1.1.4</version>
</dependency>
Once you have the dependency in place, you can begin leveraging the JSON-P API to customize the JSON binding process.
Customizing JSON-Binding in Java
Customizing Serialization
Let's consider a scenario where you want to customize the serialization process to include only certain fields from a Java object when converting it to JSON. JSON-P provides the JsonbConfig
class, which allows us to customize the JSON serialization and deserialization behavior.
Here's how you can customize the serialization process to include only specific fields:
// Create a JsonbConfig with property visibility strategy
JsonbConfig config = new JsonbConfig()
.withPropertyVisibilityStrategy(new PropertyVisibilityStrategy() {
@Override
public boolean isVisible(Field field) {
// Customize which fields are visible
return field.getName().equals("firstName") || field.getName().equals("lastName");
}
});
// Create a Jsonb instance with the custom configuration
Jsonb jsonb = JsonbBuilder.create(config);
// Serialize a Java object to JSON
String json = jsonb.toJson(new Person("John", "Doe", 30));
System.out.println(json); // Output: {"firstName":"John","lastName":"Doe"}
In this example, we create a JsonbConfig
instance and customize the property visibility strategy to include only the firstName
and lastName
fields during serialization. This level of customization allows for fine-grained control over the JSON output based on specific criteria.
Customizing Deserialization
Similarly, you can customize the deserialization process to handle incoming JSON data in a custom manner. For instance, you may want to perform additional validation or transformation when deserializing JSON into Java objects. JSON-P allows you to achieve this customization through the use of custom adapters.
Let's look at an example where we customize the deserialization process using a custom adapter:
// Define a custom adapter for deserialization
public class CustomDateAdapter implements JsonbAdapter<String, Date> {
private SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
@Override
public Date adaptFromJson(String jsonValue) throws Exception {
return dateFormat.parse(jsonValue);
}
@Override
public String adaptToJson(Date date) throws Exception {
return dateFormat.format(date);
}
}
// Create a Jsonb instance with the custom adapter
Jsonb jsonb = JsonbBuilder.create(new JsonbConfig()
.withAdapters(new CustomDateAdapter()));
// Deserialize JSON into a Java object
Person person = jsonb.fromJson("{\"name\":\"John Doe\",\"dob\":\"1990-01-01\"}", Person.class);
System.out.println(person.getDob()); // Output: Sun Jan 01 00:00:00 UTC 1990
In this example, we define a CustomDateAdapter
to handle the deserialization of date values from JSON. By providing a custom adapter, we can ensure that the deserialization process aligns with our specific date format requirements, demonstrating the flexibility of JSON-P for customization.
Handling Polymorphic Types
Another aspect of JSON binding customization involves handling polymorphic types, where a single JSON representation can map to multiple Java classes based on certain criteria. This is often encountered in scenarios involving inheritance and interfaces.
JSON-P supports handling polymorphic types through the use of type adapters, allowing for seamless serialization and deserialization of polymorphic Java objects.
// Define a parent class and two subclasses
public class Shape {
// common properties and methods
}
public class Circle extends Shape {
// circle-specific properties and methods
}
public class Rectangle extends Shape {
// rectangle-specific properties and methods
}
// Create a Jsonb instance with the custom type adapter
Jsonb jsonb = JsonbBuilder.create(new JsonbConfig()
.withAdapters(new PolymorphicTypeAdapter<>(Shape.class, Circle.class, Rectangle.class)));
// Serialize and deserialize polymorphic objects
Shape shape = new Circle(5);
String json = jsonb.toJson(shape);
Shape deserializedShape = jsonb.fromJson(json, Shape.class);
In this example, we define a PolymorphicTypeAdapter
to handle serialization and deserialization of polymorphic types, allowing us to seamlessly work with both the parent and subclass objects within the JSON processing framework.
Closing the Chapter
Customizing JSON binding in Java provides developers with the flexibility to tailor the serialization and deserialization processes to meet the specific requirements of their applications. With Jakarta JSON Processing (JSON-P), the customization capabilities extend to handling complex scenarios such as property visibility, custom adapters, and polymorphic types, empowering developers to efficiently work with JSON data in their Java applications.
By harnessing the power of JSON binding customization, developers can ensure seamless integration and data exchange, ultimately enhancing the robustness and adaptability of their Java applications.
In conclusion, mastering JSON binding customization with Jakarta JSON Processing unlocks a new level of control and precision in handling JSON data in Java, enabling developers to achieve a cohesive and efficient data processing workflow.
To delve deeper into JSON customization, check out the official JSON-P documentation and JSON-B specification for comprehensive insights and advanced techniques.
Embrace the power of JSON binding customization and elevate your Java JSON processing capabilities to new heights!