Streamline Your Tasks: Top 25 Workflow Tips & Tools
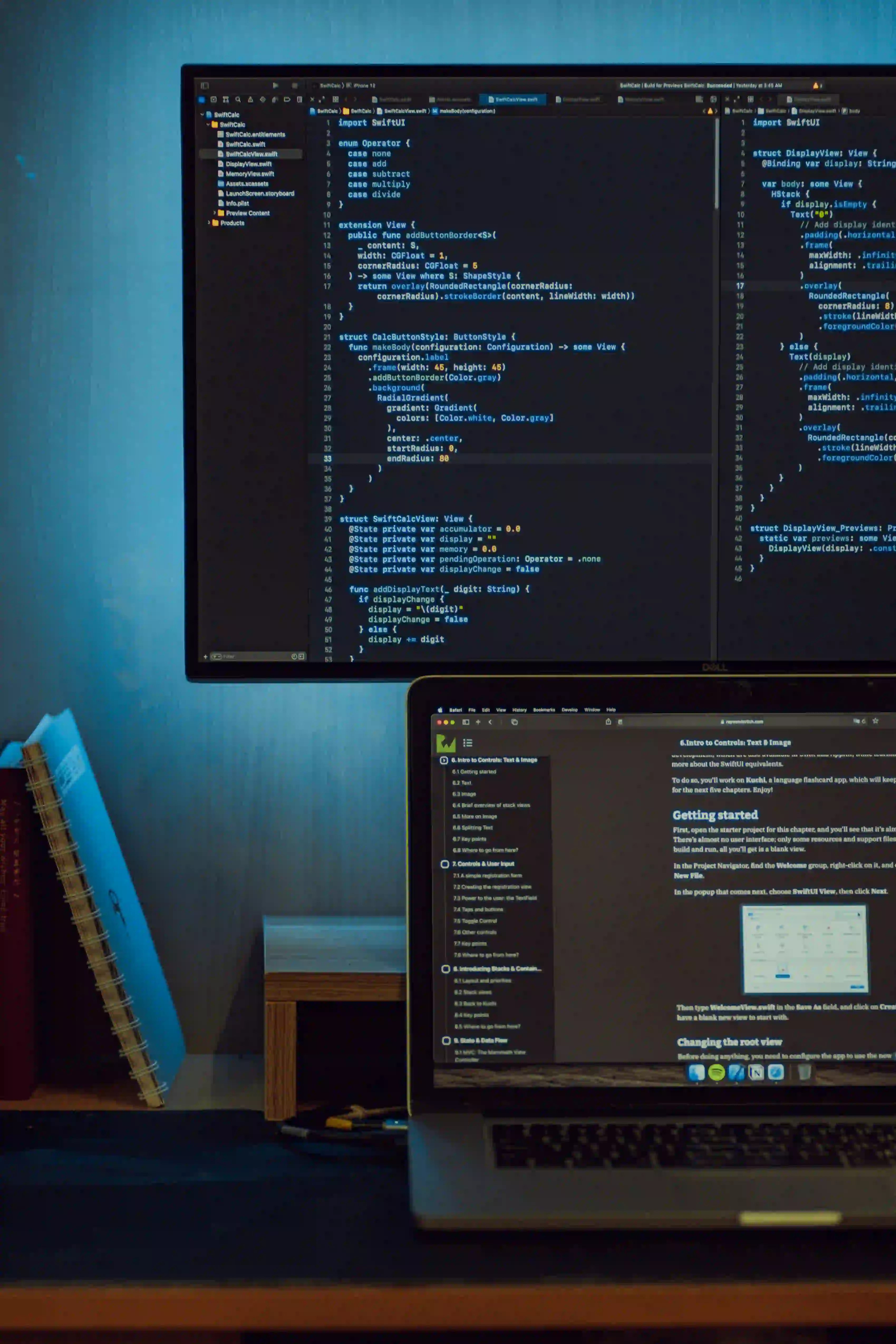
Boost Your Productivity with These 25 Java Workflow Tips and Tools
As a Java developer, improving your workflow can significantly enhance your productivity and efficiency. Whether you're working on a small project or a large-scale application, using the right tools and implementing effective workflow tips can make a substantial difference in your development process.
