Mastering Docker API Gateways in Microservice Dev
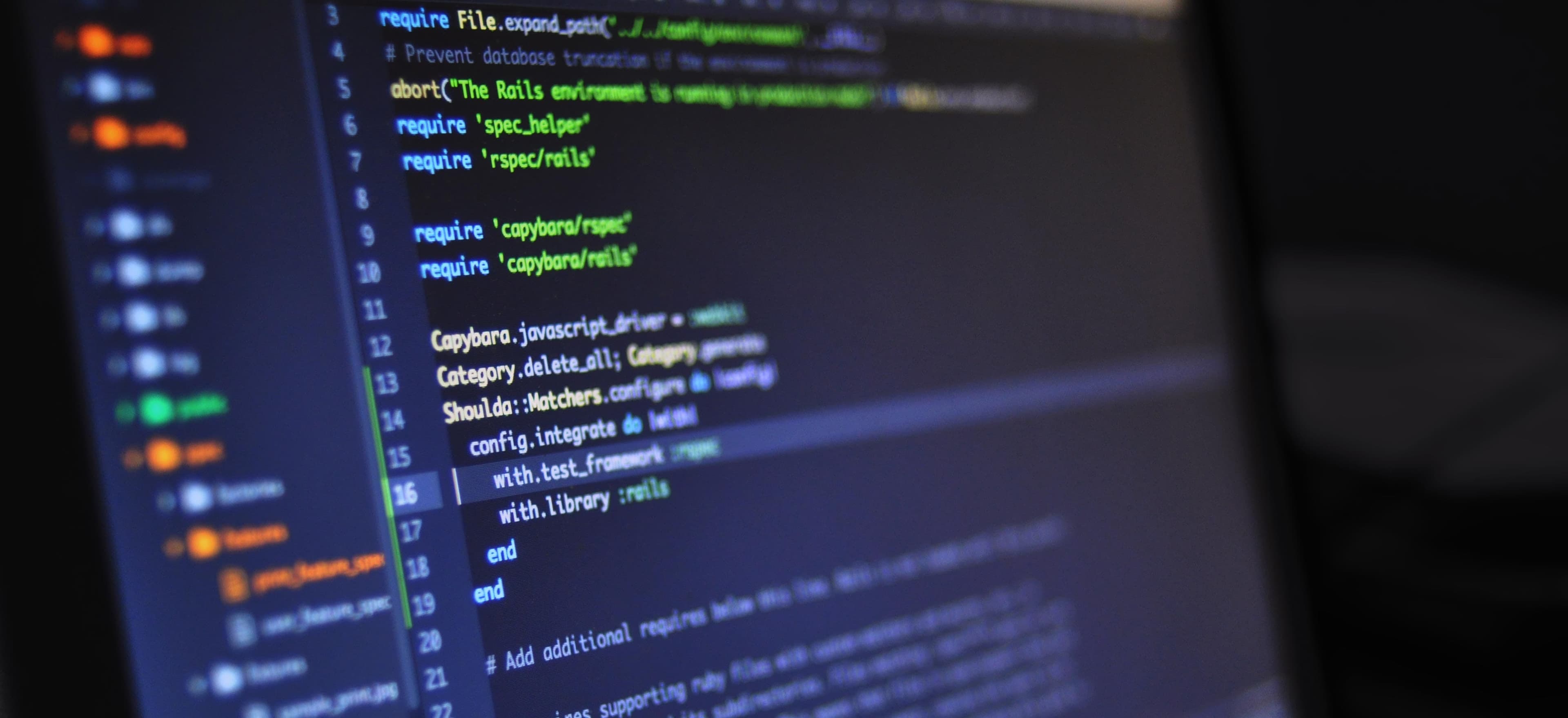
- Published on
Mastering Docker API Gateways in Microservice Development
In the complex world of microservice architectures, communication is key. However, as these architectures grow, so does the complexity of managing these communications. Enter the pivotal role of an API Gateway. The use of API Gateways, especially when combined with containerization technologies like Docker, has become a game-changer for developers. By mastering Docker API Gateways in microservice development, developers can efficiently manage services, streamline authentication and security, and ensure an optimal end-user experience.
