Boost Your Project: 7 Rules to Perfect Documentation
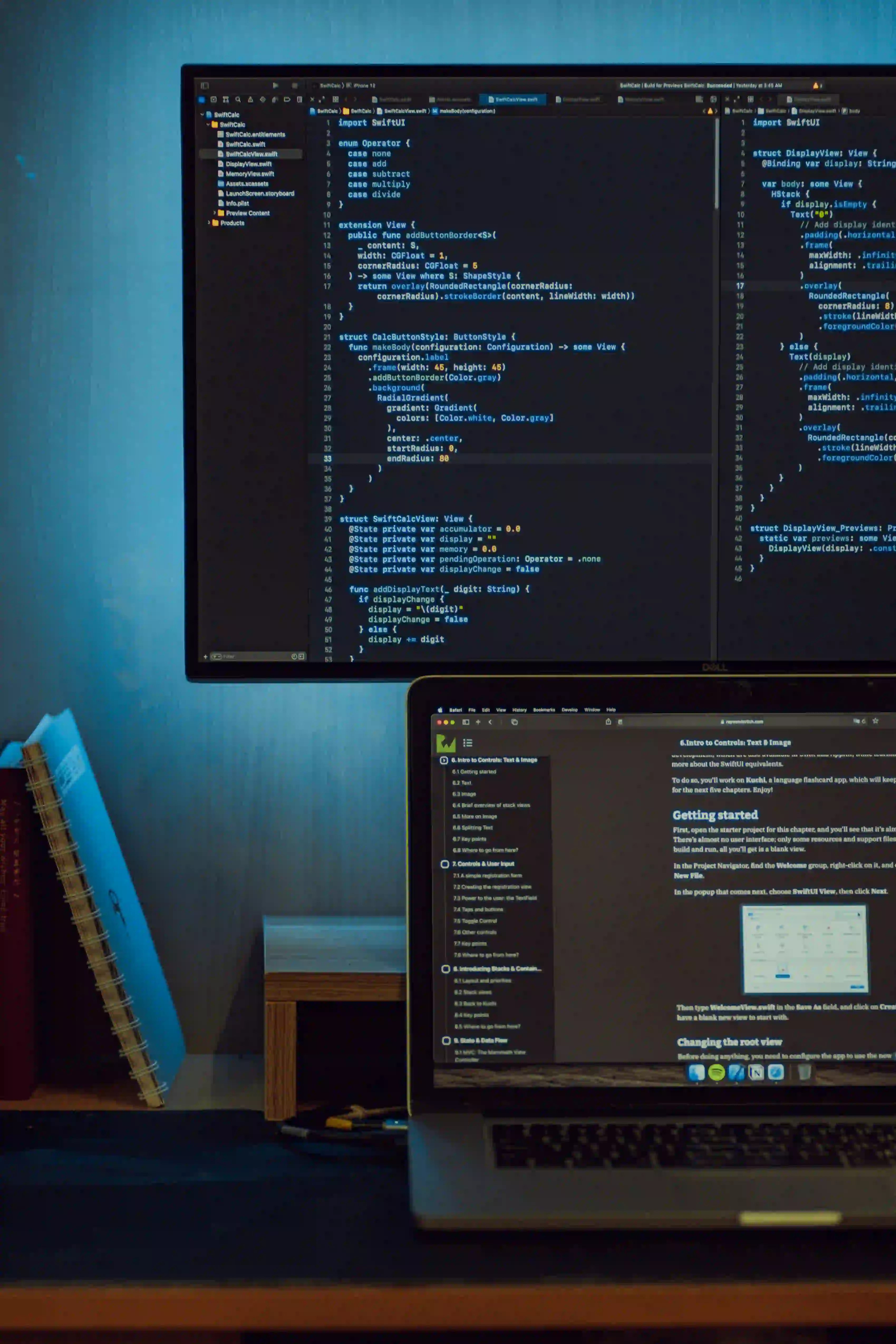
7 Essential Rules to Perfect Documentation in Java Projects
Documentation is often an overlooked aspect of software development, yet it plays a crucial role in the success of any project. Good documentation not only helps in understanding and maintaining the code but also in aiding collaboration among team members. When it comes to Java projects, writing clear and comprehensive documentation is a must-do practice. In this post, we'll outline seven essential rules to perfect documentation in Java projects, helping you boost the quality and maintainability of your codebase.
1. Use Javadoc for Public APIs
When it comes to documenting classes, interfaces, methods, and fields in Java, using Javadoc is the way to go. Javadoc is a documentation generator created by Oracle that helps in creating and maintainable documentation of APIs in Java code. By using Javadoc, you can generate API documentation in HTML format from the source code. This makes it easier for other developers to understand how to interact with your code and encourages well-documented, self-descriptive APIs.