In this article, we'll explore 25 valuable workflow tips and tools that can help you streamline your Java development process, from code organization and version control to testing and deployment.
Let's dive into the top 25 Java workflow tips and tools to elevate your development game.
1. Leverage Git for Version Control
Utilize Git for version control to track changes, collaborate with other developers, and maintain a clear history of your codebase. Git provides a robust platform for managing different versions of your code and facilitates seamless collaboration within your team. Make sure to familiarize yourself with basic Git commands such as git commit
, git push
, and git pull
to effectively manage your codebase.
// Example Git commit command
git commit -m "Add new feature implementation"
2. Integrate Continuous Integration (CI) Tools
Incorporate CI tools such as Jenkins, Travis CI, or CircleCI to automate the build and testing processes. CI tools allow you to detect issues early in the development cycle, ensuring that your codebase remains stable and reliable. By automating tasks like compilation, testing, and code analysis, you can accelerate the feedback loop and identify potential issues swiftly.
3. Embrace Containerization with Docker
Utilize Docker to create lightweight and portable containers for your Java applications. Docker simplifies the deployment process by encapsulating your application and its dependencies into a container. This approach ensures consistency across different environments, making deployment and scaling more efficient.
4. Implement Code Review Practices
Incorporate code review practices within your team to promote collaboration and maintain code quality. Tools like GitHub's pull requests and Bitbucket's code review features facilitate structured code reviews, enabling developers to provide feedback and catch potential issues before they become entrenched in the codebase.
5. Automate Documentation Generation
Leverage tools like Javadoc to automate the generation of code documentation. By writing clear and concise documentation directly within your code and using tools to generate documentation from code comments, you can ensure that your codebase remains well-documented and understandable for other developers.
/**
* Returns the sum of two integers.
* @param a The first integer
* @param b The second integer
* @return The sum of the two integers
*/
public int add(int a, int b) {
return a + b;
}
6. Utilize Dependency Management Systems
Employ robust dependency management systems such as Maven or Gradle to streamline the incorporation of third-party libraries and manage project dependencies. These tools simplify the process of managing external dependencies, ensuring that your project remains organized and efficient.
7. Leverage IDE Features for Code Refactoring
Take advantage of integrated development environments (IDEs) such as IntelliJ IDEA or Eclipse, which offer powerful code refactoring features. IDEs can assist you in restructuring your codebase, improving its maintainability, and enhancing overall code quality.
8. Adopt Unit Testing Frameworks
Embrace unit testing frameworks like JUnit or TestNG to automate the testing of individual components in your Java application. Writing unit tests ensures that each unit of your code functions as expected, providing confidence in the correctness of your implementation and facilitating future changes.
// Example JUnit test case
@Test
public void additionTest() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
9. Integrate Static Code Analysis Tools
Incorporate static code analysis tools such as FindBugs, PMD, or Checkstyle to identify potential bugs, code style violations, and other issues within your codebase. These tools provide valuable insights into code quality and help enforce consistent coding standards across your project.
10. Utilize Logging Frameworks
Integrate logging frameworks like Log4j or SLF4J to facilitate effective logging within your applications. Proper logging helps in debugging, monitoring application behavior, and tracking down issues in production environments.
// Example Log4j logging configuration
log4j.rootLogger=DEBUG, console
log4j.appender.console=org.apache.log4j.ConsoleAppender
log4j.appender.console.layout=org.apache.log4j.PatternLayout
log4j.appender.console.layout.ConversionPattern=%-4r [%t] %-5p %c %x - %m%n
11. Employ Task Automation with Gradle Scripts
Utilize Gradle build scripts to automate repetitive tasks such as compilation, testing, and packaging. Gradle offers a flexible and powerful build automation system that allows you to define custom tasks and automate various aspects of the development process.
12. Monitor Code Quality with SonarQube
Integrate SonarQube into your workflow to assess and track the overall code quality of your Java projects. SonarQube provides valuable insights into code smells, bugs, vulnerabilities, and can help enforce coding standards, ensuring the long-term maintainability of your codebase.
