Simplify Your Code: Mastering Lambda Expressions & Streams
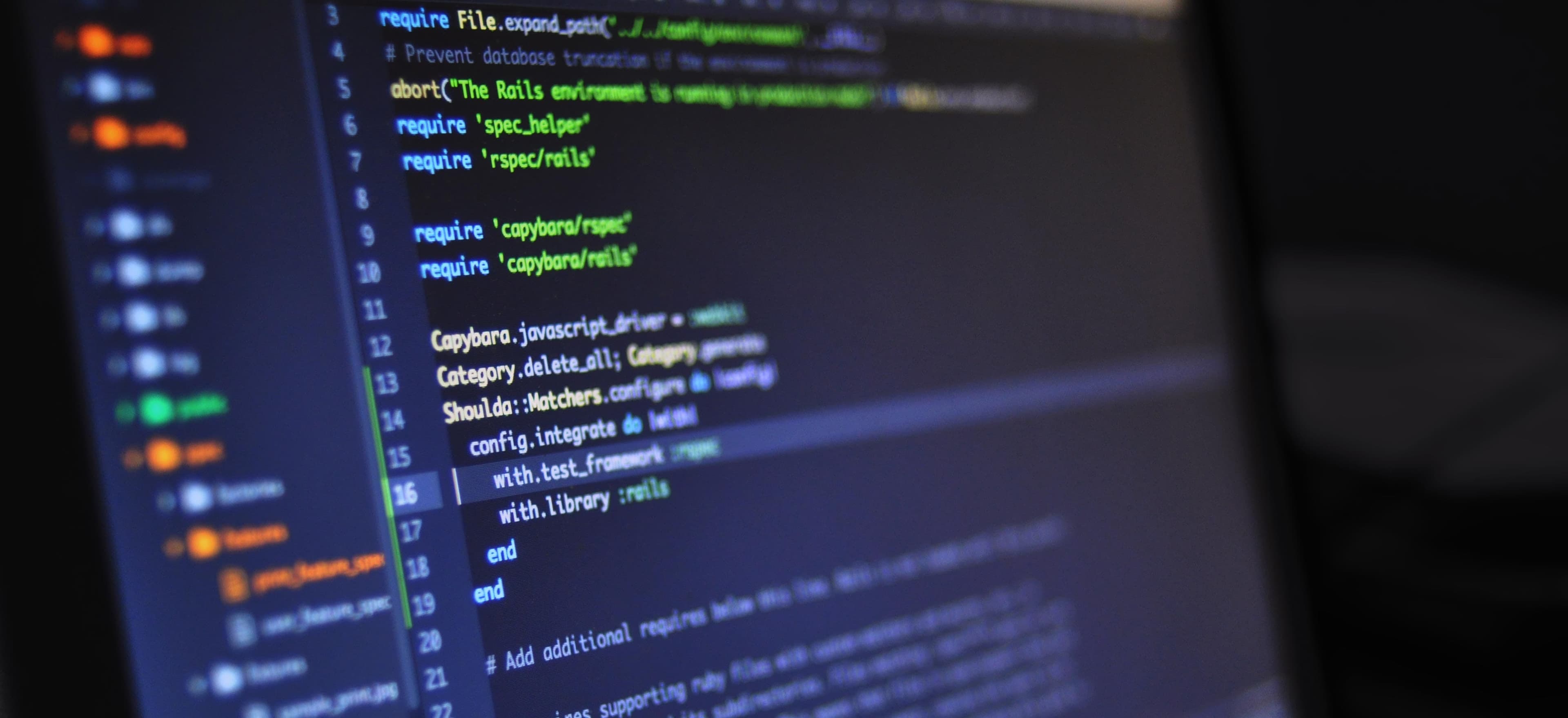
- Published on
Understanding Lambda Expressions and Streams in Java
In this blog post, we will delve into the world of Lambda expressions and Streams in Java. These features were introduced in Java 8, bringing a significant shift in the way we write code, making it more concise, readable, and efficient. Let's explore how these powerful tools can simplify your code and make your Java development experience more enjoyable.
What are Lambda Expressions?
Lambda expressions introduce a new syntax for writing anonymous functions in Java. They enable you to treat functionality as a method argument, or code as data. This is particularly useful in scenarios where you need to pass a piece of functionality around your code.
Let's consider a traditional approach to sorting a list of strings:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Prior to Java 8
Collections.sort(names, new Comparator<String>() {
@Override
public int compare(String s1, String s2) {
return s1.compareTo(s2);
}
});
With Java 8's Lambda expressions, the same task can be achieved more succinctly:
names.sort((s1, s2) -> s1.compareTo(s2));
The Lambda expression (s1, s2) -> s1.compareTo(s2)
represents the implementation of the compare
method in the Comparator interface. This concise syntax greatly reduces the boilerplate code required in the pre-Java 8 approach.
Why Use Lambda Expressions?
- Readability: Lambda expressions make your code more readable by focusing on the intention rather than the mechanics of how to achieve it.
- Conciseness: They reduce the verbosity of your code, making it more compact and maintainable.
- Functional Programming: They enable a more functional style of programming, allowing you to write more expressive and declarative code.
Leveraging Streams
Streams, introduced in Java 8, provide a new way to work with collections. A stream is a sequence of elements that supports various methods to perform computations on those elements. By using streams, you can process collections in a declarative, fluent style, without explicitly writing loops.
Let's take a look at an example of filtering a list of names to print only those starting with the letter "A" using a traditional approach:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Prior to Java 8
for(String name : names) {
if(name.startsWith("A")) {
System.out.println(name);
}
}
Using streams, the same task can be accomplished more elegantly:
names.stream()
.filter(name -> name.startsWith("A"))
.forEach(System.out::println);
In this example, names.stream()
creates a stream from the list of names. The filter
operation processes the elements and only retains those starting with the letter "A", and forEach
performs an action for each remaining element.
Benefits of Stream API
- Declarative Style: Streams allow you to write code in a more declarative style, making your intentions clearer and the code more expressive.
- Parallelism: Stream API has built-in support for parallelism, enabling easier parallel processing of data.
Common Use Cases
Lambda expressions and Streams are highly effective in a variety of scenarios, including:
- Collection Transformation: Converting and manipulating data within collections.
- Filtering and Extraction: Selecting specific elements based on certain criteria.
- Data Processing: Performing computations over a collection of data.
Key Takeaways
In conclusion, Lambda expressions and Streams bring a modern and functional approach to Java programming – allowing you to write more concise, expressive, and readable code. By leveraging these powerful features, you can simplify your code, making it more maintainable and efficient. Embracing Lambda expressions and Streams opens up opportunities for cleaner, more functional Java code, and fosters a deeper understanding of functional programming paradigms.
Now that you've gained an understanding of these features, it's time to start incorporating them into your Java projects and witness the transformation they bring to your codebase. Happy coding!
To deepen your knowledge, consider exploring this guide for further insights into Lambda expressions and Streams in Java.