/**
* Represents a simple example of a class in Java.
*/
public class ExampleClass {
/**
* A simple method to demonstrate Javadoc.
* @param input A string input
* @return A modified string
*/
public String modifyString(String input) {
// Implementation goes here
}
}
Why:
Using Javadoc not only helps in generating professional-looking API documentation but also encourages developers to document their code effectively. This, in turn, enhances the overall codebase maintainability.
2. Write Clear Code Comments
In addition to Javadoc, it's crucial to write clear and concise comments within the code. Comments should explain the why behind the code, especially in complex logic or algorithms. This helps in understanding the intention behind the code and assists other developers in maintaining and extending it in the future.
// Calculate the final price after applying discount
double finalPrice = calculatePrice(originalPrice, discount);
Why:
Clear code comments act as a guide for developers, providing insights into the rationale behind specific implementations. They make the code more understandable and aid in reducing the learning curve for new developers joining the project.
3. Document Code Changes
As projects evolve, so does the code. It's essential to keep the documentation in sync with the changes in the codebase. When making changes to the code, ensure that the corresponding documentation, including Javadocs and inline comments, is updated accordingly. This practice ensures that the documentation remains relevant and useful.
/**
* A utility class to perform mathematical operations.
*/
public class MathUtil {
/**
* Returns the absolute value of a number.
* @param number A number
* @return The absolute value of the number
*/
public int abs(int number) {
// Updated logic to handle negative numbers
if (number < 0) {
return -number;
} else {
return number;
}
}
}
Why:
Keeping the documentation up-to-date along with code changes avoids confusion and misinformation. It also saves time for developers who refer to the documentation for understanding the code.
4. Provide Usage Examples
Including usage examples in the documentation is a great way to demonstrate how to use a particular class or method. It helps in showcasing the intended usage and expected behavior of the code, promoting a quick understanding for other developers.
/**
* A utility class to perform file operations.
*/
public class FileUtils {
/**
* Reads the contents of a file and returns as a string.
* @param filePath The path to the file
* @return The contents of the file as a string
* @throws IOException If an I/O error occurs
* @see java.io.IOException
*/
public String readFile(String filePath) throws IOException {
// Implementation goes here
}
}
// Usage example:
FileUtils fileUtils = new FileUtils();
String content = fileUtils.readFile("example.txt");
System.out.println(content);
Why:
Usage examples act as a quick reference for other developers, showcasing how to effectively utilize the provided functionality. They serve as practical demonstrations, aiding developers in using the code correctly.
5. Maintain Readme Files
Apart from code-level documentation, maintaining a comprehensive README file at the project level is essential. The README should include information about the project structure, setup instructions, external dependencies, and usage guidelines. It serves as a starting point for new developers and provides an overview of the project at a glance.
# Project X
## Overview
Project X is a Java application for ...
## Installation
To run the project, follow these steps:
1. Install Java Development Kit (JDK) 11 or higher.
2. Clone the repository.
3. Run 'mvn clean install' to build the project.
4. Execute 'java -jar project-x.jar' to start the application.
...
Why:
A well-maintained README file serves as a central source of information for the project, aiding in onboarding new team members, contributors, and users. It provides a clear understanding of the project's purpose, setup, and usage.
6. Use Diagrams and Visual Aids
Incorporating UML diagrams, flowcharts, sequence diagrams, and visual aids in the documentation can provide a high-level understanding of the project's architecture and design. These visual representations help in conveying complex concepts and relationships in a more understandable manner.
|-----------------| |-----------------|
| Class A |------->| Class B |
|-----------------| |-----------------|
Why:
Visual aids offer a clear depiction of the project's structure and component interactions, facilitating easier comprehension for developers, reviewers, and stakeholders. They foster a quick grasp of the project's architecture and design.
7. Version Your Documentation
Just like the code, it's essential to version the documentation to align with the project's releases. By versioning the documentation, you can maintain historical records of changes, easily refer to specific documentation versions, and ensure that the documentation matches the corresponding codebase.
# Project X Documentation
## Version 1.0.0
- Added usage examples.
- Updated API documentation for clarity.
- Included sequence diagrams for component interactions.
Why:
Versioning documentation enables tracking changes over time, aligns documentation with specific project releases, and allows developers to refer to historical versions if needed. It ensures that the documentation accurately represents the codebase at different stages.
The Bottom Line
Perfecting documentation in Java projects is a crucial practice for enhancing code maintainability, fostering collaboration, and enabling seamless onboarding of new team members. By following these seven rules, you can ensure that your Java project's documentation is clear, comprehensive, and an asset to the overall development process.
Remember, well-documented code is a reflection of a well-managed project, and the effort put into documentation pays off in the long run.