Making the Switch: Timing Your Move to Microservices
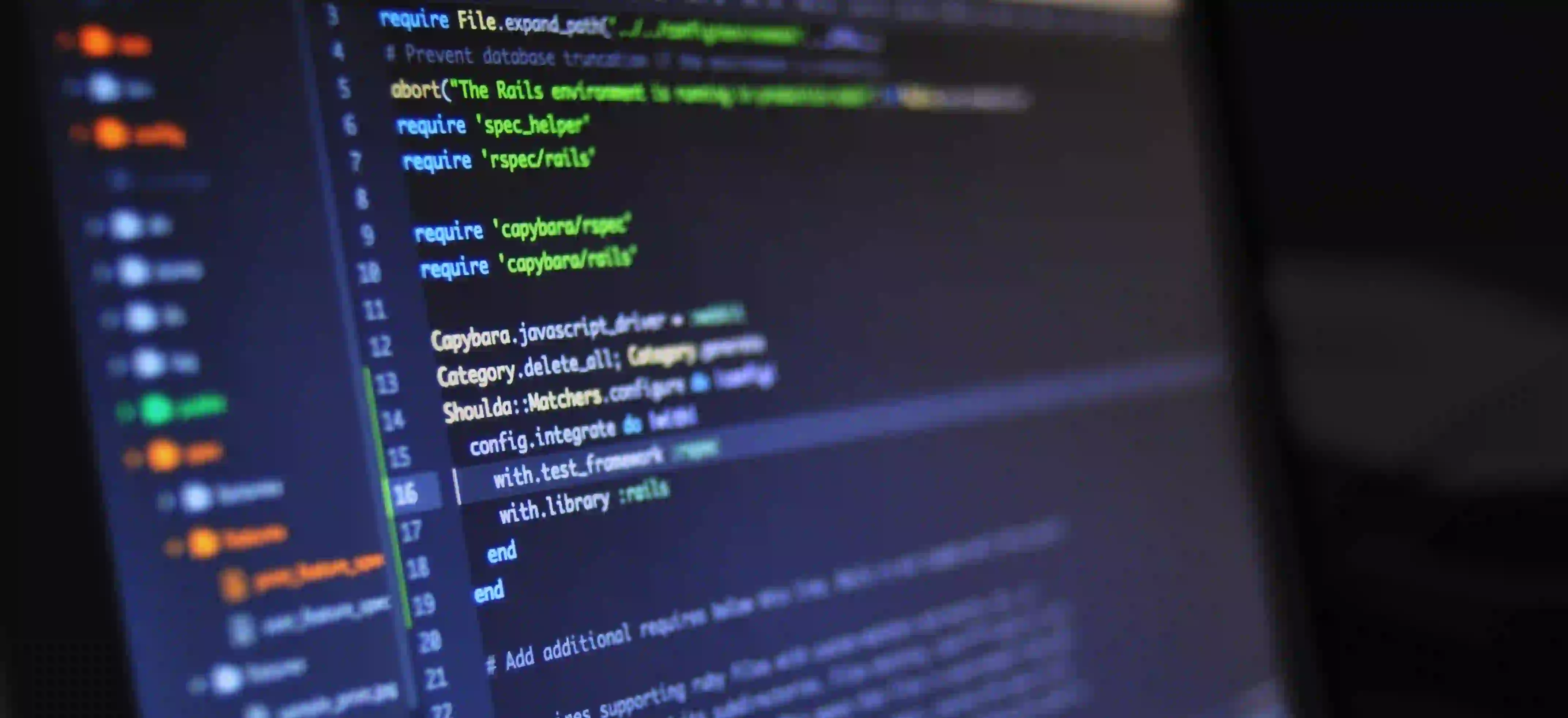
Making the Switch: Timing Your Move to Microservices
In recent years, there has been a paradigm shift in software architecture from monolithic to microservices. While monolithic architectures have their own advantages, such as simplicity and ease of development, microservices offer benefits like scalability, flexibility, and independent deployment. As a Java developer, understanding the best time to transition from a monolithic architecture to microservices is crucial. In this article, we'll delve into the considerations and strategies for timing your move to microservices in the context of Java development.
Understanding the Monolith
Before delving into the considerations for transitioning to microservices, let's first understand the characteristics of a monolithic architecture. In a monolithic application, all components are interconnected and interdependent, sharing the same codebase and often deployed as a single unit. While this architecture simplifies development and testing, it can become cumbersome and challenging to scale as the application grows. Additionally, a single codebase means that any changes or updates require redeployment of the entire application.
Signs It's Time to Transition
Several indicators suggest that it might be the right time to transition from a monolithic architecture to microservices. Some of these signs include:
-
Scalability Challenges: As the user base and load on the application increase, it becomes difficult to scale specific components without affecting the entire application.