13. Use Design Patterns for Maintainable Code
Apply design patterns such as Singleton, Factory, or Builder to write maintainable, readable, and scalable code. Design patterns provide proven solutions to common design problems, promoting code reusability and maintainability in your Java applications.
14. Implement Git Flow for Collaborative Development
Adopt Git Flow, a branching model for Git, to streamline collaborative development and release management. Git Flow defines a branching strategy that facilitates features development, bug fixing, and release preparation, enabling seamless collaboration within your development team.
15. Employ Agile Development Practices
Incorporate agile development methodologies such as Scrum or Kanban to iteratively deliver high-quality software. Agile practices promote collaboration, adaptability, and customer involvement, fostering a more responsive and efficient development process.
16. Enhance Security with OWASP Dependency-Check
Utilize OWASP Dependency-Check to identify and mitigate known vulnerabilities within your project dependencies. This tool analyzes project dependencies and provides a report of known vulnerabilities, empowering you to proactively address security issues within your Java applications.
17. Optimize Performance with Profiling Tools
Leverage profiling tools like Java Mission Control or YourKit to analyze the performance of your Java applications. Profiling tools offer insights into CPU and memory usage, thread behavior, and can help identify performance bottlenecks, enabling you to optimize your applications for better efficiency.
18. Implement Continuous Delivery Practices
Embrace continuous delivery practices to automate the deployment process and deliver software updates frequently and reliably. Automation tools such as Ansible or Chef can help streamline the deployment pipeline, enabling you to deliver changes to production with minimal manual intervention.
19. Document Coding Standards and Best Practices
Establish and document coding standards, best practices, and project guidelines to ensure consistency and clarity across your development team. Providing clear guidelines and standards promotes code maintainability, readability, and collaboration within the team.
20. Incorporate APM Solutions for Performance Monitoring
Integrate Application Performance Monitoring (APM) solutions like New Relic or AppDynamics to gain real-time insights into your Java application's performance. APM tools enable you to monitor application health, diagnose performance issues, and ensure optimal user experience.
21. Utilize Mocking Frameworks for Testing
Employ mocking frameworks like Mockito or PowerMock to create mock objects for testing. Mocking frameworks allow you to isolate components for testing, simulate behavior, and verify interactions, enhancing the effectiveness of your unit tests.
// Example Mockito mock setup
@Test
public void testProcessData() {
DataService dataService = Mockito.mock(DataService.class);
// Specify mock behavior
when(dataService.retrieveData()).thenReturn("Mocked data");
// Test the component using the mock
DataProcessor dataProcessor = new DataProcessor(dataService);
assertEquals("Processed: Mocked data", dataProcessor.processData());
}
22. Embrace DevOps Practices for Collaboration
Embrace DevOps practices to foster collaboration and communication between development and operations teams. DevOps principles emphasize automation, continuous feedback, and shared responsibilities, promoting a culture of collaboration and efficiency within your organization.
23. Use Profiling to Identify Memory Leaks
Leverage Java profiling tools to identify and troubleshoot memory leaks within your applications. Analyzing memory usage and identifying memory leaks early in the development process can prevent performance degradation and potential out-of-memory errors in production environments.
24. Implement Error Monitoring and Reporting
Integrate error monitoring and reporting tools such as Sentry or Rollbar to track and respond to errors in real-time. These tools provide insights into application errors, exceptions, and performance issues, enabling you to diagnose and address issues promptly.
25. Embrace Pair Programming for Knowledge Sharing
Adopt pair programming practices within your team to enhance knowledge sharing, code quality, and collaborative problem-solving. Pair programming fosters real-time code reviews, knowledge transfer, and enhances overall code quality through shared understanding and expertise.
Wrapping Up
By incorporating these 25 workflow tips and tools into your Java development process, you can enhance your productivity, code quality, and overall efficiency. From version control and continuous integration to testing, deployment, and collaboration practices, optimizing your workflow will contribute to delivering high-quality software and fostering a positive development environment.
Elevate your Java development game by implementing these best practices and leveraging the right tools to streamline your workflow and drive success in your projects.