Why Docker API Gateways? Understanding the Basics
Before delving into the intricacies of Docker API Gateways, it's important to understand the why. In a microservices architecture, each microservice is responsible for a specific service or function. While this modularity and isolation of services offer numerous benefits like easier scaling and deployment, it also poses significant challenges in managing multiple points of interaction. That's where Docker API Gateways come in, serving as a single-entry point for all client requests, directing them to the appropriate service, and then combining the results to send back to the client. This not only simplifies the client's communication with backend services but also offers centralized security, monitoring, and rate limiting.
Key Benefits:
-
Centralized Management: Handles all client requests and routes them to the correct microservice.
-
Enhanced Security: Offers a single point to implement authentication, SSL termination, and other security measures.
-
Simplified Client-side Communication: Clients no longer need to manage multiple endpoints for different services.
-
Performance Optimization: Capable of handling load balancing and caching to enhance performance.
Setting Up a Docker API Gateway
Now, let's dive into setting up a Docker API Gateway. For the purpose of this guide, we'll use the popular open-source API gateway, Kong, due to its extensive features and Docker compatibility.
Step 1: Running Kong in Docker
First, ensure Docker is installed on your system. Then, run Kong within Docker by executing the following command:
docker run -d --name kong \
-e "KONG_DATABASE=off" \
-e "KONG_PROXY_ACCESS_LOG=/dev/stdout" \
-e "KONG_ADMIN_ACCESS_LOG=/dev/stdout" \
-e "KONG_PROXY_ERROR_LOG=/dev/stderr" \
-e "KONG_ADMIN_ERROR_LOG=/dev/stderr" \
-e "KONG_ADMIN_LISTEN=0.0.0.0:8001" \
-p 8000:8000 \
-p 8443:8443 \
-p 8001:8001 \
-p 8444:8444 \
kong:latest
Why this command? It starts a Kong API gateway with the database disabled (for simplicity in this example), exposes necessary ports, and sets up logging. This command ensures Kong runs as a container in Docker, acting as your API Gateway.
Step 2: Configuring Services and Routes
With Kong running, the next step is to configure services and routes. This involves telling Kong where to route incoming requests. For illustrative purposes, let's assume there's a microservice called example-service
running and accessible via http://example-service:8080
.
Adding a Service:
curl -i -X POST --url http://localhost:8001/services/ \
--data 'name=example-service' \
--data 'url=http://example-service:8080'
Adding a Route to the Service:
curl -i -X POST --url http://localhost:8001/services/example-service/routes \
--data 'paths[]=/example'
What does this achieve? These commands register example-service
with Kong and specify that any requests coming to /example
should be routed to http://example-service:8080
. This is crucial for directing client requests to the correct microservice.
Leveraging Advanced Features
While setting up your API Gateway and routing requests is foundational, the real power of Docker API Gateways in microservice development lies in leveraging their advanced features, like authentication, rate limiting, and service discovery.
Implementing Authentication:
Kong, and similar gateways, offer various authentication mechanisms. For instance, you might want to secure your services using JWTs.
curl -i -X POST --url http://localhost:8001/services/example-service/plugins/ \
--data 'name=jwt' \
--data 'config.claims_to_verify=exp'
This ensures that requests to the example-service
must include a valid JWT, enhancing the security of your microservices.
Enabling Rate Limiting:
To protect your services from overload or abuse, rate limiting is essential. This can be easily configured:
curl -i -X POST --url http://localhost:8001/services/example-service/plugins/ \
--data 'name=rate-limiting' \
--data 'config.minute=100'
This limits clients to 100 requests per minute to the example-service
, safeguarding your microservice's resources.
Performance and Monitoring
Finally, performance optimization and monitoring are key aspects of managing microservices with Docker API Gateways. Tools like Prometheus for monitoring and Grafana for visualization can be integrated with Kong to provide real-time insights into the health and performance of your services.
Conclusion
Mastering Docker API Gateways in microservice development offers immense benefits, from simplified communication and enhanced security to performance optimization. While the initial setup might seem daunting, the flexibility and control it grants developers over their microservice architectures are unparalleled. By following the steps outlined in this guide and exploring advanced features, developers can effectively manage their services, ensuring scalability, security, and an optimal end-user experience.
Remember, the journey doesn’t stop here. The landscape of microservices and containerization technologies is ever-evolving. Staying updated on the latest trends and best practices, like those shared on platforms such as Docker's official blog, is crucial for any developer looking to excel in this space.
Happy coding, and may your microservice architectures be as seamless and efficient as possible!