-
Technology Diversity: When new technologies need to be integrated with the existing application, a monolithic architecture may not be conducive to seamless integration.
-
Team Autonomy: As the development team grows, it becomes challenging to work on different parts of the application independently without stepping on each other's toes.
-
Frequent Deployment Hurdles: If deploying even a small change to the application requires the entire codebase to be redeployed, it becomes a bottleneck for continuous deployment and delivery.
Embracing Microservices: Key Considerations
Moving from a monolithic architecture to microservices is a significant undertaking and requires careful consideration. Here are the key considerations when timing your move to microservices as a Java developer:
1. Domain Analysis
Identify the different domains within your application. A domain represents a distinct business functionality or area. Breaking down the monolith into microservices based on distinct domains can help in realizing the benefits of microservices architecture. For instance, a retail application might have separate domains for inventory management, order processing, and customer management.
2. Modularization and Bounded Contexts
In a monolithic architecture, modules are usually tightly coupled. However, in microservices, each service should encapsulate a bounded context, implying that the service should encapsulate all the data and business rules for a specific domain. As a Java developer, this means identifying clear boundaries between different domains and ensuring that each microservice is independently deployable.
3. Technology Decoupling
A monolithic architecture often dictates a specific technology stack for the entire application. Moving to microservices allows for technology diversity, enabling each microservice to use a technology stack that best fits its requirements. Java’s strength in microservices development lies in its ability to integrate with various technologies and its robust ecosystem.
4. Database Decoupling
In a monolithic architecture, a single database is often shared among different modules of the application. Transitioning to microservices requires careful consideration of the database strategy. One approach involves each microservice having its own database, while another approach utilizes a database per service and sharing data through API calls. The choice depends on factors such as data consistency requirements and the autonomy of each service.
5. Communication and Coordination
In a monolithic architecture, function calls are often local and in-process. With microservices, inter-service communication becomes a critical aspect. As a Java developer, adopting technologies such as RESTful APIs, messaging queues, or gRPC for communication between services is crucial. Understanding and implementing resilient communication patterns is essential for the success of microservices.
Transition Strategies for Java Developers
Transitioning from a monolithic architecture to microservices can be approached through various strategies. As a Java developer, here are three common strategies that can be employed:
1. Strangler Fig Pattern
The Strangler Fig Pattern, popularized by Martin Fowler, involves gradually replacing parts of the monolith with microservices. In Java, this can be achieved by identifying specific modules or functionalities within the monolith that can be extracted into microservices. These microservices are then gradually introduced, replacing their monolithic counterparts until the entire application is composed of microservices.
2. Branch by Abstraction
This approach involves creating abstractions that allow certain parts of the monolith to be gradually replaced by microservices. In Java, this can be realized through the use of interfaces and dependency injection. By gradually implementing these abstractions and introducing microservices behind them, the transition to microservices can be achieved without disrupting the overall functionality of the application.
3. Event-Driven Architecture
Event-driven architecture facilitates the decoupling of components within an application. Java’s support for asynchronous messaging through libraries like Apache Kafka and RabbitMQ can be leveraged to implement event-driven communication between different parts of the application. By employing event-driven patterns, Java developers can gradually transition the monolith into a set of interconnected microservices.
Code Example: Implementing RESTful Communication
@RestController
public class OrderController {
@Autowired
private OrderService orderService;
@PostMapping("/orders")
public ResponseEntity<String> createOrder(@RequestBody OrderRequest orderRequest) {
String orderId = orderService.createOrder(orderRequest);
return ResponseEntity.ok("Order created with ID: " + orderId);
}
@GetMapping("/orders/{orderId}")
public ResponseEntity<Order> getOrder(@PathVariable String orderId) {
Order order = orderService.getOrder(orderId);
return ResponseEntity.ok(order);
}
}
In this code snippet, we have a simplified OrderController
demonstrating RESTful communication in a microservices architecture. The controller exposes endpoints for creating and retrieving orders. By using Spring's @RestController
and @Autowired
annotations, we leverage Java's capabilities for building RESTful APIs.
A Final Look
Transitioning from a monolithic architecture to microservices is a significant decision that requires careful planning and consideration. As a Java developer, understanding the timing and strategies for making this transition is essential to leverage the benefits of microservices while mitigating potential challenges. By analyzing domains, embracing modularization, and choosing appropriate transition strategies, Java developers can effectively navigate the transition to microservices, unlocking scalability, flexibility, and autonomy in their applications.
In conclusion, the move to microservices is not just a technical shift, but a fundamental transformation in how software is designed, deployed, and operated. With Java's robust ecosystem and versatile capabilities, Java developers are well-equipped to embrace the microservices paradigm and drive innovation in modern software architecture.