Optimizing Data Access with Pivotal GemFire
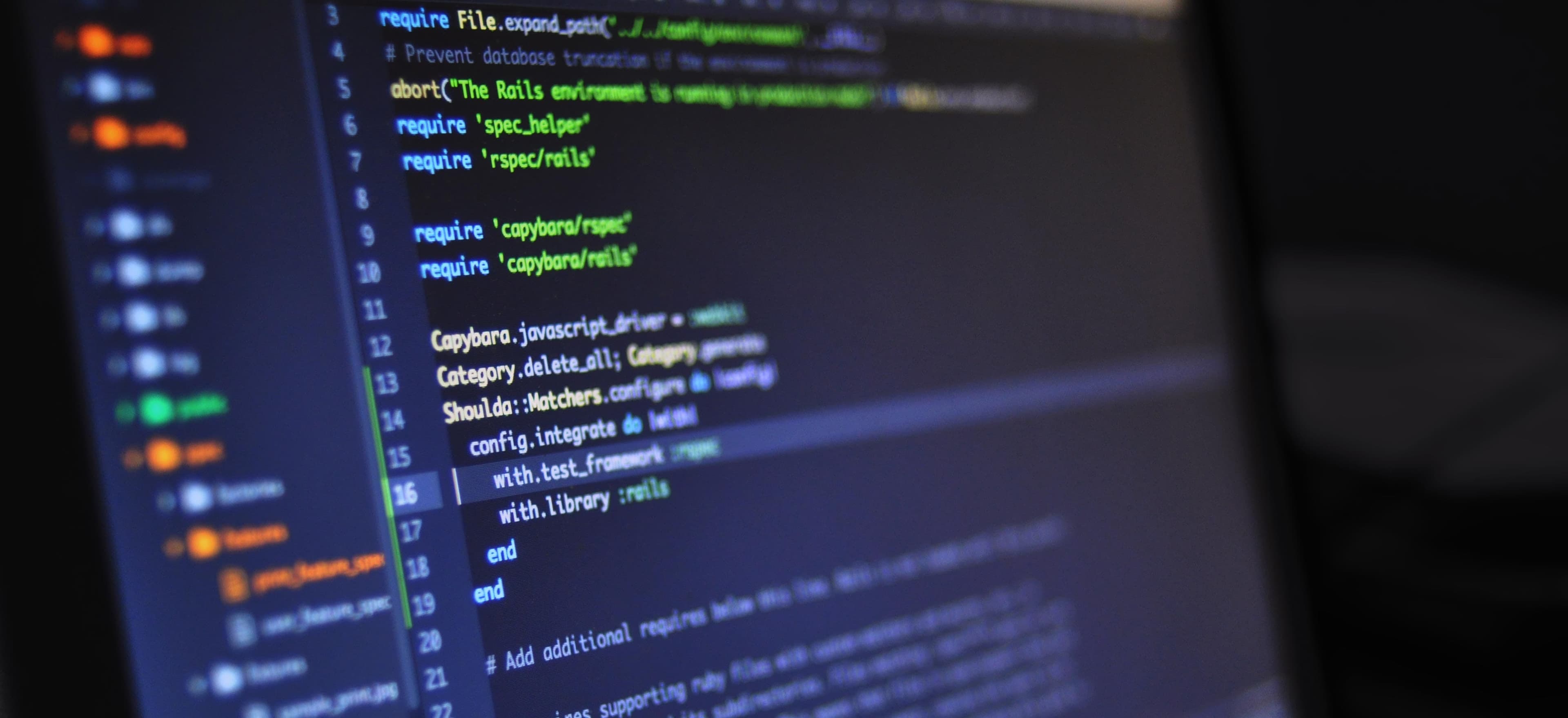
- Published on
Optimizing Data Access with Pivotal GemFire
When it comes to developing high-performance, scalable, and reliable Java applications, optimizing data access plays a critical role. One powerful tool that can help achieve these goals is Pivotal GemFire. In this article, we will explore how Pivotal GemFire can be utilized to optimize data access in Java applications.
What is Pivotal GemFire?
Pivotal GemFire is an in-memory data grid that provides high-speed, reliable access to data. It can be used as a distributed cache, a real-time event stream processor, and a system of record for data. Pivotal GemFire utilizes a distributed architecture to store and manage data across multiple nodes, providing horizontal scalability and fault tolerance.
Using Pivotal GemFire for Optimizing Data Access
1. High-Speed Data Access
Pivotal GemFire offers ultra-fast data access by storing data in-memory across the distributed grid. This in-memory storage allows for extremely low latency data access, making it ideal for use cases that require high-speed data retrieval, such as real-time analytics, trading systems, and high-frequency trading applications.
2. Horizontal Scalability
Pivotal GemFire can horizontally scale by adding more nodes to the distributed grid, allowing for seamless expansion of data storage and processing capabilities. This makes it well-suited for applications that need to handle large volumes of data and scale dynamically based on demand.
3. Data Consistency and Reliability
Pivotal GemFire ensures data consistency and reliability through features such as data partitioning, replication, and distributed transactions. These features are essential for mission-critical applications that require high data integrity and fault tolerance.
4. Real-Time Event Processing
Pivotal GemFire provides the ability to process real-time events and perform complex event stream processing. This is beneficial for applications that need to react to real-time data changes, such as IoT platforms, fraud detection systems, and real-time monitoring applications.
Integrating Pivotal GemFire into Java Applications
Integrating Pivotal GemFire into Java applications is straightforward and can be achieved using the GemFire Java API or the Spring Data GemFire project.
import org.apache.geode.cache.Region;
import org.apache.geode.cache.client.ClientCache;
import org.apache.geode.cache.client.ClientCacheFactory;
import org.apache.geode.cache.client.ClientRegionShortcut;
public class GemFireIntegration {
public static void main(String[] args) {
ClientCache cache = new ClientCacheFactory()
.addPoolLocator("localhost", 10334)
.create();
Region<String, String> region = cache
.<String, String>createClientRegionFactory(ClientRegionShortcut.CACHING_PROXY)
.create("exampleRegion");
region.put("key1", "value1");
String value = region.get("key1");
System.out.println("Retrieved value: " + value);
cache.close();
}
}
In the code snippet above, we create a GemFire client cache, define a region, put a key-value pair into the region, retrieve the value, and then close the cache. This simple example illustrates the basic steps for integrating Pivotal GemFire into a Java application.
Why Use Pivotal GemFire for Data Access?
-
High Performance: Pivotal GemFire’s in-memory data storage and distributed architecture enable high-speed data access and processing, making it suitable for performance-critical applications.
-
Scalability: The horizontal scalability of Pivotal GemFire allows for seamless expansion of data storage and processing capabilities as application demands grow.
-
Reliability: Pivotal GemFire's features for data consistency and reliability make it a solid choice for mission-critical applications where data integrity is crucial.
-
Real-Time Capabilities: The real-time event processing and complex event stream processing capabilities of Pivotal GemFire are valuable for applications that require real-time data handling.
Closing Remarks
Pivotal GemFire is a powerful tool for optimizing data access in Java applications. Its high-speed data access, horizontal scalability, data consistency, reliability, and real-time event processing capabilities make it a compelling choice for applications that demand high performance and reliability. By integrating Pivotal GemFire into Java applications, developers can achieve significant improvements in data access performance and scalability.
By leveraging Pivotal GemFire, developers can ensure that their Java applications are well-equipped to handle the challenges of modern, data-intensive environments.
In conclusion, optimizing data access with Pivotal GemFire is an effective approach to building high-performance, scalable, and reliable Java applications.
For more information on Pivotal GemFire, check out the official documentation.