Overcoming Initial Hurdles with MQTT in Java Projects
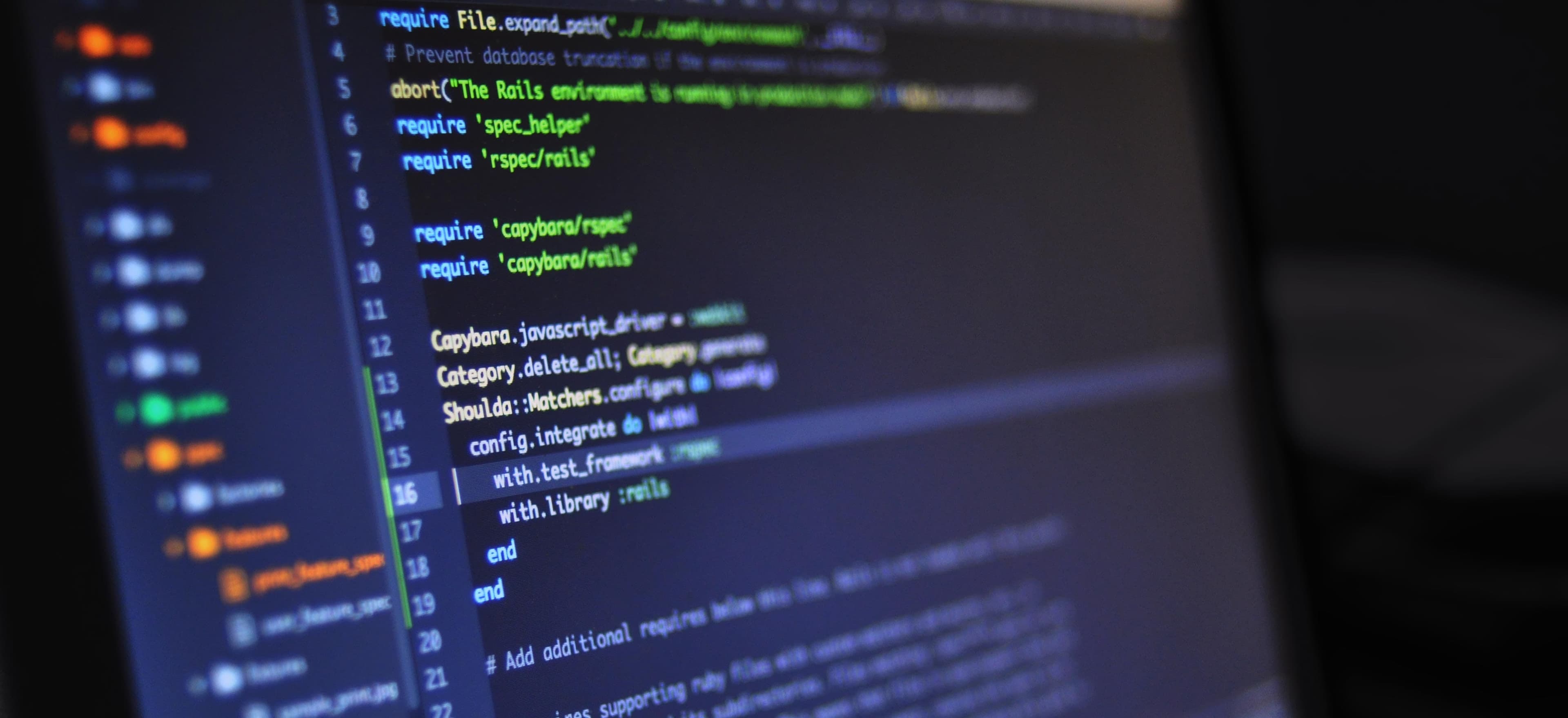
- Published on
Overcoming Initial Hurdles with MQTT in Java Projects
MQTT (Message Queuing Telemetry Transport) has become a popular choice for implementing messaging in Java projects due to its lightweight and efficient nature. However, like any new technology, working with MQTT in Java can present some initial hurdles. In this post, we will explore some common challenges developers face when starting with MQTT in Java projects and how to overcome them.
Setting Up the MQTT Client
The first challenge many developers face is setting up the MQTT client in their Java project. To overcome this, it's essential to choose a reliable MQTT client library. Eclipse Paho is a popular choice for implementing MQTT in Java. It provides a high-quality, easy-to-use API for integrating MQTT into Java applications.
Here's an example of how to set up an MQTT client using the Eclipse Paho library:
String broker = "tcp://mqtt.eclipse.org:1883";
String clientId = "JavaClient1";
MemoryPersistence persistence = new MemoryPersistence();
MqttClient client = new MqttClient(broker, clientId, persistence);
MqttConnectOptions connOpts = new MqttConnectOptions();
connOpts.setCleanSession(true);
client.connect(connOpts);
System.out.println("Connected to broker");
In this code snippet, we instantiate an MqttClient
with the broker's URL, a client ID, and a MemoryPersistence
object for storing the client's state. We then set up connection options and connect to the broker using these options.
Handling Connection Loss and Quality of Service
Another common hurdle when working with MQTT in Java is handling connection loss and Quality of Service (QoS) levels. MQTT supports three levels of QoS: 0, 1, and 2, each affecting message delivery reliability. It's crucial to understand how to handle different QoS levels and reconnect to the broker upon a connection loss.
Here's an example of how to handle connection loss and QoS levels in an MQTT Java client:
client.setCallback(new MqttCallback() {
@Override
public void connectionLost(Throwable cause) {
System.out.println("Connection lost! Reconnecting...");
// Add reconnection logic here
}
@Override
public void messageArrived(String topic, MqttMessage message) {
System.out.println("Message received: " + new String(message.getPayload()));
}
@Override
public void deliveryComplete(IMqttDeliveryToken token) {
System.out.println("Delivery complete");
}
});
client.subscribe("mytopic", 1);
In this code snippet, we set a callback on the MQTT client to handle connection loss, message arrival, and delivery completion. Upon connection loss, the client attempts to reconnect to the broker. Additionally, we subscribe to a topic with a QoS level of 1 to ensure message delivery at least once.
Managing Asynchronous Messaging
Asynchronous messaging is a fundamental aspect of MQTT, but it can become a stumbling block for developers new to the protocol. In a Java project, managing asynchronous messaging requires careful consideration of threading and concurrency to avoid blocking the main application thread.
The use of the asynchronous nature of MQTT can enhance the responsiveness of your Java application. However, it's vital to handle it properly to prevent potential issues such as message loss or thread contention.
Here's an example of managing asynchronous messaging in an MQTT Java client:
MqttAsyncClient asyncClient = new MqttAsyncClient(broker, clientId, persistence);
MqttConnectOptions connOpts = new MqttConnectOptions();
connOpts.setCleanSession(true);
asyncClient.connect(connOpts, null, new IMqttActionListener() {
@Override
public void onSuccess(IMqttToken asyncActionToken) {
System.out.println("Connected to broker asynchronously");
}
@Override
public void onFailure(IMqttToken asyncActionToken, Throwable exception) {
System.out.println("Failed to connect to broker asynchronously");
}
});
In this code snippet, we use MqttAsyncClient
to establish an asynchronous connection to the broker. We provide success and failure listeners to handle the asynchronous connection result.
Final Thoughts
Working with MQTT in Java projects can initially present some challenges, particularly in setting up the client, handling connection loss and QoS levels, and managing asynchronous messaging. By choosing a reliable MQTT client library, understanding QoS levels, and implementing proper asynchronous handling, developers can overcome these hurdles and leverage the power of MQTT in their Java applications.
In conclusion, while MQTT in Java may have its hurdles, with the right approach and understanding, developers can fully harness its capabilities, elevating the messaging aspects of their Java projects. Embracing MQTT's lightweight and efficient nature, along with proper handling of connections and messaging, can lead to robust and responsive Java applications.
In your experience, what challenges have you faced when working with MQTT in Java projects, and how did you overcome them?
Remember to check out Eclipse Paho and the official MQTT documentation for further insights into using MQTT effectively in Java.
Disclaimer: The code snippets provided are for illustrative purposes and may require additional error handling and production-level considerations depending on the specific use case.